Release 1.01
Embed:
(wiki syntax)
Show/hide line numbers
ButtonController.cpp
Go to the documentation of this file.
00001 /**************************************************************************//** 00002 * @file ButtonController.cpp 00003 * @brief Base class for wrapping the interface with the GPIO Extender. 00004 * @version: V1.0 00005 * @date: 9/17/2019 00006 00007 * 00008 * @note 00009 * Copyright (C) 2019 E3 Design. All rights reserved. 00010 * 00011 * @par 00012 * E3 Designers LLC is supplying this software for use with Cortex-M3 LPC1768 00013 * processor based microcontroller for the ESCM 2000 Monitor and Display. 00014 * * 00015 * @par 00016 * THIS SOFTWARE IS PROVIDED "AS IS". NO WARRANTIES, WHETHER EXPRESS, IMPLIED 00017 * OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. 00019 * ARM SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL, OR 00020 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00021 * 00022 ******************************************************************************/ 00023 #include "ButtonController.h" 00024 00025 00026 /******************************************************************************/ 00027 ButtonController::ButtonController() 00028 { 00029 00030 spi_io_exp = new mcp23s08 (p5, p6, p7, p26, 0x20); 00031 00032 // --------------------------------------- 00033 // set pin modes 00034 // --------------------------------------- 00035 spi_io_exp->gpioPinMode(0,0); 00036 spi_io_exp->gpioPinMode(1,0); 00037 spi_io_exp->gpioPinMode(2,0); 00038 spi_io_exp->gpioPinMode(3,0); 00039 spi_io_exp->gpioPinMode(4,0); 00040 spi_io_exp->gpioPinMode(5,1); 00041 spi_io_exp->gpioPinMode(6,1); 00042 spi_io_exp->gpioPinMode(7,1); 00043 00044 // --------------------------------------- 00045 // enable the audio amplifier 00046 // --------------------------------------- 00047 spi_io_exp->gpioPinMode(5,1); 00048 spi_io_exp->gpioDigitalWrite(5,1); 00049 spi_io_exp->gpioDigitalWrite(6,0); 00050 spi_io_exp->gpioDigitalWrite(7,0); 00051 00052 } 00053 00054 /******************************************************************************/ 00055 ButtonController::~ButtonController() 00056 { 00057 00058 } 00059 00060 /******************************************************************************/ 00061 void ButtonController::init(void) 00062 { 00063 00064 } 00065 00066 /******************************************************************************/ 00067 void ButtonController::processCmdQueue(Menu * activeMenu) 00068 { 00069 while (!cmd_queue.empty()) { 00070 00071 uint8_t e; 00072 00073 cmd_queue.pop(e); 00074 00075 printf("\n<%02x>", e); 00076 00077 switch(e) { 00078 case 0x01://up 00079 activeMenu->pressUp(); 00080 break; 00081 00082 case 0x02://down 00083 activeMenu->pressDown(); 00084 break; 00085 00086 case 0x04://clear 00087 activeMenu->pressClear(); 00088 break; 00089 00090 case 0x08://mode 00091 activeMenu->pressMode(); 00092 break; 00093 00094 case 0x10://set 00095 activeMenu->pressSet(); 00096 break; 00097 default: 00098 break; 00099 } 00100 } 00101 } 00102 00103 00104 /******************************************************************************/ 00105 uint8_t ButtonController::getCurrentState() 00106 { 00107 currentValue = spi_io_exp->readGpioPort(); 00108 00109 return currentValue; 00110 } 00111 00112 00113 /******************************************************************************/ 00114 void ButtonController::update(void) 00115 { 00116 00117 int trigger_action = 0; 00118 00119 currentValue = spi_io_exp->readGpioPort() ; 00120 currentValue = (~currentValue & 0x1F); // clear bit is stuck 00121 00122 // if (raising edge | falling edge ) 00123 if (currentValue != prevValue ) { 00124 prevValue = currentValue; 00125 countsSinceChange = 0; 00126 trigger_action = 1; 00127 00128 } 00129 else 00130 { //TODO: play with time for what is short or long hold 00131 countsSinceChange++; 00132 if ( currentValue != NO_BUTTON && countsSinceChange>=10000) 00133 { 00134 if (isHeld != 2) printf("Button Long Held:%d\n\r",currentValue); 00135 isHeld = 2; 00136 trigger_action = 1; 00137 countsSinceChange = 0; 00138 } 00139 else if (currentValue != NO_BUTTON && countsSinceChange>=5000) 00140 { 00141 if (isHeld != 1) printf("Button Short Held:%d\n\r",currentValue); 00142 isHeld = 1; 00143 trigger_action = 1; 00144 countsSinceChange = 0; 00145 } 00146 else 00147 { 00148 isHeld =0; 00149 } 00150 } 00151 00152 00153 if (trigger_action) 00154 { 00155 if (currentValue && !cmd_queue.full()) { 00156 cmd_queue.push(currentValue); 00157 } 00158 trigger_action = 0; 00159 00160 } 00161 } 00162 00163 00164 /******************************************************************************/ 00165 void ButtonController::pressButtonUp(void) 00166 { 00167 printf("Up Button Pressed:%d\n\r",currentValue); 00168 } 00169 00170 /******************************************************************************/ 00171 void ButtonController::pressButtonDown(void) 00172 { 00173 printf("Down Button Pressed:%d\n\r",currentValue); 00174 } 00175 00176 /******************************************************************************/ 00177 void ButtonController::pressButtonSet(void) 00178 { 00179 printf("Set Button Pressed:%d\n\r",currentValue); 00180 } 00181 00182 /******************************************************************************/ 00183 void ButtonController::pressButtonMode(void) 00184 { 00185 printf("Mode Button Pressed:%d\n\r",currentValue); 00186 } 00187 00188 /******************************************************************************/ 00189 void ButtonController::pressButtonClear(void) 00190 { 00191 printf("Clear Button Pressed:%d\n\r",currentValue); 00192 } 00193 00194 /******************************************************************************/ 00195 void ButtonController::releaseButton(void) 00196 { 00197 //printf("Button Released:%d\n\r",currentValue); 00198 isHeld = 0; 00199 countsSinceChange = 0; 00200 } 00201 /******************************************************************************/
Generated on Wed Jul 20 2022 06:07:03 by
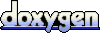