Initial Commit
Embed:
(wiki syntax)
Show/hide line numbers
robot.h
00001 #ifndef ROBOT_H 00002 #define ROBOT_H 00003 #include "QEI.h" 00004 #include "mbed.h" 00005 #include "rtos.h" 00006 #include "HC05.h" 00007 #include "MPU6050.h" 00008 #include "I2Cdev.h" 00009 00010 #include "motors.h" 00011 //#include "MPU6050.h" 00012 //#include "HC05.h" 00013 //#include "ACS712.h" 00014 //#include "MPU6050_6Axis_MotionApps20.h" 00015 //#include "DMP.h" 00016 //#include "IMUDATA.h" 00017 //#include "ADNS5090.h" 00018 #define BaudRate_bt 115200 //Baud rate of 9600 00019 #define tx_bt PTA2 //Bluetooth connection pins 00020 #define rx_bt PTA1 //Bluetooth connection pins 00021 #define EN_BT PTA4 //Bluetooth connection pins 00022 //#define tx_mpu PTE0 //MPU connection pins 00023 //#define rx_mpu PTE1 //MPU connection pins 00024 //#define mpu_bandwidth MPU6050_BW_20 //set the MPU low pass filter bandwidth to 20hz 00025 00026 #define myledd PTB8 //status LED pin 00027 #define CURRENTSENSOR_PIN PTB3 00028 #define VOLTAGESENSOR_PIN PTB0 00029 00030 #define CURRENT_R1 180 //160.0 //values of the current sensor opamp resistors 00031 #define CURRENT_R2 10 00032 #define CURRENT_R3 80 00033 #define CURRENT_R4 84.7 00034 #define VREF3_3 3.3 //digital logic voltage 00035 #define VREF5 5.0 //5v voltage //NOTE: 5v voltage is consistent when using new batts, but not using old blue batts 00036 00037 00038 //#define irL PTB1 00039 //#define irC PTB3 00040 //#define irR PTB2 00041 00042 #define LDR1 PTB1 00043 #define LDR2 PTB2 00044 #define buzz PTC8 00045 00046 #define MOT_PWMA_PIN PTA5 //Motor control pins 00047 #define MOT_PWMB_PIN PTA12 00048 #define MOT_STBY_PIN PTE31 00049 #define MOT_AIN1_PIN PTE30 00050 #define MOT_AIN2_PIN PTE29 00051 #define MOT_BIN1_PIN PTE24 00052 #define MOT_BIN2_PIN PTE25 00053 00054 #define M_PI 3.14159265359 //PI 00055 #define gyroCorrect 3720 //divide raw gyro data by this to get result in RAD/SECOND (if gyroRange is 500 rad/s) 00056 00057 //Correct direction of motors. If number = 1, forward. If number = 0, backwards (for if motors are wired backwards) 00058 #define MOTOR_R_DIRECTION 1 00059 #define MOTOR_L_DIRECTION 1 00060 #define L_MOTOR_SCALE 1 00061 #define R_MOTOR_SCALE 1 00062 #define MOTOR_INTERVAL 20 //defines the interval (in milliseconds) between when motor can be set 00063 //NOTE: Can't make this less than 20ms, because that is the PWM frequency 00064 00065 //Key bindings for remote control robot - for the future try to use arrow keys instead of 'asdw' 00066 #define ctrl_forward 'i' //forward 00067 #define ctrl_backward 'k' //back 00068 #define ctrl_left 'j' //turn left 00069 #define ctrl_right 'l' //turn right 00070 #define ctrl_calibrate 'c' //re-calibrate the accelerometer and gyro 00071 #define ctrl_turn_angle_cw 'o' // turn angle 00072 #define ctrl_turn_angle_ccw 'p' 00073 // The nRF24L01+ supports transfers from 1 to 32 bytes, but Sparkfun's 00074 // "Nordic Serial Interface Board" (http://www.sparkfun.com/products/9019) 00075 // only handles 4 byte transfers in the ATMega code. 00076 #define TRANSFER_SIZE 4 00077 #define ulrL PTD6 00078 #define ulL