
ZigBee AT Commands example for mbed XBeeLib By Digi
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /** 00002 * Copyright (c) 2015 Digi International Inc., 00003 * All rights not expressly granted are reserved. 00004 * 00005 * This Source Code Form is subject to the terms of the Mozilla Public 00006 * License, v. 2.0. If a copy of the MPL was not distributed with this file, 00007 * You can obtain one at http://mozilla.org/MPL/2.0/. 00008 * 00009 * Digi International Inc. 11001 Bren Road East, Minnetonka, MN 55343 00010 * ======================================================================= 00011 */ 00012 00013 #include "mbed.h" 00014 #include "XBeeLib.h" 00015 #if defined(ENABLE_LOGGING) 00016 #include "DigiLoggerMbedSerial.h" 00017 using namespace DigiLog; 00018 #endif 00019 00020 #define REMOTE_NODE_ADDR64_MSB ((uint32_t)0x0013A200) 00021 00022 #error "Replace next define with the LSB of the remote module's 64-bit address (SL parameter)" 00023 #define REMOTE_NODE_ADDR64_LSB ((uint32_t)0x01234567) 00024 00025 #define REMOTE_NODE_ADDR64 UINT64(REMOTE_NODE_ADDR64_MSB, REMOTE_NODE_ADDR64_LSB) 00026 00027 using namespace XBeeLib; 00028 00029 Serial *log_serial; 00030 00031 int main() 00032 { 00033 log_serial = new Serial(DEBUG_TX, DEBUG_RX); 00034 log_serial->baud(9600); 00035 log_serial->printf("Sample application to demo how to read and set local and remote AT parameters with XBeeZB\r\n\r\n"); 00036 log_serial->printf(XB_LIB_BANNER); 00037 00038 #if defined(ENABLE_LOGGING) 00039 new DigiLoggerMbedSerial(log_serial, LogLevelInfo); 00040 #endif 00041 00042 XBeeZB xbee = XBeeZB(RADIO_TX, RADIO_RX, RADIO_RESET, NC, NC, 9600); 00043 00044 RadioStatus radioStatus = xbee.init(); 00045 MBED_ASSERT(radioStatus == Success); 00046 00047 /* Wait until the device has joined the network */ 00048 log_serial->printf("Waiting for device to join the network: "); 00049 while (!xbee.is_joined()) { 00050 wait_ms(1000); 00051 log_serial->printf("."); 00052 } 00053 log_serial->printf("OK\r\n"); 00054 00055 AtCmdFrame::AtCmdResp cmdresp; 00056 00057 uint32_t value; 00058 00059 /* Read local device SL parameter */ 00060 log_serial->printf("\r\nReading local device SL parameter:\r\n"); 00061 cmdresp = xbee.get_param("SL", &value); 00062 if (cmdresp == AtCmdFrame::AtCmdRespOk) { 00063 log_serial->printf("OK. Local SL=%08x\r\n", value); 00064 00065 /* Get the local device 64 bit address to compare */ 00066 const uint64_t LocalDeviceAddr64 = xbee.get_addr64(); 00067 MBED_ASSERT(value == (LocalDeviceAddr64 & 0xFFFFFFFF)); 00068 } else { 00069 log_serial->printf("FAILED with %d\r\n", (int) cmdresp); 00070 } 00071 00072 /* Set local device NI parameter */ 00073 char ni_local[] = "ni_example_local"; 00074 log_serial->printf("\r\nSetting local device NI parameter to '%s':\r\n", ni_local); 00075 cmdresp = xbee.set_param("NI", (uint8_t*)ni_local, strlen(ni_local)); 00076 if (cmdresp == AtCmdFrame::AtCmdRespOk) { 00077 log_serial->printf("OK\r\n"); 00078 } else { 00079 log_serial->printf("FAILED with %d\r\n", (int) cmdresp); 00080 } 00081 00082 const RemoteXBeeZB remoteDevice = RemoteXBeeZB(REMOTE_NODE_ADDR64); 00083 00084 /* Read remote device SL parameter */ 00085 log_serial->printf("\r\nReading remote device SL parameter:\r\n"); 00086 cmdresp = xbee.get_param(remoteDevice, "SL", &value); 00087 if (cmdresp == AtCmdFrame::AtCmdRespOk) { 00088 log_serial->printf("OK. Remote SL=%08x\r\n", value); 00089 MBED_ASSERT(value == REMOTE_NODE_ADDR64_LSB); 00090 } else { 00091 log_serial->printf("FAILED with %d\r\n", (int) cmdresp); 00092 } 00093 00094 /* Set remote device NI parameter */ 00095 char ni_remote[] = "ni_example_remote"; 00096 log_serial->printf("\r\nSetting remote device NI parameter to '%s':\r\n", ni_remote); 00097 cmdresp = xbee.set_param(remoteDevice, "NI", (uint8_t*)ni_remote, strlen(ni_remote)); 00098 if (cmdresp == AtCmdFrame::AtCmdRespOk) { 00099 log_serial->printf("OK\r\n"); 00100 } else { 00101 log_serial->printf("FAILED with %d\r\n", (int) cmdresp); 00102 } 00103 00104 delete(log_serial); 00105 }
Generated on Tue Jul 12 2022 13:10:58 by
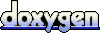