
ZigBee DIOs and ADCs example for mbed XBeeLib By Digi
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /** 00002 * Copyright (c) 2015 Digi International Inc., 00003 * All rights not expressly granted are reserved. 00004 * 00005 * This Source Code Form is subject to the terms of the Mozilla Public 00006 * License, v. 2.0. If a copy of the MPL was not distributed with this file, 00007 * You can obtain one at http://mozilla.org/MPL/2.0/. 00008 * 00009 * Digi International Inc. 11001 Bren Road East, Minnetonka, MN 55343 00010 * ======================================================================= 00011 */ 00012 00013 #include "mbed.h" 00014 #include "XBeeLib.h" 00015 #if defined(ENABLE_LOGGING) 00016 #include "DigiLoggerMbedSerial.h" 00017 using namespace DigiLog; 00018 #endif 00019 00020 #define REMOTE_NODE_ADDR64_MSB ((uint32_t)0x0013A200) 00021 00022 #error "Replace next define with the LSB of the remote module's 64-bit address (SL parameter)" 00023 #define REMOTE_NODE_ADDR64_LSB ((uint32_t)0x01234567) 00024 00025 #define REMOTE_NODE_ADDR64 UINT64(REMOTE_NODE_ADDR64_MSB, REMOTE_NODE_ADDR64_LSB) 00026 00027 using namespace XBeeLib; 00028 00029 Serial *log_serial; 00030 00031 int main() 00032 { 00033 log_serial = new Serial(DEBUG_TX, DEBUG_RX); 00034 log_serial->baud(9600); 00035 log_serial->printf("Sample application to demo how to handle remote XBeeZB devices DIOs and ADCs\r\n\r\n"); 00036 log_serial->printf(XB_LIB_BANNER); 00037 00038 #if defined(ENABLE_LOGGING) 00039 new DigiLoggerMbedSerial(log_serial, LogLevelInfo); 00040 #endif 00041 00042 XBeeZB xbee = XBeeZB(RADIO_TX, RADIO_RX, RADIO_RESET, NC, NC, 9600); 00043 00044 RadioStatus radioStatus = xbee.init(); 00045 MBED_ASSERT(radioStatus == Success); 00046 00047 /* Wait until the device has joined the network */ 00048 log_serial->printf("Waiting for device to join the network: "); 00049 while (!xbee.is_joined()) { 00050 wait_ms(1000); 00051 log_serial->printf("."); 00052 } 00053 log_serial->printf("OK\r\n"); 00054 00055 const RemoteXBeeZB remoteDevice = RemoteXBeeZB(REMOTE_NODE_ADDR64); 00056 00057 radioStatus = xbee.set_pin_config(remoteDevice, XBeeZB::DIO3_AD3, DigitalInput); 00058 MBED_ASSERT(radioStatus == Success); 00059 00060 radioStatus = xbee.set_pin_pull_up(remoteDevice, XBeeZB::DIO3_AD3, true); 00061 MBED_ASSERT(radioStatus == Success); 00062 00063 radioStatus = xbee.set_pin_config(remoteDevice, XBeeZB::DIO4, DigitalOutHigh); 00064 MBED_ASSERT(radioStatus == Success); 00065 00066 radioStatus = xbee.set_pin_config(remoteDevice, XBeeZB::DIO2_AD2, Adc); 00067 MBED_ASSERT(radioStatus == Success); 00068 00069 //#define TEST_SUPPLY_VOLTAGE 00070 00071 #ifdef TEST_SUPPLY_VOLTAGE 00072 /* Set a high V+ value so radio sends Supply Voltage information */ 00073 uint32_t v_value = 0x0C00; 00074 log_serial->printf("\r\nSetting remote device V+ parameter to 0x%04x:\r\n", v_value); 00075 AtCmdFrame::AtCmdResp cmdresp = xbee.set_param(remoteDevice, "V+", v_value); 00076 00077 if (cmdresp == AtCmdFrame::AtCmdRespOk) 00078 log_serial->printf("OK\r\n"); 00079 else 00080 log_serial->printf("FAILED with %d\r\n", (int) cmdresp); 00081 #endif 00082 00083 while(true) { 00084 IOSampleZB ioSample = xbee.get_iosample(remoteDevice); 00085 00086 if (!ioSample.is_valid()) { 00087 log_serial->printf("get_iosample failed, ADC and Digital Input reads will be invalid\r\n"); 00088 } 00089 /* Read DIO3_AD3 digital value */ 00090 DioVal dio3_val; 00091 00092 radioStatus = ioSample.get_dio(XBeeZB::DIO3_AD3, &dio3_val); 00093 MBED_ASSERT(radioStatus == Success); 00094 log_serial->printf("DIO3 value = %d\r\n", dio3_val); 00095 00096 /* Toggle LED associated to DIO4 */ 00097 static bool led_on = false; 00098 if (!led_on) 00099 radioStatus = xbee.set_dio(remoteDevice, XBeeZB::DIO4, Low); 00100 else 00101 radioStatus = xbee.set_dio(remoteDevice, XBeeZB::DIO4, High); 00102 MBED_ASSERT(radioStatus == Success); 00103 led_on = !led_on; 00104 00105 /* Read DIO2_AD2 analog value */ 00106 uint16_t adc2_val; 00107 radioStatus = ioSample.get_adc(XBeeZB::DIO2_AD2, &adc2_val); 00108 MBED_ASSERT(radioStatus == Success); 00109 log_serial->printf("ADC2 value = 0x%04x\r\n", adc2_val); 00110 00111 #ifdef TEST_SUPPLY_VOLTAGE 00112 uint16_t voltaje_val; 00113 radioStatus = ioSample.get_adc(XBeeZB::SUPPLY_VOLTAGE, &voltaje_val); 00114 if (radioStatus == Success) 00115 log_serial->printf("Remote module Supply Voltage = 0x%04x\r\n", voltaje_val); 00116 #endif 00117 00118 log_serial->printf("\r\n"); 00119 00120 wait(5); 00121 } 00122 00123 delete(log_serial); 00124 }
Generated on Tue Jul 12 2022 13:11:21 by
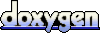