
This CLI (Command Line Interface) is based mbed-os. Both NNN50 and NQ620 are supported.
Fork of NNN40_CLI by
command-interpreter.h
00001 /** @file command-interpreter.h 00002 * @brief Processes commands coming from the serial port. 00003 * See @ref commands for documentation. 00004 * 00005 * Copyright 2014 by DELTA Corporation. All rights reserved. 00006 */ 00007 00008 #ifndef COMMAND_INTERPRETER_H 00009 #define COMMAND_INTERPRETER_H 00010 00011 #include <stdbool.h> 00012 #include <stdint.h> 00013 #include "mbed.h" 00014 //#inlcude "nordic/boards.h" 00015 00016 #define DELTA_BLE_ON 1 00017 #define DELTA_WIFI_ON 0 00018 #define SIMPLE_CMD_NAME 0 00019 00020 #ifndef CYNTEC_COMMAND_BUFFER_LENGTH 00021 #define CYNTEC_COMMAND_BUFFER_LENGTH 255 00022 #endif 00023 00024 #ifndef MAX_TOKEN_COUNT 00025 #define MAX_TOKEN_COUNT 16 00026 #endif 00027 00028 #ifdef __cplusplus 00029 extern "C" { 00030 #endif 00031 00032 enum { 00033 CYNTEC_CMD_SUCCESS, 00034 CYNTEC_CMD_ERR_NO_SUCH_COMMAND, 00035 CYNTEC_CMD_ERR_WRONG_NUMBER_OF_ARGUMENTS, 00036 CYNTEC_CMD_ERR_ARGUMENT_OUT_OF_RANGE, 00037 CYNTEC_CMD_ERR_ARGUMENT_SYNTAX_ERROR, 00038 CYNTEC_CMD_ERR_NO_MATCHED_ARGUMENT, 00039 CYNTEC_CMD_ERR_WRONG_CMD_ORDER, 00040 CYNTEC_CMD_ERR_INVALID_STATE_TO_PERFORM_OPERATION, 00041 CYNTEC_CMD_ERR_CALL_FAIL 00042 }; 00043 00044 typedef void (*CommandAction)(void); 00045 00046 typedef const struct { 00047 /** Use letters, digits, and underscores, '_', for the command name. 00048 * Command names are case-sensitive. 00049 */ 00050 const char *name; 00051 /** A reference to a function in the application that implements the 00052 * command. 00053 * If this entry refers to a nested command, then action field 00054 * has to be set to NULL. 00055 */ 00056 CommandAction action; 00057 /* 00058 * In case of a nested command (action is NULL), then this field 00059 * contains a pointer to the nested CyntecCommandEntry array. 00060 */ 00061 const char *subMenu; 00062 /** A description of the command. 00063 */ 00064 const char *description; 00065 } CyntecCommandEntry; 00066 00067 extern CyntecCommandEntry cyntecCommandTable[]; 00068 00069 uint8_t cyntecAtoi(uint8_t *str, uint8_t len); 00070 int cyntecAtoInt(uint8_t *str); 00071 uint8_t cyntecArgToUint8(uint8_t *str, uint8_t len); 00072 uint16_t cyntecAtoiUint16(uint8_t *str, uint8_t len); 00073 uint16_t cyntecArgToUint16(uint8_t *str, uint8_t len); 00074 uint32_t cyntecHexToUint32(uint8_t *str, uint8_t len); 00075 uint8_t cyntecStrCmp(uint8_t *src, uint8_t *dst, uint8_t len); 00076 00077 /** 00078 * @brief Process the given char as a command. 00079 **/ 00080 void cyntecProcessCommandInput(uint8_t input); 00081 00082 /** @brief Initialize the command interpreter. 00083 */ 00084 void cyntecCommandReaderInit(void); 00085 00086 /** Retrieves unsigned integer arguments. */ 00087 uint8_t *cyntecGetCommandArgument(uint8_t argNum, uint8_t *length); 00088 uint8_t *cyntecGetMinusArgument(uint8_t argNum, uint8_t *length); 00089 extern void clearBuffer(void); 00090 00091 /** Retrieves the token count. */ 00092 uint8_t cyntecGetCommandTokenCnt(void); 00093 00094 //gill add for accept blank in name 20150904 00095 //uint8_t *cyntecGetCommandBuffer(void); 00096 uint8_t *cyntecGetCommandTotalBuffer(void); 00097 void cyntecWriteToTotalBuffer(uint8_t input); 00098 uint8_t cyntecGetTotalIndex(void); 00099 00100 #ifdef __cplusplus 00101 } 00102 #endif 00103 #endif 00104 00105
Generated on Tue Jul 12 2022 21:48:15 by
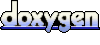