
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
MCIFileSystem.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MCIFILESYSTEM_H 00018 #define MCIFILESYSTEM_H 00019 00020 #include "mbed.h" 00021 #include "FATFileSystem.h" 00022 #include "GPDMA.h" 00023 00024 /** Access the filesystem on an SD Card using MCI 00025 * 00026 * @code 00027 * #include "mbed.h" 00028 * #include "MCIFileSystem.h" 00029 * 00030 * MCIFileSystem mcifs("mci"); 00031 * 00032 * int main() { 00033 * printf("Please insert a SD/MMC card\n"); 00034 * while(!mcifs.cardInserted()) { 00035 * wait(0.5); 00036 * } 00037 * 00038 * printf("Found SD/MMC card, writing to /mci/myfile.txt ...\n"); 00039 * 00040 * FILE *fp = fopen("/mci/myfile.txt", "w"); 00041 * if (fp != NULL) { 00042 * fprintf(fp, "Hello World!\n"); 00043 * fclose(fp); 00044 * printf("Wrote to /mci/myfile.txt\n"); 00045 * } else { 00046 * printf("Failed to open /mci/myfile.txt\n"); 00047 * } 00048 * } 00049 * @endcode 00050 */ 00051 class MCIFileSystem : public BlockDevice { 00052 public: 00053 00054 /** Create the File System for accessing an SD/MMC Card using MCI 00055 * 00056 * @param cd The pin connected to the CardDetect line 00057 */ 00058 MCIFileSystem(PinName cd = P4_16); 00059 00060 virtual ~MCIFileSystem(); 00061 00062 virtual int init(); 00063 virtual int deinit(); 00064 00065 virtual int sync(); 00066 virtual int read(void * buffer, bd_addr_t addr, bd_size_t size); 00067 virtual int program(const void * buffer, bd_addr_t addr, bd_size_t size); 00068 virtual bd_size_t get_read_size() const; 00069 virtual bd_size_t get_program_size() const; 00070 virtual bd_size_t size() const; 00071 virtual const char *get_type() const; 00072 00073 void mci_MCIIRQHandler(); 00074 void mci_DMAIRQHandler(); 00075 00076 /** Tests if a SD/MMC card is inserted or not. 00077 * 00078 * @returns 00079 * True if a card has been inserted, 00080 * False if no card is inserted or if the card detect pin is unavailable 00081 */ 00082 bool cardInserted() const; 00083 00084 private: 00085 00086 typedef enum { 00087 CardStatusNumBytes = 4, 00088 } Constants; 00089 00090 typedef enum { 00091 PowerOff = 0, 00092 PowerUp = 2, 00093 PowerOn = 3, 00094 } power_ctrl_t; 00095 00096 typedef enum { 00097 SDMMC_IDLE_ST = 0, /*!< Idle state */ 00098 SDMMC_READY_ST, /*!< Ready state */ 00099 SDMMC_IDENT_ST, /*!< Identification State */ 00100 SDMMC_STBY_ST, /*!< standby state */ 00101 SDMMC_TRAN_ST, /*!< transfer state */ 00102 SDMMC_DATA_ST, /*!< Sending-data State */ 00103 SDMMC_RCV_ST, /*!< Receive-data State */ 00104 SDMMC_PRG_ST, /*!< Programming State */ 00105 SDMMC_DIS_ST /*!< Disconnect State */ 00106 } CardState; 00107 00108 00109 typedef struct { 00110 uint8_t CmdIndex; 00111 uint32_t Data[CardStatusNumBytes]; 00112 } response_t; 00113 00114 /** 00115 * @brief SDC Clock Control Options 00116 */ 00117 typedef enum { 00118 SDC_CLOCK_ENABLE = 8, /*!< Enable SD Card Bus Clock */ 00119 SDC_CLOCK_POWER_SAVE = 9, /*!< Disable SD_CLK output when bus is idle */ 00120 SDC_CLOCK_DIVIDER_BYPASS = 10, /*!< Enable bypass of clock divide logic */ 00121 SDC_CLOCK_WIDEBUS_MODE = 11, /*!< Enable wide bus mode (SD_DAT[3:0] is used instead of SD_DAT[0]) */ 00122 } ClockControl; 00123 00124 /** 00125 * @brief SD/MMC Card specific setup data structure 00126 */ 00127 typedef struct { 00128 uint32_t response[4]; /*!< Most recent response */ 00129 uint32_t cid[4]; /*!< CID of acquired card */ 00130 uint32_t csd[4]; /*!< CSD of acquired card */ 00131 uint32_t ext_csd[512 / 4]; /*!< Ext CSD */ 00132 uint32_t card_type; /*!< Card Type */ 00133 uint16_t rca; /*!