Mouse code for the MacroRat
Embed:
(wiki syntax)
Show/hide line numbers
DigitalOut.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_DIGITALOUT_H 00017 #define MBED_DIGITALOUT_H 00018 00019 #include "platform/platform.h" 00020 #include "hal/gpio_api.h" 00021 #include "platform/mbed_critical.h" 00022 00023 namespace mbed { 00024 /** \addtogroup drivers */ 00025 /** @{*/ 00026 00027 /** A digital output, used for setting the state of a pin 00028 * 00029 * @Note Synchronization level: Interrupt safe 00030 * 00031 * Example: 00032 * @code 00033 * // Toggle a LED 00034 * #include "mbed.h" 00035 * 00036 * DigitalOut led(LED1); 00037 * 00038 * int main() { 00039 * while(1) { 00040 * led = !led; 00041 * wait(0.2); 00042 * } 00043 * } 00044 * @endcode 00045 */ 00046 class DigitalOut { 00047 00048 public: 00049 /** Create a DigitalOut connected to the specified pin 00050 * 00051 * @param pin DigitalOut pin to connect to 00052 */ 00053 DigitalOut(PinName pin) : gpio() { 00054 // No lock needed in the constructor 00055 gpio_init_out(&gpio, pin); 00056 } 00057 00058 /** Create a DigitalOut connected to the specified pin 00059 * 00060 * @param pin DigitalOut pin to connect to 00061 * @param value the initial pin value 00062 */ 00063 DigitalOut(PinName pin, int value) : gpio() { 00064 // No lock needed in the constructor 00065 gpio_init_out_ex(&gpio, pin, value); 00066 } 00067 00068 /** Set the output, specified as 0 or 1 (int) 00069 * 00070 * @param value An integer specifying the pin output value, 00071 * 0 for logical 0, 1 (or any other non-zero value) for logical 1 00072 */ 00073 void write(int value) { 00074 // Thread safe / atomic HAL call 00075 gpio_write(&gpio, value); 00076 } 00077 00078 /** Return the output setting, represented as 0 or 1 (int) 00079 * 00080 * @returns 00081 * an integer representing the output setting of the pin, 00082 * 0 for logical 0, 1 for logical 1 00083 */ 00084 int read() { 00085 // Thread safe / atomic HAL call 00086 return gpio_read(&gpio); 00087 } 00088 00089 /** Return the output setting, represented as 0 or 1 (int) 00090 * 00091 * @returns 00092 * Non zero value if pin is connected to uc GPIO 00093 * 0 if gpio object was initialized with NC 00094 */ 00095 int is_connected() { 00096 // Thread safe / atomic HAL call 00097 return gpio_is_connected(&gpio); 00098 } 00099 00100 /** A shorthand for write() 00101 */ 00102 DigitalOut& operator= (int value) { 00103 // Underlying write is thread safe 00104 write(value); 00105 return *this; 00106 } 00107 00108 DigitalOut& operator= (DigitalOut& rhs) { 00109 core_util_critical_section_enter(); 00110 write(rhs.read()); 00111 core_util_critical_section_exit(); 00112 return *this; 00113 } 00114 00115 /** A shorthand for read() 00116 */ 00117 operator int() { 00118 // Underlying call is thread safe 00119 return read(); 00120 } 00121 00122 protected: 00123 gpio_t gpio; 00124 }; 00125 00126 } // namespace mbed 00127 00128 #endif 00129 00130 /** @}*/
Generated on Tue Jul 12 2022 17:41:24 by
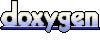