
Ikenna Adrian Ozoemena 201157039
Dependencies: mbed
RosenEngine.cpp
00001 #include "RosenEngine.h" 00002 00003 DigitalIn A(PTB9); 00004 00005 // Constructor 00006 RosenEngine::RosenEngine() 00007 { 00008 00009 } 00010 // Destructor 00011 RosenEngine::~RosenEngine() 00012 { 00013 00014 } 00015 00016 00017 void RosenEngine::init(int ship_width,int ship_height,int ship_speed,int ship_xpos,int ship_ypos) 00018 { 00019 // initialise the game parameters 00020 _ship.init(ship_width,ship_height,ship_speed,ship_xpos,ship_ypos); 00021 _no_shooters = 0; 00022 _no_seekers = 0; 00023 _enemy.init(_no_shooters,_no_seekers); 00024 _menu.init(16); 00025 _health.init(_shipUsed); 00026 _times_run = 0; 00027 _score = 0; 00028 _dead = false; 00029 _intro = false; 00030 _wait_time = 2.25; 00031 00032 } 00033 void RosenEngine::reset() 00034 { 00035 // reinitialize parameters once back is pressed and game is exited 00036 _enemy.init(_no_shooters,_no_seekers); 00037 _health.init(_shipUsed); 00038 _wait_time = 2.25; 00039 _times_run = 0; 00040 _score = 0; 00041 _no_shooters = 0; 00042 _dead = false; 00043 _no_seekers = 0; 00044 } 00045 00046 void RosenEngine::read_input(Gamepad &pad) 00047 { 00048 // read all inputs from the gamepad 00049 Vector2D mapped_coord = pad.get_coord(); 00050 _joystick.x = mapped_coord.x; 00051 _joystick.y = mapped_coord.y; 00052 _d = pad.get_direction(); 00053 wait(0.1); 00054 // printf("_joystick.x ,_joystick.y = %f , %f\n",_joystick.x, _joystick.y); 00055 } 00056 00057 void RosenEngine::draw(N5110 &lcd, Gamepad &pad) 00058 { 00059 // Draw border 00060 lcd.drawRect(0,0,78,48,FILL_TRANSPARENT); 00061 // Draw all other game assets 00062 _health.draw_health(lcd,_shipUsed); 00063 _health.draw_shields(lcd); 00064 _enemy.draw_seeker(lcd); 00065 _enemy.draw_shooter(lcd); 00066 _enemy.draw_shw(lcd,pad); 00067 draw_ship(lcd,pad); 00068 disp_points(lcd); 00069 // if player dies display death scene 00070 if(_dead == true) { 00071 game_over(lcd); 00072 } 00073 } 00074 void RosenEngine::draw_ship(N5110 &lcd, Gamepad &pad) 00075 { 00076 // find the closest enemy (used in orions firing) 00077 Vector2D inde = find_closest1(); 00078 int index = inde.x; 00079 Vector2D closest = find_closest2(index); 00080 // Draw ships and set ship parameters depending on the ship being used 00081 switch (_shipUsed) { 00082 case kestrel: 00083 _ship.set_parameters(9,6,4); 00084 _ship.draw_ship(lcd,_shipUsed); 00085 _weapons.draw(lcd,pad,_shipUsed,closest); 00086 break; 00087 case imperion: 00088 _ship.set_parameters(7,10,2); 00089 _ship.draw_ship(lcd,_shipUsed); 00090 _weapons.draw(lcd,pad,_shipUsed,closest); 00091 break; 00092 case orion: 00093 _ship.set_parameters(7,10,3); 00094 _ship.draw_ship(lcd,_shipUsed); 00095 _weapons.draw(lcd,pad,_shipUsed,closest); 00096 break; 00097 } 00098 //printf("_shipUsed = %d\n",_shipUsed); 00099 } 00100 00101 void RosenEngine::update(Gamepad &pad) 00102 { 00103 // set the ship in use (kestrel imperion or orion) 00104 set_shipUsed(); 00105 // update enemies 00106 _enemy.update_seeker(_shipPos.x, _shipPos.y); 00107 _enemy.update_shooter(_shipPos.x, _shipPos.y); 00108 _enemy.update_shw(); 00109 // update ship weapons 00110 update_ship_weapon(pad); 00111 // check collisions 00112 shooter_ship_collision(pad); 00113 