
Final Project files for mBed development.
Embed:
(wiki syntax)
Show/hide line numbers
main.h
Go to the documentation of this file.
00001 /** 00002 * @file main.h 00003 * @brief Main header file for includes and defs 00004 * @author John Wilkey 00005 * @author Alec Guertin 00006 * @author Chester Chu 00007 */ 00008 00009 #ifndef _MAIN_H 00010 #define _MAIN_H 00011 00012 #include "mbed.h" 00013 #include "m3pi.h" 00014 #include <string.h> 00015 #include <stdarg.h> 00016 #include <stdio.h> 00017 #include <math.h> 00018 00019 /* Constants used in main.c */ 00020 #define TURN_SPEED 0.15 /**< Motor power for turning */ 00021 #define DRIVE_SPEED 0.25 /**< Motor power for drawing/moving */ 00022 #define TIME_FACT 1780 /**< Multiplier for forward() and backward() */ 00023 #define CAL_SPEED 0.25 /**< Drive speed during calibration */ 00024 #define CLOSE_ENOUGH 0.0008 /**< Threshold for calibration line centering */ 00025 #define WIGGLE_MAX 30 /**< Max 'wiggles' during calibration */ 00026 #define CORNER_THRESHOLD 0.3 /**< Threshold for checking if line position denotes a corner */ 00027 #define INST_BUF_SIZE 250 /**< Size of input buffer for instructions */ 00028 #define CORRECTION_SPEED 0.2*DRIVE_SPEED /**< Amount to change speed of one wheel when following line */ 00029 #define CORRECTION_THRESHOLD 0.05 /**< Maximum tolerable deviation from line when following line */ 00030 #define RAD_TO_DEG 57.29 /**< Factor to convert radians to degrees */ 00031 #define FULL_TURN 360 /**< Degrees for a full turn */ 00032 #define HALF_TURN 180 /**< Degrees for a half turn */ 00033 #define QUARTER_TURN 90 /**< Degrees for a quarter (right angle) turn */ 00034 #define CAL_FACTOR 1.1 /**< Scaling factor for time to drive */ 00035 #define DEGREE_CORRECTION 0 /**< Amount (in degrees) added to the angle for each turn */ 00036 #define SEC_TO_MSEC 1000 /**< Scaling factor to convert seconds to milliseconds */ 00037 #define SEC_TO_USEC 1000000 /**< Scaling factor to convert seconds to microseconds */ 00038 #define MSEC_TO_USEC 1000 /**< Scaling factor to convert milliseconds to microseconds */ 00039 00040 /** 00041 * @brief get values of next PostScript instruction. 00042 * 00043 * @param buf Buffer with PS instructions. 00044 * @param x Pointer to storage for x coordinate. 00045 * @param y Pointer to storage for y coordinate. 00046 * @param draw Pointer to storage for draw/move boolean. 00047 * 00048 * @return Success or failure code. 00049 */ 00050 int retrieve_inst(char *buf, int *x, int *y, int *draw); 00051 00052 /** 00053 * @brief Driver forward for a time. 00054 * 00055 * @param[in] amt Amount to drive forward. In milliseconds. 00056 */ 00057 void forward(int amt); 00058 00059 /** 00060 * @brief Drive backward for a time. 00061 * 00062 * @param[in] amt Amount to drive backward. In milliseconds. 00063 */ 00064 void backward(int amt); 00065 00066 /** 00067 * @brief Turn right by some angle. 00068 * 00069 * @param[in] deg Desired final turn angle in degrees from start. 00070 * Note that a negative angle will turn in the opposite 00071 * direction. 00072 */ 00073 void right(float deg); 00074 00075 /** 00076 * @brief Turn left by some angle. 00077 * 00078 * @param[in] deg Desired final turn angle in degrees from start. 00079 * Note that a negative angle will turn in the opposite 00080 * direction. 00081 */ 00082 void left (float deg); 00083 00084 /** 00085 * @brief Wait for a number of seconds, possibly fractional. 00086 * 00087 * Timer resolution is on the order of microseconds. A negative wait 00088 * time does nothing. 00089 * 00090 * @param[in] amt Time to wait, in seconds. 00091 */ 00092 void timerWait(float amt); 00093 00094 /** 00095 * @brief Follows the non-reflective line to a corner 00096 * 00097 * @param[in] left boolean specifying if the corner to look for 00098 * is a left corner 00099 * 00100 * @return returns an error code 00101 */ 00102 int find_corner(int left); 00103 00104 /** 00105 * @brief Turns towards the base line and moves to reach it 00106 */ 00107 void find_line(); 00108 00109 /** 00110 * @brief Computes the Euclidean distance between two points 00111 * 00112 * @param[in] x1 the x-coordinate of the first point 00113 * @param[in] y1 the y-coordinate of the first point 00114 * @param[in] x2 the x-coordinate of the second point 00115 * @param[in] y2 the y-coordinate of the second point 00116 * 00117 * @return the distance between points (x1,y1) and (x2,y2) 00118 */ 00119 float distance(int x1, int y1, int x2, int y2); 00120 00121 /** 00122 * @brief prints to the m3pi's screen 00123 * 00124 * @param[in] line the line on the screen to print to, in {0,1} 00125 * @param[in] format the format string to print 00126 * @param[in] ... the arguments to pass into the format string 00127 */ 00128 void robot_printf(int line, const char *format, ...); 00129 00130 /** 00131 * @brief computes the angle to turn to face towards (x,y) 00132 * 00133 * @param[in] last_x the current x-coordinate 00134 * @param[in] last_y the current y-coordinate 00135 * @param[in] x the desired x-coordinate 00136 * @param[in] y the desired y-coordinate 00137 * @param[in] angle the current angle the m3pi is facing 00138 * 00139 * @return the angle to turn left 00140 */ 00141 float compute_turn_angle(int last_x, int last_y, int x, int y, float angle); 00142 00143 /** 00144 * @brief sends the signal on the output pin for the led 00145 * to turn it on 00146 */ 00147 void pen_up(); 00148 00149 /** 00150 * @brief turns off the signal to the led 00151 */ 00152 void pen_down(); 00153 00154 #endif
Generated on Mon Jul 18 2022 15:37:44 by
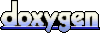