tes sim 5360
Embed:
(wiki syntax)
Show/hide line numbers
SIM5360.h
00001 #ifndef _GSM_H_ 00002 #define _GSM_H_ 00003 00004 #include <stdio.h> 00005 #include "mbed.h" 00006 00007 #define DEFAULT_TIMEOUT 5 00008 #define SMS_MAX_LENGTH 16 00009 00010 00011 enum GSM_MESSAGE { 00012 MESSAGE_RING = 0, 00013 MESSAGE_SMS = 1, 00014 MESSAGE_ERROR 00015 }; 00016 00017 00018 /** GSM class. 00019 * Used for mobile communication. attention that GSM module communicate with MCU in serial protocol 00020 */ 00021 class GSM 00022 { 00023 public: 00024 /** Create GSM instance 00025 * @param tx uart transmit pin to communicate with GSM module 00026 * @param rx uart receive pin to communicate with GSM module 00027 * @param baudRate baud rate of uart communication 00028 * @param number default phone number during mobile communication 00029 */ 00030 GSM(PinName tx, PinName rx, int baudRate,char *number) : gprsSerial(tx, rx) { 00031 //gprsSerial.baud(baudRate); 00032 phoneNumber = number; 00033 }; 00034 00035 int powerCheck(void); 00036 00037 /** init GSM module including SIM card check & signal strength & network check 00038 * @returns 00039 * 0 on success, 00040 * -1 on error 00041 */ 00042 int init(void); 00043 00044 /** Register Network of GSM module including signal strength & network check 00045 * @returns 00046 * 0 on success, 00047 * -1 on error 00048 */ 00049 int registerNet(void); 00050 00051 /** Check network status of GSM module 00052 * @returns 00053 * 0 on success, 00054 * -1 on error 00055 */ 00056 int checkNetStatus(void); 00057 00058 /** Check SIM card' Status 00059 * @returns 00060 * 0 on success, 00061 * -1 on error 00062 */ 00063 int checkSIMStatus(void); 00064 00065 /** Check signal strength 00066 * @returns 00067 * signal strength in number(ex 3,4,5,6,7,8...) on success, 00068 * -1 on error 00069 */ 00070 int checkSignalStrength(void); 00071 00072 /** Set SMS format and processing mode 00073 * @returns 00074 * 0 on success, 00075 * -1 on error 00076 */ 00077 int settingSMS(void); 00078 00079 /** Send text SMS 00080 * @param *number phone number which SMS will be send to 00081 * @param *data message that will be send to 00082 * @returns 00083 * 0 on success, 00084 * -1 on error 00085 */ 00086 int sendSMS(char *number, char *data); 00087 00088 /** Read SMS by index 00089 * @param *message buffer used to get SMS message 00090 * @param index which SMS message to read 00091 * @returns 00092 * 0 on success, 00093 * -1 on error 00094 */ 00095 int readSMS(char *message, int index); 00096 00097 /** Delete SMS message on SIM card 00098 * @param *index the index number which SMS message will be delete 00099 * @returns 00100 * 0 on success, 00101 * -1 on error 00102 */ 00103 int deleteSMS(int index); 00104 00105 /** Read SMS when coming a message,it will be store in messageBuffer. 00106 * @param message buffer used to get SMS message 00107 */ 00108 int getSMS(char* message); 00109 00110 /** Call someone 00111 * @param *number the phone number which you want to call 00112 * @returns 00113 * 0 on success, 00114 * -1 on error 00115 */ 00116 int callUp(char *number); 00117 00118 /** Auto answer if coming a call 00119 * @returns 00120 * 0 on success, 00121 * -1 on error 00122 */ 00123 int answer(void); 00124 00125 /** Join GSM network 00126 * @param *apn Access Point Name to connect network 00127 * @param *userName general is empty 00128 * @param *passWord general is empty 00129 */ 00130 int join(char* apn, char* userName = NULL, char* passWord = NULL); 00131 00132 /** Disconnect from network 00133 * @returns 00134 * 0 on success, 00135 * -1 on error 00136 */ 00137 int disconnect(void); 00138 00139 /** Set blocking of the connection 00140 * @param netopen_to time out of open the socket network in second 00141 * @param cipopen_to time out of open the connection to server in second 00142 * @param cipsend_to time out of send data to server in second 00143 * @returns 00144 * 0 on success, 00145 * -1 on error 00146 */ 00147 int SetBlocking(int netopen_to=5, int cipopen_to=5, int cipsend_to=5); 00148 00149 /** Build TCP connect 00150 * @param *ip ip address which will connect to 00151 * @param *port TCP server' port number 00152 * @returns 00153 * 0 on success, 00154 * -1 on error 00155 */ 00156 int connectTCP(char *ip, int port); 00157 00158 /** Send data to TCP server 00159 * @param *data data that will be send to TCP server 00160 * @returns 00161 * 0 on success, 00162 * -1 on error 00163 */ 00164 int sendTCPData(char *data, int len); 00165 00166 /** Send data to TCP server 00167 * @param *buff data that will be received from TCP server 00168 * @param len size of buffer to read 00169 * @returns 00170 * 0 on success, 00171 * -1 on error 00172 */ 00173 int receivedTCPData(char *buff, int len); 00174 00175 /** Close TCP connection 00176 * @returns 00177 * 0 on success, 00178 * -1 on error 00179 */ 00180 int closeTCP(void); 00181 00182 /** Clear serial pipe 00183 */ 00184 void purge(void); 00185 00186 Serial gprsSerial; 00187 //USBSerial pc; 00188 00189 private: 00190 /** Read from GSM module and save to buffer array 00191 * @param *buffer buffer array to save what read from GSM module 00192 * @param *count the maximal bytes number read from GSM module 00193 * @returns 00194 * 0 on success, 00195 * -1 on error 00196 */ 00197 int readBuffer(char *buffer,int count); 00198 00199 /** Send AT command to GSM module 00200 * @param *cmd command array which will be send to GSM module 00201 */ 00202 void sendCmd(char *cmd); 00203 00204 /** Check GSM module response before timeout 00205 * @param *resp correct response which GSM module will return 00206 * @param *timeout waiting seconds till timeout 00207 * @returns 00208 * 0 on success, 00209 * -1 on error 00210 */ 00211 int waitForResp(char *resp, int timeout); 00212 00213 /** Send AT command to GSM module and wait for correct response 00214 * @param *cmd AT command which will be send to GSM module 00215 * @param *resp correct response which GSM module will return 00216 * @param *timeout waiting seconds till timeout 00217 * @returns 00218 * 0 on success, 00219 * -1 on error 00220 */ 00221 int sendCmdAndWaitForResp(char *cmd, char *resp, int timeout); 00222 00223 Timer timeCnt; 00224 char *phoneNumber; 00225 char messageBuffer[SMS_MAX_LENGTH]; 00226 }; 00227 00228 #endif // _GSM_H_ 00229
Generated on Wed Jul 13 2022 00:17:58 by
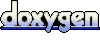