seeker_ship_collision(pad); 00114 shooterw_ship_collision(pad); 00115 imperionw_seeker_collision(pad); 00116 kestrelw_shooter_collision(pad); 00117 imperionw_shooter_collision(pad); 00118 orionw_collision(pad); 00119 // check health 00120 check_health(); 00121 // increase difficulty over time 00122 scaling(timer(12)); 00123 00124 } 00125 void RosenEngine::update_ship_weapon(Gamepad &pad) 00126 { 00127 // update ship weapons based on ship being used 00128 if(_shipUsed == kestrel) { 00129 _ship.update_ship(_joystick.x,_joystick.y); 00130 _weapons.update(); 00131 kestrelw_seeker_collision(pad); 00132 } 00133 if(_shipUsed == imperion && A == false) { 00134 _ship.update_ship(_joystick.x,_joystick.y); 00135 _weapons.update(); 00136 } 00137 if(_shipUsed == orion) { 00138 _ship.update_ship(_joystick.x,_joystick.y); 00139 _weapons.update(); 00140 } 00141 } 00142 void RosenEngine::get_pos() 00143 { 00144 // get ship position 00145 _shipPos = _ship.get_pos(); 00146 // get enemy position 00147 _seekerPos[0] = _enemy.get_seekerpos(1); 00148 _seekerPos[1] = _enemy.get_seekerpos(2); 00149 _seekerPos[2] = _enemy.get_seekerpos(3); 00150 _shooterPos[0] = _enemy.get_shooterpos(1); 00151 _shooterPos[1] = _enemy.get_shooterpos(2); 00152 _shooterPos[2] = _enemy.get_shooterpos(3); 00153 _shooterWPos[0] = _enemy.get_shwpos(1); 00154 _shooterWPos[1] = _enemy.get_shwpos(2); 00155 _shooterWPos[2] = _enemy.get_shwpos(3); 00156 // set ship size 00157 set_ship_size(); 00158 // weapons are initialized here to pass accurate size values to the weapon class 00159 _weapons.init(_shipPos.x, _shipPos.y, _shipWidth); 00160 _ycursor = _menu.get_ycursor(); 00161 } 00162 void RosenEngine::set_ship_size() 00163 { 00164 // change ship size depending on ship being used 00165 switch (_shipUsed) { 00166 case kestrel: 00167 _shipWidth = 9; 00168 _shipHeight = 6; 00169 break; 00170 case imperion: 00171 _shipWidth = 7; 00172 _shipHeight = 10; 00173 break; 00174 case orion: 00175 _shipWidth = 7; 00176 _shipHeight = 10; 00177 break; 00178 } 00179 } 00180 void RosenEngine::title(N5110 &lcd) 00181 { 00182 // display main menu screen 00183 _menu.title(lcd,_shipUsed); 00184 _menu.update(_d,_joystick); 00185 } 00186 int RosenEngine::get_ycursor() 00187 { 00188 _ycursor = _menu.get_ycursor(); 00189 return _ycursor; 00190 } 00191 void RosenEngine::set_shipUsed() 00192 { 00193 // assign the ship being used based on xcursor which is the cursor in 00194 // the ship select part of the menu 00195 int shipno = _menu.get_xcursor(); 00196 _shipUsed = (SHIP)shipno; 00197 } 00198 void RosenEngine::ship_select(N5110 &lcd) 00199 { 00200 _menu.update(_d,_joystick); 00201 _menu.disp_ships(lcd); 00202 } 00203 void RosenEngine::help(N5110 &lcd) 00204 { 00205 _lore.help(lcd); 00206 } 00207 bool RosenEngine::check_collision(int xpos1, int ypos1,int width1,int height1,int xpos2, int ypos2,int width2,int height2) 00208 { 00209 // Create arrays of all positions with appropriate height and length 00210 int xpos1_array[width1]; 00211 int ypos1_array[height1]; 00212 int xpos2_array[width2]; 00213 int ypos2_array[height2]; 00214 bool xcol = false; 00215 bool ycol = false; 00216 bool col = false; 00217 //printf("variables declared\n"); 00218 // Loop through both height and width to get a 2D aray of the