< Relative address assigned to card */ 00134 uint32_t speed; /*!< Speed */ 00135 uint32_t block_len; /*!< Card sector size */ 00136 bd_size_t device_size; /*!< Device Size */ 00137 uint32_t blocknr; /*!< Block Number */ 00138 uint32_t clk_rate; /*!< Clock rate */ 00139 } SDMMC_CARD_T; 00140 00141 typedef enum { 00142 SDC_RET_OK = 0, 00143 SDC_RET_CMD_FAILED = -5001, 00144 SDC_RET_BAD_PARAMETERS = -5002, 00145 SDC_RET_BUS_NOT_IDLE = -5003, 00146 SDC_RET_TIMEOUT = -5004, 00147 SDC_RET_ERR_STATE = -5005, 00148 SDC_RET_NOT_READY = -5006, 00149 SDC_RET_FAILED = -5007, 00150 } ReturnCode; 00151 00152 void initMCI(); 00153 00154 int32_t mci_Acquire(); 00155 uint32_t mci_GetCardStatus() const; 00156 CardState mci_GetCardState() const; 00157 ReturnCode mci_ReadBlocks(void *buffer, int32_t startBlock, int32_t blockNum); 00158 ReturnCode mci_WriteBlocks(void *buffer, int32_t startBlock, int32_t blockNum); 00159 void mci_SetClock(uint32_t freq) const; 00160 void mci_ClockControl(ClockControl ctrlType, bool enable) const; 00161 void mci_PowerControl(power_ctrl_t powerMode, uint32_t flag) const; 00162 ReturnCode mci_ExecuteCmd(uint32_t Command, uint32_t Arg, response_t* pResp) const; 00163 ReturnCode mci_SendIfCond() const; 00164 ReturnCode mci_SendOpCond(uint32_t *pOCR) const; 00165 ReturnCode mci_SendAppOpCond(uint16_t rca, bool hcs, uint32_t *pOcr, bool *pCCS) const; 00166 ReturnCode mci_GetCID(uint32_t *pCID) const; 00167 ReturnCode mci_SetAddr(uint16_t addr) const; 00168 ReturnCode mci_GetAddr(uint16_t *pRCA) const; 00169 ReturnCode mci_GetCSD(uint16_t rca, uint32_t *pCSD) const; 00170 ReturnCode mci_SelectCard(uint16_t addr) const; 00171 ReturnCode mci_GetStatus(uint16_t rca, uint32_t *pStatus) const; 00172 void mci_ProcessCSD(); 00173 ReturnCode mci_SetBusWidth(uint16_t rca, uint8_t width) const; 00174 ReturnCode mci_SetTranState(uint16_t rca) const; 00175 ReturnCode mci_SetBlockLength(uint32_t rca, uint32_t block_len) const; 00176 ReturnCode mci_SetCardParams() const; 00177 ReturnCode mci_StopTransmission(uint32_t rca) const; 00178 bool mci_CheckR1Response(uint32_t resp, ReturnCode* pCheckResult) const; 00179 void mci_WriteDelay() const; 00180 ReturnCode mci_SendCmd(uint32_t Command, uint32_t Arg, uint32_t timeout) const; 00181 ReturnCode mci_SendAppCmd(uint16_t rca) const; 00182 void mci_SetDataTransfer(uint16_t BlockNum, bool DirFromCard, uint32_t Timeout) const; 00183 void mci_GetResp(response_t* pResp) const; 00184 uint32_t mci_GetBits(int32_t start, int32_t end, uint32_t *data) const; 00185 void mci_SetCommand(uint32_t Cmd, uint32_t Arg) const; 00186 void mci_ResetCommand() const; 00187 int32_t mci_IRQHandler(uint8_t *txBuf, uint32_t *txCnt, uint8_t *rxBuf, uint32_t *rxCnt); 00188 int32_t mci_FIFOIRQHandler(uint8_t *txBuf, uint32_t *txCnt, uint8_t *rxBuf, uint32_t *rxCnt); 00189 void mci_ReadFIFO(uint32_t *pDst, bool bFirstHalf) const; 00190 void mci_WriteFIFO(uint32_t *pSrc, bool bFirstHalf) const; 00191 00192 void mci_SetupEventWakeup(); 00193 uint32_t mci_WaitForEvent() const; 00194 00195 ReturnCode _readBlocks(uint32_t card_type, uint32_t startBlock, uint32_t blockNum) const; 00196 ReturnCode _writeBlocks(uint32_t card_type, uint32_t startBlock, uint32_t blockNum) const; 00197 00198 SDMMC_CARD_T _sdCardInfo; 00199 00200 DigitalIn* _cardDetect; 00201 00202 GPDMA::DMAChannels _eventDmaChannel; /*!< DMA Channel used for transfer data */ 00203 volatile bool _eventReceived; 00204 volatile bool _eventSuccess; 00205 00206 uint32_t _init_ref_count; 00207 bool _is_initialized; 00208 }; 00209 00210 #endif
Generated on Tue Jul 12 2022 14:18:31 by
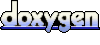