PTD5 00079 #define ulF PTD1 00080 #define ulR PTC2 00081 #define ulrR PTC1 00082 #define ulB PTC0 00083 00084 00085 extern Motors motors; 00086 extern HC05 bt; //bluetooth connection 00087 //extern Serial bt; 00088 //extern QEI wheel; 00089 extern QEI left; 00090 extern QEI right; 00091 //extern MPU6050 mpu; //MPU connection 00092 extern DigitalOut myled; //(PTE3) Processor LED (1 = off, 0 = on) 00093 //extern DigitalOut btSwitch; 00094 //extern AnalogIn currentSensor; 00095 extern DigitalOut buzzer; 00096 extern DigitalOut key;\ 00097 //extern PwmOut buzzer; 00098 extern AnalogIn LDRsensor1; 00099 extern AnalogIn LDRsensor2; 00100 //extern AnalogIn voltageSensor; 00101 /////////// Motor control variables /////////// 00102 extern PwmOut PWMA;//(MOT_PWMA_PIN); 00103 extern PwmOut PWMB;//(MOT_PWMB_PIN); 00104 extern DigitalOut AIN1;//(MOT_AIN1_PIN); 00105 extern DigitalOut AIN2;//(MOT_AIN2_PIN); 00106 extern DigitalOut BIN1;//(MOT_BIN1_PIN); 00107 extern DigitalOut BIN2;//(MOT_BIN2_PIN); 00108 extern DigitalOut STBY;//(MOT_STBY_PIN); 00109 00110 extern DigitalOut SRX; 00111 00112 extern AnalogIn uL; 00113 extern AnalogIn uF; 00114 extern AnalogIn uR; 00115 extern AnalogIn urR; 00116 extern AnalogIn urL; 00117 extern AnalogIn uB; 00118 extern int dy; 00119 extern int dx; 00120 extern int Rmotor_speed; 00121 extern int Lmotor_speed; 00122 int r_time (); 00123 void motor_control(int Lspeed, int Rspeed); //Input speed for motors. Integer between 0 and 100 00124 void stop(); //stop motors 00125 void set_direction(double angle); //set angle for the robot to face (from origin) 00126 void set_direction_deg(double angle); 00127 void set_speed(int Speed ); //set speed for robot to travel at 00128 void auto_enable(bool x); 00129 /** 00130 * MPU CONTROLS 00131 */ 00132 // calibrate the gyro and accelerometer // 00133 void calibrate(); 00134 /** 00135 * Status: find the distance, orientation, battery values, etc of the robot 00136 */ 00137 //void distanceTravelled(double x[3]) 00138 //void orientation(something quaternion? on xy plane?) 00139 double getCurrent(); //Get the current drawn by the robot 00140 double getCurrent(int n); //get the current, averaged over n samples 00141 double getVoltage(); //get the battery voltage (ask connor for completed function) 00142 double ldrread1(); 00143 double ldrread2(); 00144 void Led_on(); 00145 void Led_off(); 00146 void initRobot(); 00147 extern double pl_buzzer(); 00148 extern void pl_buzzer(int freq, int f_time); 00149 extern void Imu_yaw(void const *args); 00150 extern void encoder_thread(void const *args); 00151 extern int freq; 00152 extern int rMotor; 00153 extern int lMotor ; 00154 extern int m_speed; 00155 extern Mutex stdio_mutex; 00156 extern MPU6050 accelgyro; 00157 extern int16_t ax, ay, az; 00158 extern int16_t gx, gy, gz; 00159 extern int heading; 00160 extern int software_interuupt; 00161 extern int Encoder_x; 00162 extern int Encoder_y; 00163 extern char Selected_robot; 00164 extern bool bt_connected; 00165 //Wireless connections 00166 00167 #endif
Generated on Mon Jul 18 2022 14:52:03 by
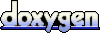