Release 1.01
Embed:
(wiki syntax)
Show/hide line numbers
mcp23s08.h
00001 /**************************************************************************//** 00002 * @file mcp23s08.cpp 00003 * @brief Base class for wrapping the interface with the GPIO Extender . 00004 * @version: V1.0 00005 * @date: 9/17/2019 00006 00007 * 00008 * @note 00009 * This is Third party software adapted to the ESCM project. 00010 * See copyright below. 00011 * 00012 * @par 00013 * E3 Designers LLC is supplying this software for use with Cortex-M3 LPC1768 00014 * processor based microcontroller for the ESCM 2000 Monitor and Display. 00015 * * 00016 * @par 00017 * THIS SOFTWARE IS PROVIDED "AS IS". NO WARRANTIES, WHETHER EXPRESS, IMPLIED 00018 * OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00019 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. 00020 * ARM SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL, OR 00021 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00022 * 00023 ******************************************************************************/ 00024 00025 /* 00026 ___ _ _ _ __ ___ ___ | |_ ___ _ _ 00027 / __|| | | || '_ ` _ \ / _ \ | __|/ _ \ | | | | 00028 \__ \| |_| || | | | | || (_) || |_| (_) || |_| | 00029 |___/ \__,_||_| |_| |_| \___/ \__|\___/ \__, | 00030 |___/ 00031 00032 gpio_expander - An attemp to create a fast and universal library for drive many GPIO chips 00033 00034 model: company: pins: protocol: Special Features: 00035 --------------------------------------------------------------------------------------------------------------------- 00036 mcp23s08 Microchip 8 SPI INT/HAEN 00037 --------------------------------------------------------------------------------------------------------------------- 00038 Version history: 00039 0.5b1: first release, just coded and never tested 00040 0.5b2: fixed 2wire version, added portPullup, tested output mode (ok) 00041 0.5b3: added some drivers 00042 0.5b4: ability to include library inside other libraries. 00043 0.5b7: Changed functionalities of some function. 00044 0.6b1: Changed gpioRegisterRead to gpioRegisterReadByte. Added gpioRegisterReadWord (for some GPIO) 00045 0.6b3: Added basic support for SPI transactions, small optimizations. 00046 0.8b3: Added 2 more commands and 2 gpio chip. 00047 0.8b4: Support for SPI Transaction post setup 00048 --------------------------------------------------------------------------------------------------------------------- 00049 Copyright (c) 2013-2014, s.u.m.o.t.o.y [sumotoy(at)gmail.com] 00050 --------------------------------------------------------------------------------------------------------------------- 00051 gpio_expander Library is free software: you can redistribute it and/or modify 00052 it under the terms of the GNU General Public License as published by 00053 the Free Software Foundation, either version 3 of the License, or 00054 (at your option) any later version. 00055 gpio_expander Library is distributed in the hope that it will be useful, 00056 but WITHOUT ANY WARRANTY; without even the implied warranty of 00057 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00058 GNU General Public License for more details. 00059 You should have received a copy of the GNU General Public License 00060 along with Foobar. If not, see <http://www.gnu.org/licenses/>. 00061 00062 +++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00063 Version:0.8b3: Added 2 more commands and 2 gpio chip. 00064 +++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00065 */ 00066 00067 /* ------------------------------ MCP23S08 WIRING ------------------------------------ 00068 This chip has a very useful feature called HAEN that allow you to share the same CS pin trough 00069 4 different addresses. Of course chip has to be Microchip and should be assigned to different addresses! 00070 Basic Address: 001000 A1 A0 (from 0x20 to 0x23) 00071 A1,A0 tied to ground = 0x20 00072 __ __ 00073 -> sck [| U |] ++++ 00074 -> mosi [| |] IO-7 00075 <- miso [| |] IO-6 00076 A1 [| |] IO-5 00077 A0 [| |] IO-4 00078 rst (con.+) [| |] IO-3 00079 cs [| |] IO-2 00080 int [| |] IO-1 00081 GND [|_____|] IO-0 00082 */ 00083 #ifndef _MCP23S08_H_ 00084 #define _MCP23S08_H_ 00085 00086 #include <inttypes.h> 00087 00088 class mcp23s08 : public SPI 00089 { 00090 00091 public: 00092 mcp23s08(PinName mosi, PinName miso, PinName clk, PinName cs_pin,const uint8_t haenAdrs); 00093 void postSetup(const uint8_t haenAdrs);//used with other libraries only 00094 virtual void begin(bool protocolInitOverride=false); //protocolInitOverride=true will not init the SPI 00095 00096 00097 void gpioPinMode(uint8_t mode); //set all pins to INPUT or OUTPUT 00098 void gpioPinMode(uint8_t pin, bool mode); //set a unique pin as IN(1) or OUT (0) 00099 void gpioPort(uint8_t value); //write data to all pins 00100 //void gpioPort(uint8_t lowByte, uint8_t highByte); //same as abowe but uses 2 separate bytes (not applicable to this chip) 00101 uint8_t readGpioPort(); //read the state of the pins (all) 00102 uint8_t readGpioPortFast(); 00103 00104 void gpioDigitalWrite(uint8_t pin, bool value); //write data to one pin 00105 void gpioDigitalWriteFast(uint8_t pin, bool value); 00106 int gpioDigitalRead(uint8_t pin); //read data from one pin 00107 uint8_t gpioRegisterReadByte(uint8_t reg); //read a uint8_t from chip register 00108 int gpioDigitalReadFast(uint8_t pin); 00109 void gpioRegisterWriteByte(uint8_t reg,uint8_t data); //write a chip register 00110 void portPullup(uint8_t data); // true=pullup, false=pulldown all pins 00111 void gpioPortUpdate(); 00112 // direct access commands 00113 uint8_t readAddress(uint8_t addr); 00114 00115 void setSPIspeed(uint32_t spispeed);//for SPI transactions 00116 00117 //------------------------- REGISTERS 00118 uint8_t IOCON; 00119 uint8_t IODIR; 00120 uint8_t GPPU; 00121 uint8_t GPIO; 00122 uint8_t GPINTEN; 00123 uint8_t IPOL; 00124 uint8_t DEFVAL; 00125 uint8_t INTF; 00126 uint8_t INTCAP; 00127 uint8_t OLAT; 00128 uint8_t INTCON; 00129 00130 private: 00131 DigitalOut cs; 00132 00133 uint8_t _cs; 00134 uint8_t _adrs; 00135 00136 uint32_t _spiTransactionsSpeed;//for SPI transactions 00137 00138 uint8_t _useHaen; 00139 uint8_t _readCmd; 00140 uint8_t _writeCmd; 00141 void startSend(bool mode); 00142 void endSend(); 00143 uint8_t _gpioDirection; 00144 uint8_t _gpioState; 00145 void writeByte(uint8_t addr, uint8_t data); 00146 }; 00147 #endif
Generated on Wed Jul 20 2022 06:07:03 by
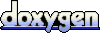