Release 1.01
Embed:
(wiki syntax)
Show/hide line numbers
SPI_MX25R.h
00001 /**************************************************************************//** 00002 * @file SPI_MX25R.cpp 00003 * @brief Base class for wrapping the interface with the SPI NOR Flash. 00004 * @version: V1.0 00005 * @date: 9/17/2019 00006 * 00007 * @note 00008 * SPI_MX25R Series SPI-Flash Memory 00009 * Macronix Low Power Serial NOR Flash 00010 * (x2, and x4 I/O modes not implemented) 00011 * 00012 * 00013 * @note 00014 * Copyright (C) 2019 E3 Design. All rights reserved. 00015 * 00016 * @par 00017 * E3 Designers LLC is supplying this software for use with Cortex-M3 LPC1768 00018 * processor based microcontroller for the ESCM 2000 Monitor and Display. 00019 * * 00020 * @par 00021 * THIS SOFTWARE IS PROVIDED "AS IS". NO WARRANTIES, WHETHER EXPRESS, IMPLIED 00022 * OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00023 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. 00024 * ARM SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL, OR 00025 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00026 * 00027 ******************************************************************************/ 00028 #ifndef _SPI_MX25R_H_ 00029 #define _SPI_MX25R_H_ 00030 00031 #include "mbed.h" 00032 00033 /** 00034 * Macronix Serial Flash Low Power Memories 00035 * SPI_MX25R Series SPI-Flash Memory 00036 */ 00037 #define CS_LOW 0 // SPI CS# (Chip Select) Setting 00038 #define CS_HIGH 1 // SPI CS# (Chip Select) Setting 00039 #define DUMMY 0x00 // Dummy byte which can be changed to any value 00040 /** 00041 * MX25R Series Register Command Table. 00042 * x2 and x4 commands not currently supported with FRDM K64F platform 00043 */ 00044 #define CMD_READ 0x03 // x1 Normal Read Data Byte 00045 #define CMD_FREAD 0x0B // x1 Fast Read Data Byte 00046 #define CMD_2READ 0xBB // x2 2READ 00047 #define CMD_DREAD 0x3B // x2 DREAD 00048 #define CMD_4READ 0xEB // x4 4READ 00049 #define CMD_QREAD 0x6B // x4 QREAD 00050 #define CMD_PP 0x02 // Page Program 00051 #define CMD_4PP 0x38 // x4 PP 00052 #define CMD_SE 0x20 // 4KB Sector Erase 00053 #define CMD_32KBE 0x52 // 32KB Block Erase 00054 #define CMD_BE 0xD8 // 64KB Block Erase 00055 #define CMD_CE 0xC7 // Chip Erase 00056 #define CMD_RDSFDP 0x5A // Read SFDP 00057 #define CMD_WREN 0x06 // Write Enable 00058 #define CMD_WRDI 0x04 // Write Disable 00059 #define CMD_RDSR 0x05 // Read Status Register 00060 #define CMD_RDCR 0x15 // Read Configuration Register 00061 #define CMD_WRSR 0x01 // Write Status Register 00062 #define CMD_PESUS 0xB0 // Program/Erase Suspend 00063 #define CMD_PERES 0x30 // Program/Erase Resume 00064 #define CMD_DP 0xB9 // Enter Deep Power Down 00065 #define CMD_SBL 0xC0 // Set Burst Length 00066 #define CMD_RDID 0x9F // Read Manufacturer and JDEC Device ID 00067 #define CMD_REMS 0x90 // Read Electronic Manufacturer and Device ID 00068 #define CMD_RES 0xAB // Read Electronic ID 00069 #define CMD_ENSO 0xB1 // Enter Secure OTP 00070 #define CMD_EXSO 0xC1 // Exit Secure OTP 00071 #define CMD_RDSCUR 0x2B // Read Security Register 00072 #define CMD_WRSCUR 0x2F // Write Security Register 00073 #define CMD_NOP 0x00 // No Operation 00074 #define CMD_RSTEN 0x66 // Reset Enable 00075 #define CMD_RST 0x99 // Reset 00076 #define CMD_RRE 0xFF // Release