L3GD20 Library using FIFO and Interrupt
Fork of L3GD20_SPI by
Embed:
(wiki syntax)
Show/hide line numbers
L3GD20.h
00001 #ifndef MBED_SPI 00002 #define MBED_SPI 00003 #include "mbed.h" 00004 #include "L3GD20_Resister.h" 00005 /** L3GD20(SPI) class 00006 * 00007 * This is the L3GD20 class. 00008 * @code 00009 #include "mbed.h" 00010 #include "L3GD20.h" 00011 00012 Serial pc(USBTX,USBRX);//tx,rx 00013 L3GD20 L3GD20(p11,p12,p13,p14,p15);//miso,mosi,sck,cs,interrupt 00014 00015 void interruption(anglerrates *val) 00016 { 00017 printf("X:%+05d\tY:%+05d\tZ:%+05d level:%02d\r\n",(val->X),(val->Y),(val->Z),(L3GD20.FIFO.level)); 00018 } 00019 int main() 00020 { 00021 L3GD20.start(L3GD20::XYZ,&interruption); 00022 while(1) { 00023 sleep(); 00024 } 00025 } 00026 * @endcode 00027 */ 00028 class L3GD20 00029 { 00030 public: 00031 L3GD20(PinName mosi, PinName miso, PinName scl, PinName cs,PinName interrupt2=NC); 00032 typedef enum { 00033 null=0x00,WhoAmI=0x0F,CtrlReg1=0x20,CtrlReg2=0x21,CtrlReg3=0x22,CtrlReg4=0x23,CtrlReg5=0x24,Reference=0x25,OutTemp=0x26,StatusReg=0x27,OutXL=0x28,OutXH=0x29,OutYL=0x2A,OutYH=0x2B,OutZL=0x2C,OutZH=0x2D,FIFOCtrlReg=0x2E,FIFOSrcReg=0x2F,INT1Cfg=0x30,INT1Src=0x31,INT1ThsXH=0x32,INT1ThsXL=0x33,INT1ThsYH=0x34,INT1ThsYL=0x35,INT1ThsZH=0x36,INT1ThsZL=0x37,INT1Duration=0x38,READ=0x80 00034 } RESISTER; 00035 /** @enum DIRECTION 00036 * enable direction\n 00037 * Example...L3GD20::XY,L3GD20::XYZ 00038 */ 00039 typedef enum { 00040 Y=0x1, 00041 X=0x1<<1, 00042 Z=0x1<<2, 00043 XY=X|Y, 00044 XZ=X|Z, 00045 YZ=Y|Z, 00046 XYZ=X|Y|Z 00047 } DIRECTION; 00048 /** @enum FIFO_mode 00049 * FIFO mode\n 00050 * Example...L3GD20::FIFOmode\n 00051 * See Datasheet 4.2_FIFO(p.16/44) 00052 */ 00053 typedef enum { 00054 BYPASSmode=0x0,FIFOmode,STREAMmode,STREAMtoFIFOmode,BYPASStoSTREAMmode 00055 } FIFO_mode; 00056 /** 00057 * FIFO status for cause of interruption\n 00058 * Example...L3GD20::watermark\n 00059 * See Datasheet 7.4_CTRL_REG3(p.33/44) 00060 */ 00061 typedef enum { 00062 none=0,empty,watermark,overrun 00063 } FIFOstatus; 00064 /** 00065 * @brief FIFO status info 00066 */ 00067 struct { 00068 FIFOstatus status;///< Type of status is enum "FIFOstatus". cause of interruption(none,empty,watermark,overrun) 00069 int level; ///< FIFO buffer level 00070 } FIFO; 00071 struct config { 00072 //read and write resister 00073 union CTRL_REG1 CTRL_REG1; 00074 union CTRL_REG2 CTRL_REG2; 00075 union CTRL_REG3 CTRL_REG3; 00076 union CTRL_REG4 CTRL_REG4; 00077 union CTRL_REG5 CTRL_REG5; 00078 union REF_DATACAP REF_DATACAP; 00079 union OUT_TEMP OUT_TEMP; 00080 union STATUS_REG STATUS_REG; 00081 union FIFO_CTRL_REG FIFO_CTRL_REG; 00082 union INT1_CFG INT1_CFG; 00083 union INT1_TSH_XH INT1_TSH_XH; 00084 union INT1_TSH_XL INT1_TSH_XL; 00085 union INT1_TSH_YH INT1_TSH_YH; 00086 union INT1_TSH_YL INT1_TSH_YL; 00087 union INT1_TSH_ZH INT1_TSH_ZH; 00088 union INT1_TSH_ZL INT1_TSH_ZL; 00089 union INT1_DURATION INT1_DURATION; 00090 } _config; 00091 struct status { 00092 //read only resister 00093 int OUT_TEMP; 00094 int