
A board support package for the LPC4088 Display Module.
Dependencies: DM_HttpServer DM_USBHost
Dependents: lpc4088_displaymodule_emwin lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI ... more
Fork of DMSupport by
QSPIFileSystem.h
00001 /* 00002 * Copyright 2014 Embedded Artists AB 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef QSPIFILESYSTEM_H 00018 #define QSPIFILESYSTEM_H 00019 00020 #include "mbed.h" 00021 #include "FileSystemLike.h" 00022 00023 /** Access the filesystem on an QSPI flash using SPIFI 00024 * 00025 * One way to utilize the 8MByte of QSPI FLASH on the LPC4088 QuickStart Board 00026 * is to place a file system on it. The QSPIFileSystem is using the 00027 * FileSystemLike interface making using it is as simple as: 00028 * 00029 * @code 00030 * #include "mbed.h" 00031 * #include "QSPIFileSystem.h" 00032 * 00033 * QSPIFileSystem qspifs("qspi"); 00034 * 00035 * int main() { 00036 * if (!qspifs.isformatted()) { 00037 * qspifs.format(); 00038 * } 00039 * 00040 * FILE *fp = fopen("/qspi/myfile.txt", "w"); 00041 * if (fp != NULL) { 00042 * fwrite("Hello World!", 12, 1, fp); 00043 * fclose(fp); 00044 * } 00045 * } 00046 * @endcode 00047 * 00048 * The file system can be formatted to a specific size in increments of 1MByte 00049 * and will allways be placed at the top of the address range. For the 8 MByte 00050 * memory on the LPC4088 QuickStart Board this means: 00051 * 00052 * <pre> 00053 * 0x28000000 0x28800000 00054 * |------|------|------|------|------|------|------|------| 00055 * qspifs.format(1) | available for program | FS | 00056 * |------|------|------|------|------|------|------|------| 00057 * </pre><pre> 00058 * |------|------|------|------|------|------|------|------| 00059 * qspifs.format(2) | available for program | FileSystem | 00060 * |------|------|------|------|------|------|------|------| 00061 * </pre><pre> 00062 * |------|------|------|------|------|------|------|------| 00063 * qspifs.format(7) | PROG | FileSystem | 00064 * |------|------|------|------|------|------|------|------| 00065 * </pre><pre> 00066 * |------|------|------|------|------|------|------|------| 00067 * qspifs.format(8) | FileSystem | 00068 * |------|------|------|------|------|------|------|------| 00069 * </pre> 00070 * The file system must be placed at the top of the memory because the linker scripts 00071 * places your program at the bottom of the memory (if needed). 00072 * 00073 * The file system is using one or more blocks (each the size of one erase block) per 00074 * file. Each file is stored in a sequence of blocks. If a file is written to so that 00075 * it's size will occupy more than one block and the block after is already used by 00076 * a different file the entire file will be moved, like this: 00077 * 00078 * <pre> 00079 * |-------|-------|-------|-------|-------|-------|-------| 00080 * Before |///////| FILE1 | FILE2 | FILE3 |///////|///////|///////| 00081 * |-------|-------|-------|-------|-------|-------|-------| 00082 * </pre><pre> 00083 * After writing |-------|-------|-------|-------|-------|-------|-------| 00084 * to FILE2 |///////| FILE1 |///////| FILE3 | FILE2 |///////| 00085 * |-------|-------|-------|-------|-------|-------|-------| 00086 * </pre> 00087 * 00088 * <b>Note: </b>As each file takes up at least one block it will limit the total number of files that 00089 * can be stored on the file system. The formula for this is roughly 00090 * <pre> 00091 * max_num_files = fs_size_in_bytes / erase_block_size 00092 * </pre> 00093 * For the SPI flash on the LPC4088 QuickStart Board it means 00094 * <pre> 00095 * max_num_files = 8MByte / 4KByte = 2048 00096 * </pre> 00097 * 00098 * Some of the blocks are used to store the table of content (TOC) at the very end of 00099 * the spi flash. The TOC will contain a list of all blocks on the flash (even those 00100 * not used by the file system) and will mark each block as "reserved","in use" or "free". 00101 * For a file system that is 4MByte on a 8MByte flash half of the blocks will be marked 00102 * as "reserved". 00103 * 00104 * <b>Note: </b>The file system will not store any date/time information. 00105 * 00106 * <b>Note: </b>The file system will not store any file attributes (hidden/system/read only). 00107 * 00108 * <b>Note: </b>The file system stores the absolute path of each file (path + file name) in 00109 * the file's first block's first 256 bytes. Folders are never stored themselves. This has 00110 * the drawback that the file system cannot hold empty folders. 00111 */ 00112 class QSPIFileSystem : public FileSystemLike { 00113 public: 00114 00115 /** Create the File System for accessing a QSPI Flash 00116 * 00117 * @param name The name used to access the virtual filesystem 00118 */ 00119 QSPIFileSystem(const char* name); 00120 00121 virtual int open(FileHandle **file, const char *filename, int flags); 00122 virtual int open(DirHandle **dir, const char *path); 00123 00124 virtual int remove(const char *filename); 00125 virtual int rename(const char *oldname, const char *newname); 00126 00127 virtual int mkdir(const char *name, mode_t mode); 00128 00129 /** Creates a new file system on the QSPI flash. 00130 * The file system will have the specified size and will always 00131 * be positioned at the end of the QSPI flash. If the fsSizeInMB is 00132 * less than the size of the QSPI flash the lower end of the flash 00133 * will be left untouched. 00134 * 00135 * @param fsSizeInMB The size of the file system 00136 * 00137 * @returns 00138 * 0 on success, 00139 * -1 on failure. 00140 */ 00141 int format(unsigned int fsSizeInMB = 8); 00142 00143 /** Tests if there is a file system present on the QSPI flash 00144 * 00145 * @returns 00146 * True if a valid file system could be found, 00147 * False on failure. 00148 */ 00149 bool isformatted(); 00150 00151 /** Retrieves the start and end addresses for the file system. 00152 * The pStartAddr and pEndAddr will only be assigned values if the 00153 * function returns true. 00154 * 00155 * @param pStartAddr Will return the start of the file system area 00156 * @param pEndAddr Will return the end of the file system area 00157 * 00158 * @returns 00159 * True if there is a file system, 00160 * False on failure. 00161 */ 00162 bool getMemoryBoundaries(uint32_t* pStartAddr, uint32_t* pEndAddr); 00163 }; 00164 00165 #endif
Generated on Tue Jul 12 2022 14:18:31 by
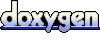