sprites position 00219 // and compare all positionr returning true is any match 00220 for(int cx = 0; cx<width1; cx=cx+1) { 00221 xpos1_array[cx]= xpos1 + cx; 00222 for(int nx = 0; nx<width2; nx=nx+1) { 00223 xpos2_array[nx]= xpos2 + nx; 00224 if(xpos2_array[nx] == xpos1_array[cx]) { 00225 xcol = true; 00226 } 00227 } 00228 } 00229 //printf("first loop done\n"); 00230 for(int cy = 0; cy<height1; cy=cy+1) { 00231 ypos1_array[cy]= ypos1 + cy; 00232 for(int ny = 0; ny<height2; ny=ny+1) { 00233 ypos2_array[ny]= ypos2 + ny; 00234 if(ypos2_array[ny] == ypos1_array[cy]) { 00235 ycol = true; 00236 } 00237 } 00238 } 00239 // if both the hight and width position values are equal a collision has occured 00240 col = (xcol & ycol); 00241 return col; 00242 } 00243 bool RosenEngine::check_collision1(int xpos1,int width1,int xpos2,int width2) 00244 { 00245 // Create arrays of all positions with appropriate width values 00246 int xpos1_array[width1]; 00247 int xpos2_array[width2]; 00248 bool xcol = false; 00249 // loop through both arrays comparing their values and return true if any are equal 00250 for(int cx = 0; cx<width1; cx=cx+1) { 00251 xpos1_array[cx]= xpos1 + cx; 00252 for(int nx = 0; nx<width2; nx=nx+1) { 00253 xpos2_array[nx]= xpos2 + nx; 00254 if(xpos2_array[nx] == xpos1_array[cx]) { 00255 xcol = true; 00256 } 00257 } 00258 } 00259 return xcol; 00260 } 00261 00262 void RosenEngine::seeker_ship_collision(Gamepad &pad) 00263 { 00264 bool col1,col2,col3; 00265 // Check for any collisions using the check_collision function 00266 col1 = check_collision(_shipPos.x,_shipPos.y,9,6,_seekerPos[0].x, _seekerPos[0].y,10,7); 00267 col2 = check_collision(_shipPos.x,_shipPos.y,9,6,_seekerPos[1].x, _seekerPos[1].y,10,7); 00268 col3 = check_collision(_shipPos.x,_shipPos.y,9,6,_seekerPos[2].x, _seekerPos[2].y,10,7); 00269 int sel = 0; 00270 if (col1 == true && _no_seekers >= 1) { 00271 sel = 1; 00272 } 00273 if (col2 == true && _no_seekers >= 2) { 00274 sel = 2; 00275 } 00276 if (col3 == true && _no_seekers >= 3) { 00277 sel = 3; 00278 } 00279 // printf("col1 = %d, col2 = %d, col3 = %d, no_seekers = %d\n",col1,col2,col3,_no_seekers); 00280 // if there is a collision between any seeker and the ship update both the seeker and the ships health 00281 if(sel != 0) { 00282 _health.update(5,pad); 00283 _health.seekerh_update(sel,10); 00284 pad.tone(500,0.05); 00285 wait(0.05); 00286 } 00287 } 00288 void RosenEngine::shooter_ship_collision(Gamepad &pad) 00289 { 00290 bool col1,col2,col3; 00291 int sel = 0; 00292 // Check for any collisions using the check_collision function 00293 col1 = check_collision(_shipPos.x,_shipPos.y,9,6,_shooterPos[0].x, _shooterPos[1].y,10,7); 00294 col2 = check_collision(_shipPos.x,_shipPos.y,9,6,_shooterPos[1].x, _shooterPos[0].y,10,7); 00295 col3 = check_collision(_shipPos.x,_shipPos.y,9,6,_shooterPos[2].x, _shooterPos[0].y,10,7); 00296 00297 if(col1 == true && _no_shooters >= 1) { 00298 sel = 1; 00299 } 00300 if(col2 == true && _no_shooters >= 2) { 00301 sel = 2; 00302 } 00303 if(col3 == true && _no_shooters >= 3) { 00304 sel = 3; 00305 } 00306 // if there is a collision between any shooter and the ship update both the shooter and the ships health 00307 if(sel != 0) {// sel is default 0 and will only change if a collision