
First commit
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "BLEDevice.h" 00003 #include "FindmeService.h" 00004 #include "BatteryService.h" 00005 #include "DeviceInformationService.h" 00006 #include "DFUService.h" 00007 00008 #define UPDATE_PARAMS_FOR_LONGER_CONNECTION_INTERVAL 0 00009 00010 BLEDevice ble; 00011 DigitalOut led1(p0); 00012 DigitalOut Buz(p1); 00013 00014 void NoAlert(void){ 00015 Buz = 0; 00016 } 00017 void MildAlert(void){ 00018 Buz = !Buz; 00019 wait(2); 00020 } 00021 void HighAlert(void){ 00022 Buz = !Buz; 00023 wait(1); 00024 } 00025 00026 const static char DEVICE_NAME[] = "FindME"; 00027 static const uint16_t uuid16_list[] = {GattService::UUID_IMMEDIATE_ALERT_SERVICE, //Find me! 00028 GattService::UUID_BATTERY_SERVICE, 00029 GattService::UUID_DEVICE_INFORMATION_SERVICE, 00030 DFUServiceShortUUID}; 00031 00032 static volatile bool triggerSensorPolling = false; 00033 00034 void disconnectionCallback(Gap::Handle_t handle, Gap::DisconnectionReason_t reason) 00035 { 00036 ble.startAdvertising(); // restart advertising 00037 } 00038 00039 void onConnectionCallback(Gap::Handle_t handle, Gap::addr_type_t peerAddrType, const Gap::address_t peerAddr, const Gap::ConnectionParams_t *params) 00040 { 00041 #if UPDATE_PARAMS_FOR_LONGER_CONNECTION_INTERVAL 00042 00043 #define MIN_CONN_INTERVAL 250 /**< Minimum connection interval (250 ms) */ 00044 #define MAX_CONN_INTERVAL 350 /**< Maximum connection interval (350 ms). */ 00045 #define CONN_SUP_TIMEOUT 6000 /**< Connection supervisory timeout (6 seconds). */ 00046 #define SLAVE_LATENCY 4 00047 00048 Gap::ConnectionParams_t gap_conn_params; 00049 gap_conn_params.minConnectionInterval = Gap::MSEC_TO_GAP_DURATION_UNITS(MIN_CONN_INTERVAL); 00050 gap_conn_params.maxConnectionInterval = Gap::MSEC_TO_GAP_DURATION_UNITS(MAX_CONN_INTERVAL); 00051 gap_conn_params.connectionSupervisionTimeout = Gap::MSEC_TO_GAP_DURATION_UNITS(CONN_SUP_TIMEOUT); 00052 gap_conn_params.slaveLatency = SLAVE_LATENCY; 00053 ble.updateConnectionParams(handle, &gap_conn_params); 00054 #endif /* #if UPDATE_PARAMS_FOR_LONGER_CONNECTION_INTERVAL */ 00055 } 00056 00057 void periodicCallback(void) 00058 { 00059 led1 = !led1; /* Do blinky on LED1 while we're waiting for BLE events */ 00060 triggerSensorPolling = true; 00061 } 00062 00063 int main(void) 00064 { //NRF_CLOCK->LFCLKSRC = CLOCK_LFCLKSRC_SRC_RC; 00065 //NRF_CLOCK->XTALFREQ = 0x00; 00066 //NRF_CLOCK->EVENTS_HFCLKSTARTED = 0; 00067 //NRF_CLOCK->TASKS_HFCLKSTART = 1; 00068 //while (NRF_CLOCK->EVENTS_HFCLKSTARTED == 0) 00069 //{// Do nothing. 00070 //} 00071 Buz = 0; 00072 led1 = 0; 00073 Ticker ticker; 00074 //Ticker Buzzer; 00075 ticker.attach(periodicCallback, 1); 00076 00077 ble.init(); 00078 ble.onDisconnection(disconnectionCallback); 00079 #if UPDATE_PARAMS_FOR_LONGER_CONNECTION_INTERVAL 00080 ble.onConnection(onConnectionCallback); 00081 #endif /* #if UPDATE_PARAMS_FOR_LONGER_CONNECTION_INTERVAL */ 00082 00083 00084 BatteryService battery(ble); 00085 DeviceInformationService deviceInfo(ble, "Cyntec", "Combo module", "SN1", "hw-rev1", "fw-rev1", "soft-rev1"); 00086 FindMeService fmService(ble); 00087 DFUService DFU(ble); 00088 00089 /* Setup advertising. */ 00090 /* Setting advertising string*/ 00091 ble.accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); 00092 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, (uint8_t *)uuid16_list, sizeof(uuid16_list)); 00093 ble.accumulateAdvertisingPayload(GapAdvertisingData::GENERIC_TAG); 00094 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00095 /* Setting advertising parameters*/ 00096 ble.setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00097 ble.setAdvertisingInterval(Gap::MSEC_TO_ADVERTISEMENT_DURATION_UNITS(1000)); //0x20~0x4000, 0.625ms, 20ms~10.24s 00098 ble.setAdvertisingTimeout(0x1e); //Timeout, stop advertising after 30sec 00099 ble.startAdvertising(); 00100 00101 while (true) { 00102 if (triggerSensorPolling && ble.getGapState().connected) { 00103 triggerSensorPolling = false; 00104 00105 switch(fmService.AlertValue) 00106 { 00107 case 0: 00108 //led1 = 0; 00109 //Buz = 0; 00110 NoAlert(); 00111 break; 00112 case 1: 00113 //led1 = 1; 00114 MildAlert(); 00115 break; 00116 case 2: 00117 //Buz = 1; 00118 HighAlert(); 00119 break; 00120 default: 00121 led1 = 1; 00122 Buz = 1; 00123 } 00124 00125 } else { 00126 ble.waitForEvent(); 00127 } 00128 } 00129 }
Generated on Sat Jul 16 2022 04:20:51 by
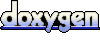