
Ikenna Adrian Ozoemena 201157039
Dependencies: mbed
Lore.cpp
00001 #include "Lore.h" 00002 // ship sprites 00003 const int seekerSprite[7][9] = { 00004 {1,1,0,0,0,0,0,1,1}, 00005 {1,0,1,0,0,0,1,0,1}, 00006 {1,0,0,1,1,1,0,0,1}, 00007 {0,1,0,0,0,0,0,1,0}, 00008 {0,0,1,0,0,0,1,0,0}, 00009 {0,0,1,1,0,1,1,0,0}, 00010 {0,0,0,0,1,0,0,0,0}, 00011 }; 00012 const int shooterSprite[10][11] = { 00013 {0,0,1,0,0,0,0,0,1,0,0}, 00014 {0,1,0,1,0,0,0,1,0,1,0}, 00015 {1,0,0,1,0,1,0,1,0,0,1}, 00016 {1,0,0,1,1,0,1,1,0,0,1}, 00017 {0,1,0,0,0,0,0,0,0,1,0}, 00018 {0,1,0,0,1,1,1,0,0,1,0}, 00019 {0,0,1,0,0,1,0,0,1,0,0}, 00020 {0,0,0,1,0,0,0,1,0,0,0}, 00021 {0,0,0,0,1,0,1,0,0,0,0}, 00022 {0,0,0,0,0,1,0,0,0,0,0}, 00023 }; 00024 const int orionSprite[10][7] = { 00025 {0,0,0,1,0,0,0}, 00026 {0,0,1,0,1,0,0}, 00027 {0,1,0,0,0,1,0}, 00028 {1,0,0,1,0,0,1}, 00029 {0,1,0,0,0,1,0}, 00030 {0,0,1,0,1,0,0}, 00031 {1,0,0,1,0,0,1}, 00032 {1,0,1,0,1,0,1}, 00033 {1,1,0,0,0,1,1}, 00034 {1,0,0,0,0,0,1}, 00035 00036 }; 00037 const int seekerSpriteLarge[14][17] = { 00038 {1,1,1,1,0,0,0,0,0,0,0,0,0,1,1,1,1}, 00039 {1,0,0,0,1,0,0,0,0,0,0,0,1,0,0,0,1}, 00040 {1,0,0,0,0,1,0,0,0,0,0,1,0,0,0,0,1}, 00041 {1,0,0,0,0,0,1,1,1,1,1,0,0,0,0,0,1}, 00042 {1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1}, 00043 {0,1,0,0,0,0,0,0,1,0,0,0,0,0,0,1,0}, 00044 {0,0,1,0,0,0,0,1,0,1,0,0,0,0,1,0,0}, 00045 {0,0,0,1,0,0,0,1,0,1,0,0,0,1,0,0,0}, 00046 {0,0,0,1,0,0,0,1,0,1,0,0,0,1,0,0,0}, 00047 {0,0,0,1,0,0,0,1,0,1,0,0,0,1,0,0,0}, 00048 {0,0,0,1,1,1,0,0,1,0,0,1,1,1,0,0,0}, 00049 {0,0,0,0,0,0,1,0,0,0,1,0,0,0,0,0,0}, 00050 {0,0,0,0,0,0,0,1,0,1,0,0,0,0,0,0,0}, 00051 {0,0,0,0,0,0,0,0,1,0,0,0,0,0,0,0,0}, 00052 00053 00054 }; 00055 00056 // Constructor 00057 Lore::Lore() 00058 { 00059 00060 } 00061 // Destructor 00062 Lore::~Lore() 00063 { 00064 00065 } 00066 void Lore::help(N5110 &lcd) 00067 { 00068 // display help 00069 lcd.clear(); 00070 lcd.refresh(); 00071 ships1(lcd); 00072 lcd.refresh(); 00073 } 00074 void Lore::display(N5110 &lcd,int rand) 00075 { 00076 // set the output to be a random number between 0 and 6 00077 int random = rand%6; 00078 lcd.clear(); 00079 lcd.refresh(); 00080 // Display a random tip 00081 switch (random) { 00082 case 0: 00083 shooter(lcd); 00084 break; 00085 case 1: 00086 seeker(lcd); 00087 break; 00088 case 2: 00089 kestrel(lcd); 00090 break; 00091 case 3: 00092 orion(lcd); 00093 break; 00094 case 4: 00095 imperion(lcd); 00096 break; 00097 case 5: 00098 menu1(lcd); 00099 break; 00100 } 00101 lcd.refresh(); 00102 wait(3); 00103 00104 } 00105 00106 void Lore::seeker(N5110 &lcd) 00107 { 00108 lcd.drawSprite(33,5,14,17,(int *)seekerSpriteLarge); 00109 lcd.printString(" Seekers ",2,3); 00110 lcd.printString(" explode ",2,4); 00111 lcd.printString(" on impact ",2,5); 