Demo the function of RTC module AM1805
Dependencies: mbed-dev
Fork of I2C_HelloWorld_Mbed by
main.cpp
00001 #include "mbed.h" 00002 #include "AM1805.h" 00003 00004 #define DEMO_INPUT_INTERRUPT 0 00005 #define DEMO_COUNTDOWN_TIMER 0 00006 #define DEMO_ALARM_FUNCTION 1 00007 00008 DigitalOut oneLed(LED1); 00009 DigitalOut twoLed(LED2); 00010 DigitalOut indLed(LED3); 00011 DigitalOut errorLed(LED4); 00012 00013 typedef enum 00014 { 00015 DEFAULT_FLAG = 0xFF, /**< Default State */ 00016 INIT_FLAG = 0x01, /**< Initialize Done */ 00017 HOST_RUN_ONE = 0x02, /**< Run to mode 1 */ 00018 HOST_RUN_TWO = 0x04 /**< Run to mode 2 */ 00019 } demo_mode_t; 00020 00021 int main() { 00022 00023 demo_mode_t demo_status = DEFAULT_FLAG; 00024 00025 /* attempt to read the current status */ 00026 wait(5); 00027 demo_status = (demo_mode_t)am1805_read_ram(0); 00028 00029 if ( demo_status == DEFAULT_FLAG ) 00030 { 00031 /* Not config AM1805 yet */ 00032 indLed = 1; 00033 wait(1); 00034 00035 if ( am1805_init() == false ) 00036 { 00037 while(1) { 00038 wait(0.1); 00039 errorLed = !errorLed; 00040 } 00041 } 00042 00043 #if DEMO_INPUT_INTERRUPT 00044 am1805_config_input_interrupt(XT1_INTERRUPT); 00045 #elif DEMO_COUNTDOWN_TIMER 00046 am1805_config_countdown_timer( PERIOD_SEC, 10, REPEAT_PLUSE_1_64_SEC, PIN_nTIRQ); 00047 #elif DEMO_ALARM_FUNCTION 00048 00049 time_reg_struct_t time_regs; 00050 00051 time_regs.hundredth = 0x00; 00052 time_regs.second = 0x08; 00053 time_regs.minute = 0x00; 00054 time_regs.hour = 0x00; 00055 time_regs.date = 0x00; 00056 time_regs.month = 0x00; 00057 time_regs.year = 0x00; 00058 time_regs.weekday = 0x00; 00059 time_regs.century = 0x00; 00060 time_regs.mode = 0x02; 00061 /* Set specific alarm time */ 00062 am1805_config_alarm(time_regs, ONCE_PER_MINUTE, PULSE_1_4_SEC, PIN_FOUT_nIRQ); 00063 #endif 00064 wait(0.2); 00065 am1805_write_ram(0,HOST_RUN_ONE); 00066 wait(0.2); 00067 am1805_set_sleep(7,1); 00068 } else { 00069 switch(demo_status){ 00070 case HOST_RUN_ONE: 00071 for(uint8_t i = 0;i < 5;i++) 00072 { 00073 wait(1); 00074 oneLed = !oneLed; 00075 } 00076 am1805_write_ram(0,HOST_RUN_TWO); 00077 wait(0.2); 00078 am1805_set_sleep(7,1); 00079 break; 00080 case HOST_RUN_TWO: 00081 for(uint8_t i = 0;i < 5;i++) 00082 { 00083 wait(1); 00084 twoLed = !twoLed; 00085 } 00086 am1805_write_ram(0,HOST_RUN_ONE); 00087 wait(0.2); 00088 am1805_set_sleep(7,1); 00089 break; 00090 default: 00091 errorLed = 1; 00092 break; 00093 } 00094 } 00095 00096 while (1) { 00097 } 00098 }
Generated on Wed Jul 13 2022 11:36:42 by
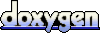