
DigiMesh Send Data example for mbed XBeeLib By Digi
Fork of XBeeZB_Send_Data by
main.cpp
00001 /** 00002 * Copyright (c) 2015 Digi International Inc., 00003 * All rights not expressly granted are reserved. 00004 * 00005 * This Source Code Form is subject to the terms of the Mozilla Public 00006 * License, v. 2.0. If a copy of the MPL was not distributed with this file, 00007 * You can obtain one at http://mozilla.org/MPL/2.0/. 00008 * 00009 * Digi International Inc. 11001 Bren Road East, Minnetonka, MN 55343 00010 * ======================================================================= 00011 */ 00012 00013 #include "mbed.h" 00014 #include "XBeeLib.h" 00015 #if defined(ENABLE_LOGGING) 00016 #include "DigiLoggerMbedSerial.h" 00017 using namespace DigiLog; 00018 #endif 00019 00020 #define REMOTE_NODE_ADDR64_MSB ((uint32_t)0x0013A200) 00021 00022 #error "Replace next define with the LSB of the remote module's 64-bit address (SL parameter)" 00023 #define REMOTE_NODE_ADDR64_LSB ((uint32_t)0x01234567) 00024 00025 #define REMOTE_NODE_ADDR64 UINT64(REMOTE_NODE_ADDR64_MSB, REMOTE_NODE_ADDR64_LSB) 00026 00027 using namespace XBeeLib; 00028 00029 Serial *log_serial; 00030 00031 static void send_broadcast_data(XBeeDM& xbee) 00032 { 00033 const char data[] = "send_broadcast_data"; 00034 const uint16_t data_len = strlen(data); 00035 00036 const TxStatus txStatus = xbee.send_data_broadcast((const uint8_t *)data, data_len); 00037 if (txStatus == TxStatusSuccess) 00038 log_serial->printf("send_broadcast_data OK\r\n"); 00039 else 00040 log_serial->printf("send_broadcast_data failed with %d\r\n", (int) txStatus); 00041 } 00042 00043 static void send_data_to_remote_node(XBeeDM& xbee, const RemoteXBeeDM& RemoteDevice) 00044 { 00045 const char data[] = "send_data_to_remote_node"; 00046 const uint16_t data_len = strlen(data); 00047 00048 const TxStatus txStatus = xbee.send_data(RemoteDevice, (const uint8_t *)data, data_len); 00049 if (txStatus == TxStatusSuccess) 00050 log_serial->printf("send_data_to_remote_node OK\r\n"); 00051 else 00052 log_serial->printf("send_data_to_remote_node failed with %d\r\n", (int) txStatus); 00053 } 00054 00055 static void send_explicit_data_to_remote_node(XBeeDM& xbee, const RemoteXBeeDM& RemoteDevice) 00056 { 00057 char data[] = "send_explicit_data_to_remote_node"; 00058 const uint16_t data_len = strlen(data); 00059 const uint8_t dstEP = 0xE8; 00060 const uint8_t srcEP = 0xE8; 00061 const uint16_t clusterID = 0x0011; 00062 const uint16_t profileID = 0xC105; 00063 00064 const TxStatus txStatus = xbee.send_data(RemoteDevice, dstEP, srcEP, clusterID, profileID, (const uint8_t *)data, data_len); 00065 if (txStatus == TxStatusSuccess) 00066 log_serial->printf("send_explicit_data_to_remote_node OK\r\n"); 00067 else 00068 log_serial->printf("send_explicit_data_to_remote_node failed with %d\r\n", (int) txStatus); 00069 } 00070 00071 int main() 00072 { 00073 log_serial = new Serial(DEBUG_TX, DEBUG_RX); 00074 log_serial->baud(9600); 00075 log_serial->printf("Sample application to demo how to send unicast and broadcast data with the XBeeDM\r\n\r\n"); 00076 log_serial->printf(XB_LIB_BANNER); 00077 00078 #if defined(ENABLE_LOGGING) 00079 new DigiLoggerMbedSerial(log_serial, LogLevelInfo); 00080 #endif 00081 00082 XBeeDM xbee = XBeeDM(RADIO_TX, RADIO_RX, RADIO_RESET, NC, NC, 9600); 00083 00084 RadioStatus radioStatus = xbee.init(); 00085 MBED_ASSERT(radioStatus == Success); 00086 00087 const RemoteXBeeDM remoteDevice = RemoteXBeeDM(REMOTE_NODE_ADDR64); 00088 00089 send_broadcast_data(xbee); 00090 send_data_to_remote_node(xbee, remoteDevice); 00091 send_explicit_data_to_remote_node(xbee, remoteDevice); 00092 00093 delete(log_serial); 00094 }
Generated on Mon Jul 18 2022 04:10:40 by
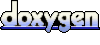