
Mobile Security System - Revision 1.0
Dependencies: FXOS8700Q N5110 SDFileSystem SRF02 mbed
main.h
00001 /** 00002 @file main.h 00003 @brief Header file containing functions prototypes, defines and global variables. 00004 @brief Revision 1.0. 00005 @author Daniel Gibbons 00006 @date May 2016 00007 */ 00008 00009 #ifndef MAIN_H 00010 #define MAIN_H 00011 00012 #define PI 3.14159265359 00013 00014 #include "mbed.h" 00015 #include "N5110.h" 00016 #include "SRF02.h" 00017 #include "FXOS8700Q.h" 00018 #include "SDFileSystem.h" 00019 00020 00021 /** 00022 @namespace lcd 00023 @brief N5110 LCD connections 00024 @namespace srf02 00025 @brief SRF02 sensor connections 00026 @namespace i2c 00027 @brief K64F I2C connections for FXOS8700Q on-board Accelerometer 00028 @namespace acc 00029 @brief K64F FXOS8700Q on-board Accelerometer connections 00030 @namespace sd 00031 @brief SD card connections 00032 @namespace pc 00033 @brief UART connection to PC for debugging 00034 @namespace led_alarm 00035 @brief LED to indicate status of the alarm: flashing -> setting or triggered ; constant -> set 00036 @namespace buzzer 00037 @brief Indicates status of the alarm: buzzes when alarm is setting and when triggered 00038 @namespace button_0 00039 @brief First button interrupt 00040 @namespace button_1 00041 @brief Second button interrupt 00042 @namespace button_c 00043 @brief Third button interrupt 00044 @namespace sw2 00045 @brief K64F on-board switch 00046 @namespace sw3 00047 @brief K64F on-board switch 00048 @namespace setting_distance 00049 @brief This ticker fires to read the distance when the system is setting 00050 @namespace intruder_distance 00051 @brief This ticker fires to read the distance when the system is set 00052 @namespace alerts 00053 @brief This ticker fires to turn and off the led and buzzer 00054 @namespace pin_timeout 00055 @brief This ticker fires to timeout the user if they don't enter the pin within an alotted time of triggering the device 00056 @namespace setting_screen 00057 @brief This ticker fires to display the setting animation on the setting screen 00058 @namespace accelerometer 00059 @brief This ticker fires whilst in the setting screen to get accelerometer data 00060 @namespace transition 00061 @brief This timeout transitions the system to the set state, five seconds after entering the setting state, or to the menu if the device is moved in the setting state 00062 @namespace tamper_transition 00063 @brief This timeout transitions the system to the menu if the device is moved in the setting state 00064 @namespace buzz 00065 @brief This timeout turns off the buzzer one second after the device is set 00066 @namespace confirm 00067 @brief This timeout transitions to a screen asking the user to confirm the pin that they have just entered 00068 @namespace r_led 00069 @brief K64F on-board red LED 00070 @namespace g_led 00071 @brief K64F on-board green LED 00072 @namespace b_led 00073 @brief K64F on-board blue LED 00074 00075 */ 00076 00077 // VCC, SCE, RST, D/C, MOSI, SCLK, LED 00078 N5110 lcd(PTE26,PTA0,PTC4,PTD0,PTD2,PTD1,PTC3); 00079 SRF02 srf02(I2C_SDA,I2C_SCL); 00080 I2C i2c(PTE25, PTE24); 00081 FXOS8700QAccelerometer acc(i2c, FXOS8700CQ_SLAVE_ADDR1); 00082 // MOSI, MISO, SCK, CS 00083 SDFileSystem sd(PTE3, PTE1, PTE2, PTE4, "sd"); 00084 Serial pc(USBTX,USBRX); 00085 PwmOut led_alarm(PTC10); 00086 PwmOut buzzer(PTC11); 00087 InterruptIn button_0(PTB23); 00088 InterruptIn button_1(PTA2); 00089 InterruptIn button_c(PTC2); 00090 InterruptIn sw2(SW2); 00091 InterruptIn sw3(SW3); 00092 Ticker setting_distance; 00093 Ticker intruder_distance; 00094 Ticker alerts; 00095 Ticker pin_timeout; 00096 Ticker setting_screen; 00097 Ticker accelerometer; 00098 Timeout transition; 00099 Timeout tamper_transition; 00100 Timeout buzz; 00101 Timeout confirm; 00102 DigitalOut r_led(LED_RED); 00103 DigitalOut g_led(LED_GREEN); 00104 DigitalOut b_led(LED_BLUE); 00105 00106 struct State { 00107 00108 /* array of next states which depend on which button was pressed 00109 - nextState[0] ----> button_0 pressed 00110 - nextState[1] ----> button_1 pressed 00111 - nextState[2] ----> button_c pressed (Option 1) 00112 - nextState[3] ----> button_c pressed (Option 2) 00113 */ 00114 00115 int nextState[4]; 00116 00117 }; 00118 typedef const struct State STyp; 00119 00120 // fsm stores all nine states that 'Mobile Security System' is comprised off 00121 STyp fsm[9] = { 00122 {0,0,0,0}, // 0 - initialisation (title screen) 00123 {2,3,1,1}, // 1 - main menu (Set alarm or set new password) 00124 {2,2,4,1}, // 2 - set alarm (enter password) 00125 {3,3,1,1}, // 3 - set new password (enter new password) 00126 {4,4,4,4}, // 4 - setting calibration 00127 {5,6,5,5}, // 5 - alarm activated 00128 {6,6,1,5}, // 6 - deactivate without triggering (enter password) 00129 {7,7,1,8}, // 7 - alarm triggered (enter password) 00130 {8,1,8,8} // 8 - alarm triggered 00131 }; 00132 00133 FILE *pin ; /*!