tes sim 5360
Embed:
(wiki syntax)
Show/hide line numbers
SIM5360.cpp
00001 #include "SIM5360.h" 00002 00003 int GSM::readBuffer(char *buffer,int count) 00004 { 00005 int i = 0; 00006 timeCnt.start(); // start timer 00007 while(1) { 00008 while (gprsSerial.readable()) { 00009 char c = gprsSerial.getc(); 00010 if (c == '\r' || c == '\n') c = '$'; 00011 buffer[i++] = c; 00012 if(i > count)break; 00013 } 00014 if(i > count)break; 00015 if(timeCnt.read() > DEFAULT_TIMEOUT) { 00016 timeCnt.stop(); 00017 timeCnt.reset(); 00018 break; 00019 } 00020 } 00021 wait(0.5); 00022 while(gprsSerial.readable()) { // display the other thing.. 00023 char c = gprsSerial.getc(); 00024 } 00025 return 0; 00026 } 00027 00028 void cleanBuffer(char *buffer, int count) 00029 { 00030 for(int i=0; i < count; i++) { 00031 buffer[i] = '\0'; 00032 } 00033 } 00034 00035 void GSM::purge(void) 00036 { 00037 while(gprsSerial.readable()) 00038 { 00039 gprsSerial.getc(); 00040 } 00041 } 00042 00043 void GSM::sendCmd(char *cmd) 00044 { 00045 gprsSerial.puts(cmd); 00046 } 00047 00048 int GSM::waitForResp(char *resp, int timeout) 00049 { 00050 int len = strlen(resp); 00051 int sum=0; 00052 timeCnt.start(); 00053 00054 while(1) { 00055 if(gprsSerial.readable()) { 00056 char c = gprsSerial.getc(); 00057 sum = (c==resp[sum]) ? sum+1 : 0; 00058 if(sum == len)break; 00059 } 00060 if(timeCnt.read() > timeout) { // time out 00061 timeCnt.stop(); 00062 timeCnt.reset(); 00063 return -1; 00064 } 00065 } 00066 timeCnt.stop(); // stop timer 00067 timeCnt.reset(); // clear timer 00068 while(gprsSerial.readable()) { // display the other thing.. 00069 char c = gprsSerial.getc(); 00070 } 00071 00072 return 0; 00073 } 00074 00075 int GSM::sendCmdAndWaitForResp(char *cmd, char *resp, int timeout) 00076 { 00077 sendCmd(cmd); 00078 return waitForResp(resp,timeout); 00079 } 00080 00081 int GSM::powerCheck(void) 00082 { 00083 return sendCmdAndWaitForResp("AT\r\n", "OK", 2); 00084 } 00085 00086 int GSM::init(void) 00087 { 00088 int i=10; 00089 00090 while (i--) { 00091 // purge any messages 00092 purge(); 00093 00094 // check interface 00095 int r = sendCmdAndWaitForResp("AT\r\n", "OK", DEFAULT_TIMEOUT); 00096 wait_ms(100); 00097 if(r == 0) break; 00098 } 00099 if (i < 0) { 00100 goto failure; 00101 } 00102 00103 if(0 != sendCmdAndWaitForResp("ATE0\r\n", "OK", DEFAULT_TIMEOUT)) 00104 goto failure; 00105 00106 if(0 != sendCmdAndWaitForResp("AT+CMEE=2\r\n", "OK", DEFAULT_TIMEOUT)) 00107 goto failure; 00108 00109 if(0 != checkSIMStatus()) { 00110 goto failure; 00111 } 00112 00113 if(0 != sendCmdAndWaitForResp("AT+CGREG=1\r\n", "OK", DEFAULT_TIMEOUT)){ 00114 goto failure; 00115 } 00116 00117 if(0 != sendCmdAndWaitForResp("AT+CREG=1\r\n", "OK", DEFAULT_TIMEOUT)){ 00118 goto failure; 00119 } 00120 00121 if(0 != settingSMS()){ 00122 goto failure; 00123 } 00124 00125 return 0; 00126 00127 failure: 00128 return -1; 00129 } 00130 00131 int GSM::registerNet(void) 00132 { 00133 int ret = -1; 00134 int i = 10; 00135 while(i--){ 00136 int r = checkNetStatus(); 00137 if(r == 0){ 00138 ret = 0; 00139 break; 00140 } 00141 wait_ms(1000); 00142 } 00143 00144 if(checkSignalStrength()<1) { 00145 goto failure; 00146 } 00147 00148 failure: 00149 return ret; 00150 } 00151 00152 int GSM::checkNetStatus(void) 00153 { 00154 int netReg = -1; 00155 int gprsReg = -1; 00156 char gprsBuffer[30]; 00157 cleanBuffer(gprsBuffer,30); 00158 00159 sendCmd("AT+CREG?