
This CLI (Command Line Interface) is based mbed-os. Both NNN50 and NQ620 are supported.
Fork of NNN40_CLI by
main.cpp
00001 #include <mbed.h> 00002 00003 #include "Gap.h" 00004 #include "command-interpreter.h" 00005 #include "nrf_gpio.h" 00006 00007 #include "ble_cli.h" 00008 00009 #if defined(TARGET_DELTA_DFCM_NNN50) 00010 #error Delta_CLI is not supported on NNN50 temporary due to the memory constraint 00011 #endif 00012 00013 #define uart_buffer_size 64 //Do not increase uart_buffer_size to prevent out of memory, 00014 #define uart_baudrate 38400 //supported baudrate range between 1200 to 230400 (38400) for NQ620 (NNN50) 00015 unsigned char uart_buf[uart_buffer_size]; 00016 unsigned int i = 0; 00017 00018 Serial console(USBTX, USBRX); 00019 00020 void CLI_execute() { 00021 // if (i>=2) 00022 // if (uart_buf[i-2] == 0x0D && uart_buf[i-1] == 0x0A){//detecting CR LF 00023 if (console.readable()) return;//retrun if it is not the end of packet 00024 for (int j=0; j<i; j++) 00025 cyntecProcessCommandInput(uart_buf[j]); 00026 //console.putc(uart_buf[j]);//used for debug only 00027 i=0; 00028 // } 00029 } 00030 void uart_interrupt() { 00031 uart_buf[i++] = console.getc(); 00032 CLI_execute(); 00033 } 00034 00035 int main(void) 00036 { 00037 00038 console.attach(&uart_interrupt); 00039 console.baud(uart_baudrate); 00040 console.printf("\r\nBLE initialized\r\n"); 00041 cynBLEInitCommand(); 00042 }
Generated on Tue Jul 12 2022 21:48:15 by
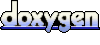