
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
Registry.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef REGISTRY_H 00018 #define REGISTRY_H 00019 00020 #include "mbed.h" 00021 #include "rtos.h" 00022 #include "InternalEEPROM.h" 00023 00024 /** 00025 * Example of using the Registry class: 00026 * 00027 * @code 00028 * #include "mbed.h" 00029 * #include "DMBoard.h" 00030 * 00031 * int main(void) { 00032 * DMBoard* board = &DMBoard::instance(); 00033 * board->init(); 00034 * ... 00035 * board->registry() 00036 * } 00037 * @endcode 00038 */ 00039 class Registry { 00040 public: 00041 00042 enum RegistryError { 00043 Ok = 0, 00044 ReadOnlyError, 00045 MemoryError, 00046 EEPROMReadError, 00047 EEPROMWriteError, 00048 NoSuchKeyError, 00049 KeyLenError, 00050 ValueLenError, 00051 InvalidPositionError, 00052 RegistryFullError, 00053 }; 00054 00055 /** Get the only instance of the Registry 00056 * 00057 * @returns The Registry 00058 */ 00059 static Registry& instance() 00060 { 00061 static Registry singleton; 00062 return singleton; 00063 } 00064 00065 /** Loads all (if any) values from the internal EEPROM 00066 * 00067 * @returns 00068 * Ok on success 00069 * An error code on failure 00070 */ 00071 RegistryError load(); 00072 00073 /** Sets (or replaces if existing) the value for the key 00074 * 00075 * The key/value parameters are copied so it is ok to free them 00076 * after calling this function. 00077 * 00078 * @param key the key to create or update 00079 * @param val the value to store for the key 00080 * 00081 * @returns 00082 * Ok on success 00083 * An error code on failure 00084 */ 00085 RegistryError setValue(const char* key, const char* val); 00086 00087 /** Gets (if any) the the value for the key 00088 * 00089 * If Ok is returned then pVal will point to allocated memory 00090 * that must be deallocated with free() by the caller. 00091 * 00092 * @param key the key to look for 00093 * @param pVal will hold the value if successful 00094 * 00095 * @returns 00096 * Ok on success 00097 * NoSuchKeyError if there is no value for the key 00098 * An error code on failure 00099 */ 00100 RegistryError getValue(const char* key, char** pVal); 00101 00102 /** Retrieves the key-value pair at the specified index 00103 * 00104 * If Ok is returned then pKey and pVal will point to allocated 00105 * memory that must be deallocated with free() by the caller. 00106 * 00107 * @param pos the index to look for 00108 * @param pKey will hold the key if successful 00109 * @param pVal will hold the value if successful 00110 * 00111 * @returns 00112 * Ok on success 00113 * An error code on failure 00114 */ 00115 RegistryError entryAt(int pos, char** pKey, char** pVal); 00116 00117 /** Returns the number of key-value pairs in the registry 00118 * 00119 * @returns the number of key-value pairs 00120 */ 00121 int numEntries() { return _numEntries; } 00122 00123 RegistryError registerListener(); 00124 00125 /** Stores the registry in the internal EEPROM 00126 * 00127 * @returns 00128 * Ok on success 00129 * An error code on failure 00130 */ 00131 RegistryError store(); 00132 00133 00134 private: 00135 enum Constants { 00136 NumEntries = InternalEEPROM::EEPROM_NUM_PAGES, 00137 EntryLen = 32, 00138 EntrySize = 2*EntryLen, 00139 }; 00140 00141 typedef struct { 00142 char key[EntryLen]; 00143 char val[EntryLen]; 00144 } reg_entry_t; 00145 00146 uint8_t* _data; 00147 int _numEntries; 00148 reg_entry_t* _entries; 00149 bool _modified[NumEntries]; 00150 Mutex _mutex; 00151 00152 explicit Registry(); 00153 // hide copy constructor 00154 Registry(const Registry&); 00155 // hide assign operator 00156 Registry& operator=(const Registry&); 00157 ~Registry(); 00158 00159 RegistryError fromEEPROM(); 00160 RegistryError toEEPROM(); 00161 int find(const char* key); 00162 }; 00163 00164 #endif
Generated on Tue Jul 12 2022 14:18:31 by
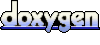