\r\n"); 00160 readBuffer(gprsBuffer,30); 00161 if((NULL != strstr(gprsBuffer,"+CREG: 0,1"))) { 00162 netReg = 0; 00163 } 00164 00165 wait_ms(100); 00166 sendCmd("AT+CREG?\r\n"); 00167 readBuffer(gprsBuffer,30); 00168 if((NULL != strstr(gprsBuffer,"+CGREG: 0,1"))) { 00169 gprsReg = 0; 00170 } 00171 00172 return netReg | gprsReg; 00173 } 00174 00175 int GSM::checkSIMStatus(void) 00176 { 00177 char gprsBuffer[30]; 00178 int count = 0; 00179 cleanBuffer(gprsBuffer,30); 00180 while(count < 3) { 00181 sendCmd("AT+CPIN?\r\n"); 00182 readBuffer(gprsBuffer,30); 00183 if((NULL != strstr(gprsBuffer,"+CPIN: READY"))) { 00184 break; 00185 } 00186 count++; 00187 wait(1); 00188 } 00189 00190 if(count == 3) { 00191 return -1; 00192 } 00193 return 0; 00194 } 00195 00196 int GSM::checkSignalStrength(void) 00197 { 00198 char gprsBuffer[100]; 00199 int index,count = 0; 00200 cleanBuffer(gprsBuffer,100); 00201 while(count < 3) { 00202 sendCmd("AT+CSQ\r\n"); 00203 readBuffer(gprsBuffer,25); 00204 if(sscanf(gprsBuffer, "AT+CSQ$$$$+CSQ: %d", &index)>0) { 00205 break; 00206 } 00207 count++; 00208 wait(1); 00209 } 00210 if(count == 3) { 00211 return -1; 00212 } 00213 return index; 00214 } 00215 00216 int GSM::settingSMS(void) 00217 { 00218 if(0 != sendCmdAndWaitForResp("AT+CNMI=2,2\r\n", "OK", DEFAULT_TIMEOUT)) { 00219 return -1; 00220 } 00221 if(0 != sendCmdAndWaitForResp("AT+CMGF=1\r\n", "OK", DEFAULT_TIMEOUT)) { 00222 return -1; 00223 } 00224 return 0; 00225 } 00226 00227 int GSM::sendSMS(char *number, char *data) 00228 { 00229 char cmd[64]; 00230 while(gprsSerial.readable()) { 00231 char c = gprsSerial.getc(); 00232 } 00233 snprintf(cmd, sizeof(cmd),"AT+CMGS=\"%s\"\r\n",number); 00234 if(0 != sendCmdAndWaitForResp(cmd,">",DEFAULT_TIMEOUT)) { 00235 return -1; 00236 } 00237 wait(1); 00238 gprsSerial.puts(data); 00239 gprsSerial.putc((char)0x1a); 00240 return 0; 00241 } 00242 00243 int GSM::readSMS(char *message, int index) 00244 { 00245 int i = 0; 00246 char gprsBuffer[100]; 00247 char *p,*s; 00248 gprsSerial.printf("AT+CMGR=%d\r\n",index); 00249 cleanBuffer(gprsBuffer,100); 00250 readBuffer(gprsBuffer,100); 00251 if(NULL == ( s = strstr(gprsBuffer,"+CMGR"))) { 00252 return -1; 00253 } 00254 if(NULL != ( s = strstr(gprsBuffer,"+32"))) { 00255 p = s + 6; 00256 while((*p != '$')&&(i < SMS_MAX_LENGTH-1)) { 00257 message[i++] = *(p++); 00258 } 00259 message[i] = '\0'; 00260 } 00261 return 0; 00262 } 00263 00264 int GSM::deleteSMS(int index) 00265 { 00266 char cmd[32]; 00267 snprintf(cmd,sizeof(cmd),"AT+CMGD=%d\r\n",index); 00268 sendCmd(cmd); 00269 return 0; 00270 } 00271 00272 int GSM::getSMS(char* message) 00273 { 00274 if(NULL != messageBuffer) { 00275 strncpy(message,messageBuffer,SMS_MAX_LENGTH); 00276 } 00277 return 0; 00278 } 00279 00280 int GSM::callUp(char *number) 00281 { 00282 if(0 != sendCmdAndWaitForResp("AT+COLP=1\r\n","OK",5)) { 00283 return -1; 00284 } 00285 wait(1); 00286 gprsSerial.printf("\r\nATD%s;\r\n",NULL==number?phoneNumber:number); 