Release 1.01
Embed:
(wiki syntax)
Show/hide line numbers
ButtonController.h
Go to the documentation of this file.
00001 /**************************************************************************//** 00002 * @file ButtonController.h 00003 * @brief Base class for wrapping the interface with the GPIO Extender. 00004 * @version: V1.0 00005 * @date: 9/17/2019 00006 00007 * 00008 * @note 00009 * Copyright (C) 2019 E3 Design. All rights reserved. 00010 * 00011 * @par 00012 * E3 Designers LLC is supplying this software for use with Cortex-M3 LPC1768 00013 * processor based microcontroller for the ESCM 2000 Monitor and Display. 00014 * * 00015 * @par 00016 * THIS SOFTWARE IS PROVIDED "AS IS". NO WARRANTIES, WHETHER EXPRESS, IMPLIED 00017 * OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. 00019 * ARM SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL, OR 00020 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00021 * 00022 ******************************************************************************/ 00023 00024 #ifndef _BUTTON_CONTROLLER_ 00025 #define _BUTTON_CONTROLLER_ 00026 00027 #include "mbed.h" 00028 #include "rtos.h" 00029 #include "mcp23s08.h" 00030 #include "Menu.h" 00031 00032 /** 00033 * service to manage the external GPIO expander board. 00034 */ 00035 typedef enum 00036 { 00037 NO_BUTTON = 27, 00038 BUTTON_UP = 26, 00039 BUTTON_DOWN = 25, 00040 BUTTON_MODE = 19, 00041 BUTTON_SET = 11, 00042 BUTTON_CLEAR = 0 00043 } tButtonValue ; 00044 00045 typedef void (*t_ButtonPressCallback)(void); 00046 00047 00048 /*** 00049 * 00050 * This class wrapps the communication with the GPIO expander over SPI bus. 00051 * PIN 0 : Up 00052 * PIN 1 : Down 00053 * PIN 2 : Clear 00054 * PIN 3 : Mode 00055 * PIN 4 : Set 00056 * PIN 5 : Enable Audio 00057 * PIN 6 : na 00058 * PIN 7 : na 00059 * 00060 * MASK = 0x1F; 00061 * 00062 */ 00063 class ButtonController 00064 { 00065 public : 00066 00067 mcp23s08 * spi_io_exp; 00068 Mutex _mutex; 00069 unsigned char currentValue; 00070 unsigned char prevValue; 00071 unsigned char confirmedValue; 00072 unsigned int countsSinceChange; 00073 unsigned char isServiced; 00074 unsigned char isHeld; 00075 00076 00077 CircularBuffer<uint8_t, 64> cmd_queue; 00078 00079 ButtonController(); 00080 ~ButtonController(); 00081 00082 void pressButtonUp(void); 00083 void pressButtonDown(void); 00084 void pressButtonClear(void); 00085 void pressButtonMode(void); 00086 void pressButtonSet(void); 00087 void releaseButton(void); 00088 00089 void init(void); 00090 void update(void); 00091 void processCmdQueue(Menu * activeMenu); 00092 00093 uint8_t getCurrentState (); 00094 }; 00095 00096 00097 #endif
Generated on Wed Jul 20 2022 06:07:03 by
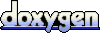