< File pointer */ 00134 00135 int entered_pin [4]; /*!< Pin user enters */ 00136 int set_pin [4]; /*!< Pin that is saved to SD card and this is the current pin for the system */ 00137 int index_array [4]; /*!< Array to index the reading from the SD card */ 00138 00139 int pin_counter ; /*!< Increments to store the entered pin */ 00140 int incorrect_pin_flag ; /*!< If the entered pin doesn't match the set pin this flag is incremented to 1 */ 00141 00142 float acc_X ; /*!< Current x-axis reading from the accelerometer */ 00143 float acc_Y ; /*!< Current y-axis reading from the accelerometer */ 00144 float acc_Z ; /*!< Current z-axis reading from the accelerometer */ 00145 float setting_acc_X ; /*!< X-axis reading from the accelerometer when setting screen is initialised */ 00146 float setting_acc_Y ; /*!< Y-axis reading from the accelerometer when setting screen is initialised */ 00147 float setting_acc_Z ; /*!< Z-axis reading from the accelerometer when setting screen is initialised */ 00148 00149 int g_current_state ; /*!< The current state of the system */ 00150 int g_next_state ; /*!< The next state of the system */ 00151 00152 float distance [10]; /*!< Stores 10 distance readings from SRF02 */ 00153 float one_second_distance ; /*!< The total of all 10 distance readings taken in a second */ 00154 double one_second_avg_distance ; /*!< one_second_distance divided by 10 */ 00155 double initial_setting_distance ; /*!< The one_second_avg_distance calculated when in the setting state (state 4) */ 00156 00157 int setting_distance_counter ; /*!< increments to 10 distance readings can be stored over 1 second */ 00158 int intruder_distance_counter ; /*!< increments to 10 distance readings can be stored over 1 second */ 00159 int pin_timeout_counter ; /*!< increments to 20 to enable the 20 second timeout when the alarm is triggered */ 00160 int setting_alarm_counter ; /*!< increments in steps of five and is used for the setting screen animation */ 00161 00162 int seconds_till_timeout ; /*!< 21 - pin_timeout_counter */ 00163 00164 volatile int g_setting_distance_flag ; /*!< Flag in setting_distance_isr */ 00165 volatile int g_intruder_distance_flag ; /*!< Flag in intruder_distance_isr */ 00166 volatile int g_button_0_flag ; /*!< Flag in button_0_isr */ 00167 volatile int g_button_1_flag ; /*!< Flag in button_1_isr */ 00168 volatile int g_button_c_flag ; /*!< Flag in button_c_isr */ 00169 volatile int g_led_buzzer_flag ; /*!< Flag in led_buzzer_isr */ 00170 volatile int g_acc_flag ; /*!< Flag in acc_isr */ 00171 volatile int g_pin_timeout_flag ; /*!< Flag in pin_timeout_isr */ 00172 volatile int g_setting_screen_flag ; /*!< Flag in setting_screen_isr */ 00173 00174 char buffer [14]; /*!< Stores strings that are comprised of variables that are going to be displayed on the LCD */ 00175 int length ; /*!< Stores the length of strings that are comprised of variables that are going to be displayed on the LCD */ 00176 00177 /// 00178 /// Initialisation Functions 00179 /// 00180 void error(); /*!< Hangs flashing on_board LED */ 00181 00182 void init_serial(); /*!< Set-up the serial port */ 00183 00184 void init_K64F(); /*!< Set-up the on-board LEDs and switches */ 00185 00186 void init_buttons(); /*!< Set-up the three external buttons */ 00187 00188 void init_variables(); /*!< Initialises all variables to zero */ 00189 /// 00190 /// Interrupt Service Routines (ISRs) 00191 /// 00192 void setting_distance_isr(); /*!< Interrupt Service Routine that triggers when setting_distance ticker fires */ 00193 00194 void intruder_distance_isr(); /*!< Interrupt Service Routine that triggers when intruder_distance ticker fires */ 00195 00196 void button_0_isr(); /*!< Interrupt Service Routine that triggers when button_0 is pressed by the user */ 00197 00198 void button_1_isr(); /*!< Interrupt Service Routine that triggers when button_1 is pressed by the user */ 00199 00200 void button_c_isr(); /*!< Interrupt Service Routine that triggers when button_c is pressed by the user */ 00201 00202 void led_buzzer_isr(); /*!< Interrupt Service Routine that triggers when alerts ticker fires */ 00203 00204 void acc_isr(); /*!< Interrupt Service Routine that triggers when accelerometer ticker fires */ 00205 00206 void pin_timeout_isr(); /*!< Interrupt Service Routine that triggers when pin_timeout ticker fires */ 00207 00208 void setting_screen_isr(); /*!