occurs 00308 _health.update(1,pad); 00309 _health.shooterh_update(sel,10); 00310 pad.tone(500,0.05); 00311 wait(0.05); 00312 } 00313 } 00314 void RosenEngine::shooterw_ship_collision(Gamepad &pad) 00315 { 00316 bool col1,col2,col3; 00317 int sel = 0; 00318 // Check for any collisions using the check_collision function 00319 col1 = check_collision(_shipPos.x,_shipPos.y,9,6,_shooterWPos[0].x, _shooterWPos[1].y,2,2); 00320 col2 = check_collision(_shipPos.x,_shipPos.y,9,6,_shooterWPos[1].x, _shooterWPos[2].y,2,2); 00321 col3 = check_collision(_shipPos.x,_shipPos.y,9,6,_shooterWPos[2].x, _shooterWPos[2].y,2,2); 00322 00323 if(col1 == true && _no_shooters >= 1) { 00324 sel = 1; 00325 } 00326 if(col2 == true && _no_shooters >= 2) { 00327 sel = 2; 00328 } 00329 if(col3 == true && _no_shooters >= 3) { 00330 sel = 3; 00331 } 00332 // if there is a collision and the ship was hit update the ships damage 00333 if(sel != 0) {// sel is default 0 and will only change if a collision occurs 00334 _health.update(1,pad); 00335 pad.tone(500,0.05); 00336 wait(0.05); 00337 } 00338 } 00339 void RosenEngine::kestrelw_seeker_collision(Gamepad &pad) 00340 { // the kestrel fires a projectile whose x and y values must be checked with the 00341 // x, and y, values of every enemy to check for a collision 00342 bool col1, col2, col3; 00343 int sel = 0; 00344 Vector2D missle_pos = _weapons.get_pos(_shipUsed); // get the kestrel's projectile position 00345 // Check for any collisions using the check_collision function 00346 col1 = check_collision(_seekerPos[0].x,_seekerPos[0].y,9,6,missle_pos.x,missle_pos.y,1,1); 00347 col2 = check_collision(_seekerPos[1].x,_seekerPos[1].y,9,6,missle_pos.x,missle_pos.y,1,1); 00348 col3 = check_collision(_seekerPos[2].x,_seekerPos[2].y,9,6,missle_pos.x,missle_pos.y,1,1); 00349 if (col1 == true && _no_seekers >= 1) { 00350 sel = 1; 00351 } 00352 if (col2 == true && _no_seekers >= 2) { 00353 sel = 2; 00354 } 00355 if (col3 == true && _no_seekers >= 3) { 00356 sel = 3; 00357 } 00358 // if there are collisions update the seekers health 00359 if(sel != 0) {// sel is default 0 and will only change if a collision occurs 00360 pad.tone(500,0.05); 00361 _health.seekerh_update(sel,5); 00362 wait(0.05); 00363 } 00364 } 00365 void RosenEngine::imperionw_seeker_collision(Gamepad &pad) 00366 { // the imperion fires a lazer to the top of screen as such we don't need to check all the 00367 // x, y values for the sprite to be sure of collisions just the x value. as long as the ship is above 00368 // the targetted enemy a collision will register 00369 bool col1,col2,col3; 00370 int sel = 0; 00371 if(_shipUsed == imperion) { 00372 if(_shipPos.y > _seekerPos[0].y + 6) { // only regiser if seeker is above ship 00373 col1 = check_collision1(_seekerPos[0].x,9,_shipPos.x + 2,3); // check for collision using the check_collision1 function 00374 if (col1 == true && A == true && _no_seekers >= 1) { 00375 sel = 1; // if there is a collision set an appropriate select value 00376 } 00377 } 00378 if(_shipPos.y > _seekerPos[1].y + 6) { 00379 col2 = check_collision1(_seekerPos[1].x,9,_shipPos.x + 2,3); 00380 if (col2 == true && A == true && _no_seekers >= 2) { 00381 sel = 2; 00382 } 00383 } 00384 if(_shipPos.y > _seekerPos[0].y + 6) { 00385 col3 = check_collision1(_seekerPos[2].x,9,_shipPos.x + 2,3); 00386 if (col3 == true && A == true && _no_seekers >= 3) { 00387 sel = 3; 00388 } 00389 } 00390 } 00391 // if a collision occured update the seekers health 00392 if(sel != 0) {// sel is default 0 and will only change if a collision occurs 00393 _health.seekerh_update(sel,10); 00394 pad.tone(500,0.05); 00395 wait(0.05); 00396 } 00397 } 00398 void RosenEngine::kestrelw_shooter_collision(Gamepad &pad) 00399 { 00400 Vector2D missle_pos = _weapons.get_pos(_shipUsed); // get the kestrel's projectile position 00401 bool col1, col2, col3; 00402 int sel = 0; 00403 // Check for any collisions using the check_collision function 00404 col1 = check_collision(_shooterPos[0].x,_shooterPos[0].y,9,6,missle_pos.x,missle_pos.y,1,1); 00405 col2 = check_collision(_shooterPos[1].x,_shooterPos[1].y,9,6,missle_pos.x,missle_pos.y,1,1); 00406 col3 = check_collision(_shooterPos[2].x,_shooterPos[2].y,9,6,missle_pos.x,missle_pos.y,1,1); 00407 if (col1 == true && _no_shooters >= 1) { 00408 sel = 1; 00409 } 00410 if (col2 == true && _no_shooters >= 2) { 00411 sel = 2; 00412 } 00413 if (col3 == true && _no_shooters >= 3) { 00414 sel = 3; 00415 } 00416 // if a collision did occur update the right shooter using the select value 00417 if(sel != 0) { // sel is default 0 and will only change if a collision occurs 00418 pad.tone(500,0.05); 00419 _health.shooterh_update(sel,5); 00420 wait(0.05); 00421 } 00422 } 00423 void RosenEngine::imperionw_shooter_collision(Gamepad &pad) 00424 { 00425 bool col1,col2,col3; 00426 int sel = 0; 00427 // check collision using check_collision1 function 00428 if(_shipUsed == imperion) { 00429 if(_shipPos.y > _shooterPos[0].y + 6) { 00430 col1 = check_collision1(_shooterPos[0].x,9,_shipPos.x + 2,3); 00431 if (col1 == true && A == true && _no_shooters >= 1) { 00432 sel = 1; 00433 } 00434 } 00435 if(_shipPos.y > _shooterPos[1].y + 6) { 00436 col2 = check_collision1(_shooterPos[1].x,9,_shipPos.x + 2,3); 00437 if (col2 == true && A == true && _no_shooters >= 2) { 00438 sel = 2; 00439 } 00440 } 00441 if(_shipPos.y > _shooterPos[2].y + 6) { 00442 col3 = check_collision1(_shooterPos[2].x,9,_shipPos.x + 2,3); 00443 if (col3 == true && A == true && _no_shooters >= 3) { 00444 sel = 3; 00445 } 00446 } 00447 } 00448 // if a collision did occur update the shooters health 00449 if(sel != 0) {// sel is default 0 and will only change if a collision occurs 00450 _health.shooterh_update(sel,10); 00451 pad.tone(500,0.05); 00452 wait(0.05); 00453 } 00454 } 00455 void RosenEngine::orionw_collision(Gamepad &pad) 00456 { // the orions weapon is an arc weapon that latches on to the nearest enemy. 00457 // as the nearest enemy position has already been found in dind_closest() 00458 // all we need to do now is ensure that the enemy is within range of the orions weapon 00459 Vector2D inde = find_closest1(); 00460 int index1 = inde.x; 00461 int index2 = inde.x - 3; 00462 int distance = inde.y; 00463 00464 // enemy 1,2 and 3 are shooters 00465 if(_no_shooters >= index1 && A == true && distance < 15) {// the wepon fires when a is pressed and the enemy ship is within the distaance 00466 _health.shooterh_update(index1,10); 00467 pad.tone(500,0.05); 00468 wait(0.05); 00469 } 00470 // enemy 4,5 and 6 are