00112 } 00113 void Lore::shooter(N5110 &lcd) 00114 { 00115 lcd.drawSprite(16,2,10,11,(int *)shooterSprite); 00116 lcd.drawSprite(38,5,10,11,(int *)shooterSprite); 00117 lcd.drawSprite(59,2,10,11,(int *)shooterSprite); 00118 lcd.printString("Shooters will",2,2); 00119 lcd.printString(" allign next ",2,3); 00120 lcd.printString("to you before",2,4); 00121 lcd.printString(" they fire",2,5); 00122 } 00123 void Lore::kestrel(N5110 &lcd) 00124 { 00125 lcd.printString("the kestrel's",2,0); 00126 lcd.printString("fire rate ",2,1); 00127 lcd.printString("increases the",2,2); 00128 lcd.printString(" closer you",2,3); 00129 lcd.printString("are to danger",2,4); 00130 } 00131 void Lore::imperion(N5110 &lcd) 00132 { 00133 lcd.printString("the imperion ",2,0); 00134 lcd.printString(" excels in ",2,1); 00135 lcd.printString("damage output ",2,2); 00136 lcd.printString(" but is ",2,3); 00137 lcd.printString(" immobile ",2,4); 00138 lcd.printString("during firing ",2,5); 00139 } 00140 void Lore::orion(N5110 &lcd) 00141 { 00142 lcd.drawSprite(70,34,10,7,(int *)orionSprite); 00143 lcd.drawLine(70 + 2,34 + 2,45,30,FILL_BLACK); 00144 lcd.drawLine(70 + 2,34 + 2,55,48,FILL_BLACK); 00145 lcd.printString("the orion is",2,0); 00146 lcd.printString("the only ship",2,1); 00147 lcd.printString("capable of ",2,2); 00148 lcd.printString("harming",2,3); 00149 lcd.printString("escaping ",2,4); 00150 lcd.printString("enemies ",2,5); 00151 } 00152 void Lore::menu1(N5110 &lcd) 00153 { 00154 lcd.printString(" you can ",2,0); 00155 lcd.printString(" switch ships ",2,1); 00156 lcd.printString(" in the menu ",2,2); 00157 lcd.printString("some are better",2,3); 00158 lcd.printString(" for certain ",2,4); 00159 lcd.printString(" tasks. ",2,5); 00160 } 00161 00162 void Lore::ships1(N5110 &lcd) 00163 { 00164 lcd.printString("You have a",2,0); 00165 lcd.printString("choice of ",2,1); 00166 lcd.printString("three ships,",2,2); 00167 lcd.printString(" press a to ",2,3); 00168 lcd.printString("fire weapons ",2,4); 00169 } 00170 00171 void Lore::intro(N5110 &lcd) 00172 { 00173 lcd.clear(); 00174 lcd.refresh(); 00175 lcd.printString("You're tasked",2,0); 00176 lcd.printString(" with holding",2,1); 00177 lcd.printString(" the line ",2,2); 00178 lcd.printString(" from an ",2,3); 00179 lcd.printString("invading army ",2,4); 00180 lcd.refresh(); 00181 wait(2); 00182 lcd.clear(); 00183 lcd.refresh(); 00184 lcd.printString(" as an ",2,0); 00185 lcd.printString(" expendable ",2,1); 00186 lcd.printString(" asset, ",2,2); 00187 lcd.printString(" you are not ",2,3); 00188 lcd.printString(" expected to",2,4); 00189 lcd.printString(" survive ",2,5); 00190 lcd.refresh(); 00191 wait(1); 00192 }
Generated on Wed Jul 13 2022 17:45:42 by
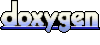