Read Enhanced Mode 00077 00078 00079 class SPI_MX25R 00080 { 00081 public: 00082 /** 00083 * Macronix MX25R Low Power and Wide Vcc SPI-Flash Memory Family 00084 * 00085 * @param SI/SIO0 SPI_MOSI pin 00086 * @param SO/SI01 SPI_MISO pin 00087 * @param SCLK SPI_CLK pin 00088 * @param CSb SPI_CS pin 00089 */ 00090 SPI_MX25R(PinName mosi, PinName miso, PinName sclk, PinName cs) ; 00091 00092 ~SPI_MX25R() ; 00093 00094 SPI m_spi; 00095 DigitalOut m_cs ; 00096 int _mode ; 00097 00098 /// Write Enable 00099 void writeEnable(void) ; 00100 00101 /// Write Disable 00102 void writeDisable(void) ; 00103 00104 /// Reset Enable 00105 void resetEnable(void) ; 00106 00107 /// Reset 00108 void reset(void) ; 00109 00110 /// Program or Erase Suspend 00111 void pgmersSuspend(void) ; 00112 00113 /// Program or Erase Resume 00114 void pgmersResume(void) ; 00115 00116 /// Enter Deep Power Down 00117 void deepPowerdown(void) ; 00118 00119 /// Set Burst Length 00120 void setBurstlength(void) ; 00121 00122 /// Release from Read Enhanced Mode 00123 void releaseReadenhaced(void) ; 00124 00125 /// No Operation 00126 void noOperation(void) ; 00127 00128 /// Enter OTP Area 00129 void enterSecureOTP(void) ; 00130 00131 /// Exit OTP Area 00132 void exitSecureOTP(void) ; 00133 00134 /// Chip Erase 00135 void chipErase(void) ; 00136 00137 /// Write Status and Configuration Reg 1 and 2 00138 void writeStatusreg(int addr) ; 00139 00140 /// Write Security Reg 00141 void writeSecurityreg(int addr) ; 00142 00143 /** Page Program 00144 * 00145 * @param int addr start address 00146 * @param uint8_t *data data buffer 00147 * @param int numData the number of data to be written 00148 */ 00149 void programPage(int addr, uint8_t *data, int numData) ; 00150 00151 /** Sector Erase 00152 * 00153 * @param int addr specify the sector to be erased 00154 */ 00155 void sectorErase(int addr) ; 00156 00157 /** Block Erase 00158 * 00159 * @param int addr specify the sector to be erased 00160 */ 00161 void blockErase(int addr) ; 00162 00163 /** 32KB Block Erase 00164 * 00165 * @param int addr specify the sector to be erased 00166 */ 00167 void blockErase32KB(int addr) ; 00168 00169 /** Read Status Register 00170 * 00171 * @returns uint8_t status register value 00172 */ 00173 uint8_t readStatus(void) ; 00174 00175 /** Read Security Register 00176 * 00177 * @returns uint8_t security register value 00178 */ 00179 uint8_t readSecurity(void) ; 00180 00181 /** Read Manufacturer and JEDEC Device ID 00182 * 00183 * @returns uint32_t Manufacturer ID, Mem Type, Device ID 00184 */ 00185 uint32_t readID(void) ; 00186 00187 /** Read Electronic Manufacturer and Device ID 00188 * 00189 * @returns uint32_t Manufacturer ID, Device ID 00190 */ 00191 uint32_t readREMS(void) ; 00192 00193 /** Read Electronic ID 00194 * 00195 * @returns uint8_t Device ID 00196 */ 00197 uint8_t readRES(void) ; 00198 00199 /** Read Configuration Register 00200 * 00201 * @returns uint32_t configuration register value 00202 */ 00203 uint32_t readConfig(void) ; 00204 uint8_t readSFDP(int addr) ; 00205 uint8_t readFREAD(int addr) ; 00206 uint8_t read8(int addr) ; 00207 void write8(int addr, uint8_t data) ; 00208 private: 00209 00210 } ; 00211 #endif // _SPI_MX25R_H_
Generated on Wed Jul 20 2022 06:07:03 by
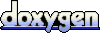