Release 1.01
Embed:
(wiki syntax)
Show/hide line numbers
mcp23s08.cpp
Go to the documentation of this file.
00001 /**************************************************************************//** 00002 * @file mcp23s08.cpp 00003 * @brief Base class for wrapping the interface with the GPIO Extender . 00004 * @version: V1.0 00005 * @date: 9/17/2019 00006 00007 * 00008 * @note 00009 * Copyright (C) 2019 E3 Design. All rights reserved. 00010 * 00011 * @par 00012 * E3 Designers LLC is supplying this software for use with Cortex-M3 LPC1768 00013 * processor based microcontroller for the ESCM 2000 Monitor and Display. 00014 * * 00015 * @par 00016 * THIS SOFTWARE IS PROVIDED "AS IS". NO WARRANTIES, WHETHER EXPRESS, IMPLIED 00017 * OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. 00019 * ARM SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL, OR 00020 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00021 * 00022 ******************************************************************************/ 00023 #include "mbed.h" 00024 #include "mcp23s08.h" 00025 00026 #define INPUT 0 00027 #define OUTPUT 1 00028 00029 #define LOW 0 00030 #define HIGH 1 00031 00032 mcp23s08::mcp23s08(PinName mosi, PinName miso, PinName clk, PinName cs_pin,const uint8_t haenAdrs) : SPI(mosi, miso, clk), cs(cs_pin) { 00033 format(8, 3); 00034 frequency(2000000); 00035 00036 postSetup(haenAdrs); 00037 00038 } 00039 00040 00041 void mcp23s08::postSetup(const uint8_t haenAdrs){ 00042 if (haenAdrs >= 0x20 && haenAdrs <= 0x23){//HAEN works between 0x20...0x23 00043 _adrs = haenAdrs; 00044 _useHaen = 1; 00045 } else { 00046 _adrs = 0; 00047 _useHaen = 0; 00048 } 00049 _readCmd = (_adrs << 1) | 1; 00050 _writeCmd = _adrs << 1; 00051 //setup register values for this chip 00052 IOCON = 0x05; 00053 IODIR = 0x00; 00054 GPPU = 0x06; 00055 GPIO = 0x09; 00056 GPINTEN = 0x02; 00057 IPOL = 0x01; 00058 DEFVAL = 0x03; 00059 INTF = 0x07; 00060 INTCAP = 0x08; 00061 OLAT = 0x0A; 00062 INTCON = 0x04; 00063 } 00064 00065 void mcp23s08::begin(bool protocolInitOverride) { 00066 00067 cs=1; 00068 wait(0.1); 00069 _useHaen == 1 ? writeByte(IOCON,0b00101000) : writeByte(IOCON,0b00100000); 00070 /* 00071 if (_useHaen){ 00072 writeByte(IOCON,0b00101000);//read datasheet for details! 00073 } else { 00074 writeByte(IOCON,0b00100000); 00075 } 00076 */ 00077 _gpioDirection = 0xFF;//all in 00078 _gpioState = 0x00;//all low 00079 } 00080 00081 00082 uint8_t mcp23s08::readAddress(uint8_t addr){ 00083 uint8_t low_byte = 0x00; 00084 startSend(1); 00085 SPI::write(addr); 00086 low_byte = (uint8_t)SPI::write(0x00); 00087 endSend(); 00088 return low_byte; 00089 } 00090 00091 00092 00093 void mcp23s08::gpioPinMode(uint8_t mode){ 00094 if (mode == INPUT){ 00095 _gpioDirection = 0xFF; 00096 } else if (mode == OUTPUT){ 00097 _gpioDirection = 0x00; 00098 _gpioState = 0x00; 00099 } else { 00100 _gpioDirection = mode; 00101 } 00102 writeByte(IODIR,_gpioDirection); 00103 } 00104 00105 void mcp23s08::gpioPinMode(uint8_t pin, bool mode){ 00106 if (pin < 8){//0...7 00107 mode == INPUT ? _gpioDirection |= (1 << pin) :_gpioDirection &= ~(1 << pin); 00108 writeByte(IODIR,_gpioDirection); 00109 } 00110 } 00111 00112 void mcp23s08::gpioPort(uint8_t value){ 00113 if (value == HIGH){ 00114 _gpioState = 0xFF; 00115 } else if (value == LOW){ 00116 _gpioState = 0x00; 00117 } else { 00118 _gpioState = value; 00119 } 00120 writeByte(GPIO,_gpioState); 00121 } 00122 00123 00124 uint8_t mcp23s08::readGpioPort(){ 00125 return readAddress(GPIO); 00126 } 00127 00128 uint8_t mcp23s08::readGpioPortFast(){ 00129 return _gpioState; 00130 } 00131 00132 int mcp23s08::gpioDigitalReadFast(uint8_t pin){ 00133 if (pin < 8){//0...7 00134 int temp = _gpioState & (1 << pin); 00135 return temp; 00136 } else { 00137 return 0; 00138 } 00139 } 00140 00141 void mcp23s08::portPullup(uint8_t data) { 00142 if (data == HIGH){ 00143 _gpioState = 0xFF; 00144 } else if (data == LOW){ 00145 _gpioState = 0x00; 00146 } else { 00147 _gpioState = data; 00148 } 00149 writeByte(GPPU, _gpioState); 00150 } 00151 00152 00153 00154 00155 void mcp23s08::gpioDigitalWrite(uint8_t pin, bool value){ 00156 if (pin < 8){//0...7 00157 value == HIGH ? _gpioState |= (1 << pin) : _gpioState &= ~(1 << pin); 00158 writeByte(GPIO,_gpioState); 00159 } 00160 } 00161 00162 void mcp23s08::gpioDigitalWriteFast(uint8_t pin, bool value){ 00163 if (pin < 8){//0...8 00164 value == HIGH ? _gpioState |= (1 << pin) : _gpioState &= ~(1 << pin); 00165 } 00166 } 00167 00168 void mcp23s08::gpioPortUpdate(){ 00169 writeByte(GPIO,_gpioState); 00170 } 00171 00172 int mcp23s08::gpioDigitalRead(uint8_t pin){ 00173 if (pin < 8) return (int)(readAddress(GPIO) & 1 << pin); 00174 return 0; 00175 } 00176 00177 uint8_t mcp23s08::gpioRegisterReadByte(uint8_t reg){ 00178 uint8_t data = 0; 00179 startSend(1); 00180 SPI::write(reg); 00181 data = (uint8_t)SPI::write(0x00); 00182 endSend(); 00183 return data; 00184 } 00185 00186 00187 void mcp23s08::gpioRegisterWriteByte(uint8_t reg,uint8_t data){ 00188 writeByte(reg,(uint8_t)data); 00189 } 00190 00191 /* ------------------------------ Low Level ----------------*/ 00192 void mcp23s08::startSend(bool mode){ 00193 cs=0; 00194 mode == 1 ? SPI::write(_readCmd) : SPI::write(_writeCmd); 00195 } 00196 00197 void mcp23s08::endSend(){ 00198 cs=1; 00199 } 00200 00201 00202 void mcp23s08::writeByte(uint8_t addr, uint8_t data){ 00203 startSend(0); 00204 SPI::write(addr); 00205 SPI::write(data); 00206 endSend(); 00207 }
Generated on Wed Jul 20 2022 06:07:03 by
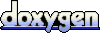