ssss
Embed:
(wiki syntax)
Show/hide line numbers
Audio.cpp
00001 #include "Audio.h" 00002 00003 extern Serial dbg; 00004 00005 double nada[10] = {0, 247, 262, 294, 330, 349, 392, 440, 494, 523}; 00006 //double nadas[16] = { 6, 6, 0, 6, 00007 // 0, 4, 6, 0, 00008 // 8, 0, 0, 0, 00009 // 1, 0, 0, 0 }; 00010 // 00011 unsigned int nadas[8] = { 1, 2, 3, 4, 00012 5, 6, 7, 8 }; 00013 00014 Audio::Audio(PinName buzzer): _out(buzzer) 00015 { 00016 } 00017 00018 void Audio::SetVolume(double volume) 00019 { 00020 vol = volume; 00021 } 00022 00023 void Audio::SetDuration(unsigned int milisec) 00024 { 00025 ms = milisec; 00026 } 00027 00028 void Audio::PlayNote(unsigned int angka) 00029 { 00030 if(angka > 8) angka -= 8; 00031 00032 double Nada = nada[angka]; 00033 PlayNote(Nada*4.24, ms, vol); 00034 } 00035 00036 void Audio::mute() 00037 { 00038 DigitalOut *out = new DigitalOut(_out); 00039 out->write(0); 00040 delete out; 00041 } 00042 00043 void Audio::PlayNote(double frequency, double duration, double volume) 00044 { 00045 PwmOut *out = new PwmOut(_out); 00046 out->period(1.0/frequency); 00047 out->write(volume/2.0); 00048 wait_ms(duration); 00049 out->write(0.0); 00050 delete out; 00051 } 00052 00053 // ----------------------------------------------------------------------------- 00054 MyAudio::MyAudio(PinName buzz) : Audio (buzz) 00055 {} 00056 00057 void MyAudio::play(const int &code) 00058 { 00059 switch(code) 00060 { 00061 case 0 : 00062 mute(); 00063 break; 00064 case 1 : 00065 playing(6); 00066 set(); 00067 playing(1); 00068 set(); 00069 mute(); 00070 break; 00071 case 2 : 00072 00073 // break; 00074 case 3 : 00075 00076 // break; 00077 case 4 : 00078 set(); 00079 playing(1); 00080 break; 00081 case 5 : 00082 set(); 00083 for(int i=0; i<3; i++)playing(4); 00084 break; 00085 default: 00086 set(100, 0.5); 00087 for(int j=0; j<8; j++) 00088 { 00089 playing(nadas[j]); 00090 } 00091 set(); 00092 mute(); 00093 break; 00094 } 00095 } 00096 00097 void MyAudio::set(double dur, double vol) 00098 { 00099 Audio::SetVolume(vol); 00100 Audio::SetDuration(dur); 00101 } 00102 00103 void MyAudio::playing(unsigned int note) 00104 { 00105 Audio::PlayNote(note); 00106 } 00107 00108 void MyAudio::mute() 00109 { 00110 Audio::mute(); 00111 }
Generated on Mon Aug 1 2022 04:18:18 by
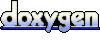