< Interrupt Service Routine that triggers when setting_screen ticker fires */ 00209 /// 00210 /// Button Functions 00211 /// 00212 void button_0_protocol(); /*!< Protocol when button_0 is pressed */ 00213 00214 void button_1_protocol(); /*!< Protocol when button_1 is pressed */ 00215 00216 void button_c_protocol(); /*!< Protocol when button_c is pressed */ 00217 /// 00218 /// State Functions 00219 /// 00220 void state_0_screen(); /*!< Sets the screen and the necessary tickers and timeouts for state 0 */ 00221 00222 void state_1_screen(); /*!< Sets the screen and the necessary tickers and timeouts for state 1 */ 00223 00224 void state_2_screen(); /*!< Sets the screen and the necessary tickers and timeouts for state 2 */ 00225 00226 void state_3_screen(); /*!< Sets the screen and the necessary tickers and timeouts for state 3 */ 00227 00228 void state_4_screen(); /*!< Sets the screen and the necessary tickers and timeouts for state 4 */ 00229 00230 void state_5_screen(); /*!< Sets the screen and the necessary tickers and timeouts for state 5 */ 00231 00232 void state_6_screen(); /*!< Sets the screen and the necessary tickers and timeouts for state 6 */ 00233 00234 void state_7_screen(); /*!< Sets the screen and the necessary tickers and timeouts for state 7 */ 00235 00236 void state_8_screen(); /*!< Sets the screen and the necessary tickers and timeouts for state 8 */ 00237 00238 void lcd_border(); /*!< Prints a border to the Nokia 5110 LCD */ 00239 00240 void pin_text_box(); /*!< Prints a box to the Nokia 5110 LCD for the states where a pin is required */ 00241 00242 void screen_progression(); /*!< Calls check pin to see if the entered pin is correct and from there either proceed or go back */ 00243 00244 void screen_selection(); /*!< Calls the relevant state_X_screen() depending on the value of g_next_state */ 00245 /// 00246 /// Screen Animation Functions 00247 /// 00248 void setting_animation(); /*!< Prints the animation on the setting screen to the Nokia 5110 LCD */ 00249 00250 void timeout_protocol(); /*!< Prints timeout counter when the alarm is triggered */ 00251 /// 00252 /// Read Distance Functions 00253 /// 00254 void get_setting_distance(); /*!< Gets the distance from the SRF02 and then increments the setting_distance_counter */ 00255 00256 void get_intruder_distance(); /*!< Gets the distance from the SRF02 and then increments the intruder_distance_counter */ 00257 00258 void calculate_setting_distance(); /*!< Averages the past 10 distance readings and stores them in the initial_setting_distance */ 00259 00260 void calculate_intruder_distance(); /*!< Averages the past 10 distance readings and compares this value to the initial_setting_distance to detect a potential intruder */ 00261 /// 00262 /// Timeout Functions 00263 /// 00264 void screen_5_transition(); /*!< Called five seconds after the setting screen is called */ 00265 00266 void pin_confirm(); /*!< Clears the screen when a pin is entered to tell the user to press button 'C' to confirm the entered pin */ 00267 00268 void state_1_transition(); /*!< Sends the user back to state 1 if the device is tampered in the setting state */ 00269 00270 void alarm_setting_buzz(); /*!< Turns off buzzer one second after entering state 5 */ 00271 /// 00272 /// Accelerometer Functions 00273 /// 00274 void get_axis_data(); /*!< Gets the accelerometer data from the K64F on_board accelerometer and stores them in acc_X, acc_Y and acc_Z */ 00275 00276 void compare_axis_data(); /*!< Compares the accelerometer data from the K64F on-board accelerometer with the stored values of setting_acc_X, setting_acc_Y and setting_acc_Z */ 00277 00278 void device_tampered_protocol(); /*!< If the device is moved whilst in the setting state this function is called to stop the alarm from setting */ 00279 /// 00280 /// Pin Functions 00281 /// 00282 void enter_pin(); /*!< Prints '*' to the Nokia 5110 LCD when a pin is being entered */ 00283 00284 void reset_entered_pin(); /*!< Resets the entered_pin array to have elements with a value of -1 */ 00285 00286 void check_pin(); /*!< Compares the entered_pin to the set_pin */ 00287 00288 void change_pin(); /*!< Writes entered_pin to the SD card when a pin is entered in state 3 - adapted from 2545_SD_Card Example by Dr. C.A. Evans */ 00289 00290 void read_pin(); /*!< Reads pin from the SD card to the set_pin array - adapted from 2545_SD_Card Example by Dr. C.A. Evans */ 00291 00292 void delete_file(char filename[]); /*!< Deletes the old pin from the SD card - taken from 2545_SD_Card Example by Dr. C.A. Evans */ 00293 00294 /** 00295 00296 */ 00297 00298 #endif
Generated on Sun Jul 17 2022 01:32:30 by
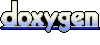