Mouse code for the MacroRat
Embed:
(wiki syntax)
Show/hide line numbers
mbed_assert.h
00001 00002 /** \addtogroup platform */ 00003 /** @{*/ 00004 /* mbed Microcontroller Library 00005 * Copyright (c) 2006-2013 ARM Limited 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); 00008 * you may not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, 00015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 */ 00019 #ifndef MBED_ASSERT_H 00020 #define MBED_ASSERT_H 00021 00022 #include "mbed_preprocessor.h" 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 /** Internal mbed assert function which is invoked when MBED_ASSERT macro failes. 00029 * This function is active only if NDEBUG is not defined prior to including this 00030 * assert header file. 00031 * In case of MBED_ASSERT failing condition, error() is called with the assertation message. 00032 * @param expr Expresion to be checked. 00033 * @param file File where assertation failed. 00034 * @param line Failing assertation line number. 00035 */ 00036 void mbed_assert_internal(const char *expr, const char *file, int line); 00037 00038 #ifdef __cplusplus 00039 } 00040 #endif 00041 00042 #ifdef NDEBUG 00043 #define MBED_ASSERT(expr) ((void)0) 00044 00045 #else 00046 #define MBED_ASSERT(expr) \ 00047 do { \ 00048 if (!(expr)) { \ 00049 mbed_assert_internal(#expr, __FILE__, __LINE__); \ 00050 } \ 00051 } while (0) 00052 #endif 00053 00054 00055 /** MBED_STATIC_ASSERT 00056 * Declare compile-time assertions, results in compile-time error if condition is false 00057 * 00058 * The assertion acts as a declaration that can be placed at file scope, in a 00059 * code block (except after a label), or as a member of a C++ class/struct/union. 00060 * 00061 * @note 00062 * Use of MBED_STATIC_ASSERT as a member of a struct/union is limited: 00063 * - In C++, MBED_STATIC_ASSERT is valid in class/struct/union scope. 00064 * - In C, MBED_STATIC_ASSERT is not valid in struct/union scope, and 00065 * MBED_STRUCT_STATIC_ASSERT is provided as an alternative that is valid 00066 * in C and C++ class/struct/union scope. 00067 * 00068 * @code 00069 * MBED_STATIC_ASSERT(MBED_LIBRARY_VERSION >= 120, 00070 * "The mbed library must be at least version 120"); 00071 * 00072 * int main() { 00073 * MBED_STATIC_ASSERT(sizeof(int) >= sizeof(char), 00074 * "An int must be larger than a char"); 00075 * } 00076 * @endcode 00077 */ 00078 #if defined(__cplusplus) && (__cplusplus >= 201103L || __cpp_static_assert >= 200410L) 00079 #define MBED_STATIC_ASSERT(expr, msg) static_assert(expr, msg) 00080 #elif !defined(__cplusplus) && __STDC_VERSION__ >= 201112L 00081 #define MBED_STATIC_ASSERT(expr, msg) _Static_assert(expr, msg) 00082 #elif defined(__cplusplus) && defined(__GNUC__) && defined(__GXX_EXPERIMENTAL_CXX0X__) \ 00083 && (__GNUC__*100 + __GNUC_MINOR__) > 403L 00084 #define MBED_STATIC_ASSERT(expr, msg) __extension__ static_assert(expr, msg) 00085 #elif !defined(__cplusplus) && defined(__GNUC__) && !defined(__CC_ARM) \ 00086 && (__GNUC__*100 + __GNUC_MINOR__) > 406L 00087 #define MBED_STATIC_ASSERT(expr, msg) __extension__ _Static_assert(expr, msg) 00088 #elif defined(__ICCARM__) 00089 #define MBED_STATIC_ASSERT(expr, msg) static_assert(expr, msg) 00090 #else 00091 #define MBED_STATIC_ASSERT(expr, msg) \ 00092 enum {MBED_CONCAT(MBED_ASSERTION_AT_, __LINE__) = sizeof(char[(expr) ? 1 : -1])} 00093 #endif 00094 00095 /** MBED_STRUCT_STATIC_ASSERT 00096 * Declare compile-time assertions, results in compile-time error if condition is false 00097 * 00098 * Unlike MBED_STATIC_ASSERT, MBED_STRUCT_STATIC_ASSERT can and must be used 00099 * as a member of a C/C++ class/struct/union. 00100 * 00101 * @code 00102 * struct thing { 00103 * MBED_STATIC_ASSERT(2 + 2 == 4, 00104 * "Hopefully the universe is mathematically consistent"); 00105 * }; 00106 * @endcode 00107 */ 00108 #define MBED_STRUCT_STATIC_ASSERT(expr, msg) int : (expr) ? 0 : -1 00109 00110 00111 #endif 00112 00113 /** @}*/
Generated on Tue Jul 12 2022 17:41:24 by
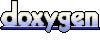