
Code for collecting sensor data
Dependencies: SX1276Lib_inAir mbed
Fork of Sensors by
main.cpp
00001 #include <stdio.h> 00002 #include "mbed.h" 00003 #include "sx1276-inAir.h" 00004 00005 #define STRING_LENGTH 30 00006 #define SUPPLY_VOLTAGE 3.3 00007 00008 Serial pc(USBTX, USBRX); //Create a serial connection to the PC 00009 AnalogIn ain(PA_0); //Configure pin PA0 as an analog input for the temperature sensor 00010 //DigitalIn din(PB_10); //Configure pin PC2 as a digital input for the reed switch 00011 InterruptIn door(PB_10); 00012 00013 float reading_float; 00014 bool reading_bool; 00015 00016 //Return the temperature from the sensor, in degrees celsius 00017 float getTemp() { 00018 float reading = ain.read(); 00019 float output_voltage = reading * SUPPLY_VOLTAGE; 00020 return (output_voltage - 0.25) / 0.028; 00021 } 00022 00023 //Return the door state. If True, the door is closed. If False, the door is open. 00024 bool getDoorState() { 00025 return door.read(); 00026 } 00027 00028 //Code that executes on a rising edge 00029 //For reasons unknown, this executes twice instead of once when the magnet connects 00030 //i.e. there are two falling edges. Could implement debouncing, but that takes effort 00031 void transmitDoorClosing() { 00032 printf("The door has been closed\r\n"); 00033 } 00034 00035 //Code that executes on a falling edge 00036 void transmitDoorOpening() { 00037 printf("The door has been opened\r\n"); 00038 } 00039 00040 00041 int main() { 00042 00043 //Configure the serial connection (baud rate = 19200, 8 data bits, 1 stop bit) 00044 pc.baud(9600); 00045 pc.format(8, SerialBase::None, 1); 00046 00047 door.rise(transmitDoorClosing); //Rising edge occurs when the "door opens" 00048 door.fall(transmitDoorOpening); //Falling edge occurs when the "door closes" 00049 00050 while(1) { 00051 00052 char reading_string[STRING_LENGTH]; 00053 reading_float = getTemp(); 00054 sprintf(reading_string, " Temp: %.8f\r\n", reading_float); 00055 pc.printf("Temperature = %s\r\n", reading_string); 00056 00057 wait_ms(500); 00058 00059 } 00060 }
Generated on Fri Jul 22 2022 14:45:02 by
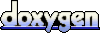