00287 return 0; 00288 } 00289 00290 int GSM::answer(void) 00291 { 00292 gprsSerial.printf("ATA\r\n"); 00293 return 0; 00294 } 00295 00296 int GSM::join(char* apn, char* userName, char* passWord) 00297 { 00298 char gprsBuffer[64]; 00299 int ret = 0; 00300 00301 cleanBuffer(gprsBuffer,64); 00302 snprintf(gprsBuffer,sizeof(gprsBuffer),"AT+CGSOCKCONT=1,\"IP\",\"%s\"\r\n", apn); 00303 if(0 != sendCmdAndWaitForResp(gprsBuffer, "OK", DEFAULT_TIMEOUT)) { 00304 ret = -1; 00305 goto failure; 00306 } 00307 00308 cleanBuffer(gprsBuffer,64); 00309 snprintf(gprsBuffer,sizeof(gprsBuffer),"AT+CSOCKAUTH=1,1,\"%s\",\"%s\"\r\n", passWord, userName); 00310 if(0 != sendCmdAndWaitForResp(gprsBuffer, "OK", DEFAULT_TIMEOUT)) { 00311 ret = -1; 00312 goto failure; 00313 } 00314 00315 if(0 != sendCmdAndWaitForResp("AT+CSOCKSETPN=1\r\n", "OK", DEFAULT_TIMEOUT)) { 00316 ret = -1; 00317 goto failure; 00318 } 00319 00320 if(0 != sendCmdAndWaitForResp("AT+CIPMODE=0\r\n", "OK", DEFAULT_TIMEOUT)) { 00321 ret = -1; 00322 goto failure; 00323 } 00324 00325 if(0 != sendCmdAndWaitForResp("AT+NETOPEN\r\n", "OK", DEFAULT_TIMEOUT)) { 00326 ret = -1; 00327 goto failure; 00328 } 00329 00330 failure: 00331 return ret; 00332 00333 } 00334 00335 int GSM::SetBlocking(int netopen_to, int cipopen_to, int cipsend_to) 00336 { 00337 int ret = 0; 00338 char gprsBuffer[64]; 00339 00340 cleanBuffer(gprsBuffer,64); 00341 snprintf(gprsBuffer,sizeof(gprsBuffer),"AT+CIPTIMEOUT=%d,%d,%d\r\n", netopen_to, cipopen_to, cipsend_to); 00342 if(0 != sendCmdAndWaitForResp(gprsBuffer, "OK", DEFAULT_TIMEOUT)) { 00343 ret = -1; 00344 goto failure; 00345 } 00346 00347 failure: 00348 return ret; 00349 } 00350 00351 int GSM::connectTCP(char *ip, int port) 00352 { 00353 int ret = 0; 00354 char cipstart[64]; 00355 #if 0 00356 if(0 != sendCmdAndWaitForResp("AT+CIPOPEN=0,\"TCP\",\"\",\r\n", "OK", 5)) { 00357 ret = -1; 00358 goto failure; 00359 } 00360 #endif 00361 sprintf(cipstart, "AT+CIPOPEN=0,\"TCP\",\"%s\",%d\r\n", ip, port); 00362 if(0 != sendCmdAndWaitForResp(cipstart, "OK", DEFAULT_TIMEOUT)) { 00363 ret = -1; 00364 goto failure; 00365 } 00366 00367 purge(); 00368 00369 failure: 00370 return ret; 00371 } 00372 00373 int GSM::sendTCPData(char *data, int len) 00374 { 00375 int ret = 0; 00376 char cmd[64]; 00377 00378 snprintf(cmd,sizeof(cmd),"AT+CIPSEND=0,%d\r\n",len); 00379 if(0 != sendCmdAndWaitForResp(cmd,">",DEFAULT_TIMEOUT)) { 00380 ret = -1; 00381 goto failure; 00382 } 00383 00384 if(0 != sendCmdAndWaitForResp(data,"OK",DEFAULT_TIMEOUT)) { 00385 ret = -1; 00386 goto failure; 00387 } 00388 00389 failure: 00390 return ret; 00391 } 00392 00393 int GSM::receivedTCPData(char *buff, int len) 00394 { 00395 readBuffer(buff, len); 00396 00397 if(strlen(buff) <= 0) return -1; 00398 00399 return 0; 00400 } 00401 00402 int GSM::closeTCP(void) 00403 { 00404 if(0 != sendCmdAndWaitForResp("AT+CIPCLOSE\r\n","OK",DEFAULT_TIMEOUT)) { 00405 return -1; 00406 } 00407 return 0; 00408 } 00409 00410 int GSM::disconnect(void) 00411 { 00412 if(0 != sendCmdAndWaitForResp("AT+NETCLOSE\r\n","OK",DEFAULT_TIMEOUT)) { 00413 return -1; 00414 } 00415 return 0; 00416 }
Generated on Wed Jul 13 2022 00:17:58 by
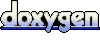