
ZigBee Modem Status example for mbed XBeeLib By Digi
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /** 00002 * Copyright (c) 2015 Digi International Inc., 00003 * All rights not expressly granted are reserved. 00004 * 00005 * This Source Code Form is subject to the terms of the Mozilla Public 00006 * License, v. 2.0. If a copy of the MPL was not distributed with this file, 00007 * You can obtain one at http://mozilla.org/MPL/2.0/. 00008 * 00009 * Digi International Inc. 11001 Bren Road East, Minnetonka, MN 55343 00010 * ======================================================================= 00011 */ 00012 00013 #include "mbed.h" 00014 #include "XBeeLib.h" 00015 #if defined(ENABLE_LOGGING) 00016 #include "DigiLoggerMbedSerial.h" 00017 using namespace DigiLog; 00018 #endif 00019 00020 using namespace XBeeLib; 00021 00022 Serial *log_serial; 00023 00024 /** Callback function, invoked at modem status reception */ 00025 static void modem_status_cb(AtCmdFrame::ModemStatus status) 00026 { 00027 log_serial->printf("\r\nModem Status: 0x%x\r\n", status); 00028 } 00029 00030 int main() 00031 { 00032 log_serial = new Serial(DEBUG_TX, DEBUG_RX); 00033 log_serial->baud(9600); 00034 log_serial->printf("Sample application to demo how to receive modem status changes with the XBeeZB\r\n\r\n"); 00035 log_serial->printf(XB_LIB_BANNER); 00036 00037 #if defined(ENABLE_LOGGING) 00038 new DigiLoggerMbedSerial(log_serial, LogLevelInfo); 00039 #endif 00040 00041 XBeeZB xbee = XBeeZB(RADIO_TX, RADIO_RX, RADIO_RESET, NC, NC, 9600); 00042 00043 /* Register callbacks */ 00044 xbee.register_modem_status_cb(&modem_status_cb); 00045 00046 RadioStatus const radioStatus = xbee.init(); 00047 MBED_ASSERT(radioStatus == Success); 00048 00049 while (true) { 00050 xbee.process_rx_frames(); 00051 wait_ms(100); 00052 log_serial->printf("."); 00053 } 00054 00055 delete(log_serial); 00056 }
Generated on Tue Jul 12 2022 13:12:27 by
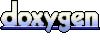