seekers so 3 is subtracted from inde.x to compensate 00471 if(_no_seekers >= index2 && A == true && distance < 15) { 00472 _health.seekerh_update(index2,10); 00473 pad.tone(500,0.05); 00474 wait(0.05); 00475 } 00476 00477 00478 } 00479 void RosenEngine::check_health() 00480 { 00481 // check player and enemy health 00482 check_se_health(); 00483 check_sh_health(); 00484 Vector2D hp = _health.get_hp(); 00485 if(hp.x <= 0) { 00486 // printf("player deaad\n"); 00487 _dead = true; 00488 } 00489 00490 } 00491 void RosenEngine::check_se_health() 00492 { 00493 // set sel (select) as default 0 00494 int sel = 0; 00495 // get the seekers health 00496 int seeker1_health = _health.get_seekerh(1); 00497 int seeker2_health = _health.get_seekerh(2); 00498 int seeker3_health = _health.get_seekerh(3); 00499 // printf("seeker1_h = %d, seeker2_h = %d, seeker3_h = %d\n",seeker1_health,seeker2_health,seeker3_health); 00500 // set sel (select) depending on if any seekers health falls below zero 00501 if(seeker1_health == 0) { 00502 sel = 1; 00503 } 00504 if(seeker2_health == 0) { 00505 sel = 2; 00506 } 00507 if(seeker3_health == 0) { 00508 sel = 3; 00509 } 00510 // reset seeker and update score when seekers health goes to zero 00511 if(sel != 0) { 00512 _enemy.reset_seeker(sel); 00513 _health.reset_seekerh(sel); 00514 _score = _score + 10; 00515 } 00516 } 00517 void RosenEngine::check_sh_health() 00518 { // set sel (select) as default 0 00519 int sel = 0; 00520 // get the shooters health 00521 int shooter1_health = _health.get_shooterh(1); 00522 int shooter2_health = _health.get_shooterh(2); 00523 int shooter3_health = _health.get_shooterh(3); 00524 // set sel depending on if any shooters health falls below zero 00525 if(shooter1_health == 0) { 00526 sel = 1; 00527 } 00528 if(shooter2_health == 0) { 00529 sel = 2; 00530 } 00531 if(shooter3_health == 0) { 00532 sel = 3; 00533 } 00534 // reset shooter and update score when shooter health goes to zero 00535 if(sel != 0) { 00536 _enemy.reset_shooter(sel); 00537 _health.reset_shooterh(sel); 00538 _score = _score + 20; 00539 } 00540 } 00541 int RosenEngine::rand_no() 00542 { 00543 // seeds and returns a random number using the ctime library 00544 srand(time(NULL)); 00545 int rand_no = (rand() %45) + 1; 00546 return rand_no; 00547 } 00548 float RosenEngine::timer(int fps) 00549 { 00550 // using the amount of times run and the frames per second set the amount of 00551 // time past is calculated 00552 _times_run = _times_run + 1; 00553 float time_frame = 1.0f/fps; 00554 float time_elapsed = _times_run * time_frame; 00555 // printf("time elapsed = %f,time frame = %f, _times_run = %d\n",time_elapsed,time_frame,_times_run); 00556 return time_elapsed; 00557 } 00558 void RosenEngine::score(int points) 00559 { 00560 _score = _score + points; 00561 } 00562 bool RosenEngine::dead() 00563 { 00564 return _dead; 00565 } 00566 00567 void RosenEngine::scaling(float time_elapsed) 00568 { 00569 // increases difficulty as game progresses 00570 if(time_elapsed == _wait_time) { // check if time elapsed is equal to the wit time 00571 _no_shooters = _no_shooters + 1; // update number of shooters and seekers after wait time 00572 _no_seekers = _no_seekers + 1; //increase wait time 00573 _enemy.sh_scaling(time_elapsed); 