STATUS_REG; 00095 union FIFO_SRC_REG FIFO_SRC_REG; 00096 int INT1_SRC; 00097 } _status; 00098 //Class method 00099 /** @fn void L3GD20::start(DIRECTION enable); 00100 * Start command send to module 00101 * @param enable 00102 */ 00103 void start(DIRECTION enable); 00104 /** @fn void L3GD20::start(DIRECTION enable,void (*func)(anglerrates*)); 00105 * Start with interrupt 00106 * @param enable L3GD20 channel 00107 * @param func user function(call by InterrtptIn) 00108 */ 00109 void start(DIRECTION enable,void (*func)(anglerrates*)); 00110 /** @fn void L3GD20::stop() 00111 * stop sampling command send 00112 */ 00113 void stop(); 00114 /** @fn void L3GD20::sleep() 00115 * sleep command send 00116 */ 00117 void sleep(); 00118 /** @fn void L3GD20::read(anglerrates* val,DIRECTION direction) 00119 * read angler rates with direction(L3GD20::XY)\n 00120 * This is read and calcurate dps, reading value is set to class instanse 00121 */ 00122 void read(anglerrates* val,DIRECTION direction); 00123 /** @fn int L3GD20::readTemperature() 00124 * read temperature without format 00125 * (I don't know meaning of value.) 00126 */ 00127 int readTemperature(); 00128 /** @fn void L3GD20::enableFIFO(FIFO_mode mode,FIFOstatus interrupt,const int threshold) 00129 * @brief L3GD20mode need to reset(to bypass mode) when filled FIFObuffor 00130 * @param mode FIFOmode(L3GD20::BYPASSmode,FIFOmode,STREAMmode,STREAMtoFIFOmode,BYPASStoSTREAMmode) 00131 * @param interrupt cause of interrupt(L3GD20::none,empty,watermark,overrun) 00132 * @param threshold interruption threshold(1 to 30 vaild) 00133 */ 00134 void enableFIFO(FIFO_mode mode,FIFOstatus interrupt,const int threshold=0); 00135 /** @fn int L3GD20::updateFIFO(void) 00136 * @brief Update FIFO status 00137 * @return FIFO buffer level 00138 */ 00139 int updateFIFO(void); 00140 void allReadOut(); 00141 anglerrates value;///< @brief latest angler rates 00142 protected: 00143 //write command to resister 00144 void write(RESISTER reg,int val); 00145 //read resister for resister 00146 void read(RESISTER reg,int* val); 00147 //just send reboot command 00148 void reboot(); 00149 //reset FIFO To restart data collection, resister must be written back to Bypass mode. 00150 void resetFIFO(); 00151 //read all configration rester 00152 void configReadOut(void); 00153 //read all status rester 00154 void statusReadOut(void); 00155 //for InterruptIn function ,call userFunction in this methed 00156 void interrupt(void); 00157 /* 00158 void datarate(uint8_t rate,uint8_t bandwidth); 00159 void setDataFormat(); 00160 void filter(uint8_t mode,uint8_t frequency); 00161 void channelSource(uint8_t channnel,uint8_t dataSelection,uint8_t interruptSelection); 00162 void FIFO(uint8_t mode,uint8_t watermark); 00163 void interrupt(uint8_t source,uint8_t threthold,uint8_t duration,uint8_t Wait); 00164 */ 00165 void (*userFunction)(anglerrates*); 00166 SPI _spi; 00167 DigitalOut _cs; 00168 InterruptIn _int2; 00169 }; 00170 00171 #endif
Generated on Wed Jul 13 2022 09:18:54 by
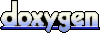