00574 _wait_time = _wait_time + 10.00; 00575 } 00576 // limit the number of seekers and number of shooters to 3 00577 if(_no_shooters > 3) { 00578 _no_shooters = 3; 00579 } 00580 if(_no_seekers > 3) { 00581 _no_seekers = 3; 00582 } 00583 // set approriate enemy numbers 00584 _enemy.set_noshooters(_no_shooters); 00585 _enemy.set_noseekers(_no_seekers); 00586 // printf("time_elapsed = %f, no_shooters = %d, wait_time = %f\n",time_elapsed,_no_shooters,_wait_time); 00587 } 00588 void RosenEngine::game_over(N5110 &lcd) 00589 { 00590 // Display random tips after every loss 00591 _lore.display(lcd,rand_no()); 00592 } 00593 void RosenEngine::intro(N5110 &lcd) 00594 { 00595 // display intro 00596 _lore.intro(lcd); 00597 _intro = true; 00598 } 00599 void RosenEngine::disp_points(N5110 &lcd) 00600 { 00601 // display players points on screen 00602 char buffer[10]; 00603 sprintf(buffer,"%d",_score); 00604 lcd.printString(buffer,2,0); 00605 } 00606 Vector2D RosenEngine::get_enemynum() 00607 { 00608 // return the number of enemy shooters and seekers currently on play 00609 return{_no_shooters,_no_seekers}; 00610 } 00611 int RosenEngine::range(int x1, int y1, float x2, float y2) 00612 { 00613 // calculates average distance between two points 00614 float rangex = (abs(x1 - x2)); 00615 float rangey = (abs(y1 - y2)); 00616 int distance = floor((rangex+rangey)/2); 00617 return distance; 00618 } 00619 Vector2D RosenEngine::find_closest1() 00620 { 00621 // get the distance for all enemies 00622 int sh1 = range(_shipPos.x,_shipPos.y,_shooterPos[0].x,_shooterPos[0].y); 00623 int sh2 = range(_shipPos.x,_shipPos.y,_shooterPos[1].x,_shooterPos[1].y); 00624 int sh3 = range(_shipPos.x,_shipPos.y,_shooterPos[2].x,_shooterPos[2].y); 00625 int se1 = range(_shipPos.x,_shipPos.y,_seekerPos[0].x,_seekerPos[0].y); 00626 int se2 = range(_shipPos.x,_shipPos.y,_seekerPos[1].x,_seekerPos[1].y); 00627 int se3 = range(_shipPos.x,_shipPos.y,_seekerPos[2].x,_seekerPos[2].y); 00628 00629 int close[6] = {sh1,sh2,sh3,se1,se2,se3}; 00630 // find index of the smallest element 00631 int index = 0; 00632 int smallest = close[0]; 00633 for(int i=0; i<6; i=i+1) { 00634 if(smallest>close[i]) { 00635 smallest=close[i]; 00636 index = i; 00637 } 00638 } 00639 // return the index, so we know what the closest enemy, and the distance to check its in range 00640 return {(index + 1),smallest}; 00641 } 00642 Vector2D RosenEngine::find_closest2(int index) 00643 { 00644 // return the position of the closest enemy 00645 if(index == 1 && _no_shooters >= 1) { 00646 return {_shooterPos[0].x,_shooterPos[0].y}; 00647 } 00648 if(index == 2 && _no_shooters >= 2) { 00649 return {_shooterPos[1].x,_shooterPos[1].y}; 00650 } 00651 if(index == 3 && _no_shooters >= 3) { 00652 return {_shooterPos[2].x,_shooterPos[2].y}; 00653 } 00654 if(index == 4 && _no_seekers >= 1 ) { 00655 return {_seekerPos[0].x,_seekerPos[0].y}; 00656 } 00657 if(index == 5 && _no_seekers >= 2) { 00658 return {_seekerPos[1].x,_seekerPos[1].y}; 00659 } 00660 if(index == 6 && _no_seekers >= 3) { 00661 return {_seekerPos[2].x,_seekerPos[2].y}; 00662 } 00663 00664 } 00665 Vector2D RosenEngine::get_shipPos() 00666 { 00667 return{_shipPos}; 00668 }
Generated on Wed Jul 13 2022 17:45:42 by
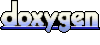