uses pushing box to publish to google spreadsheets with a state machine instead of a while loop
Fork of GSM_Library by
gsmqueue.h
00001 #ifndef GSMQUEUE_H 00002 #define GSMQUEUE_H 00003 00004 /* gsmqueue.h 00005 * Contains functions to read from the DMA buffer in a queue fashion 00006 */ 00007 00008 //Memory block of char size alocated for DMA 00009 #define BUFFER_LENGTH 2000 00010 #define MAX_SMS_LENGTH 700 //Absolute SMS length max = 900 (let's do 700 to be safe) 00011 #define QUEUETAIL (char*)DMA_TCD0_DADDR 00012 00013 00014 //Public functions ------------------------------------------------------------------------------ 00015 //Initialize variables 00016 void queueInit(); 00017 00018 //Send gsm a command 00019 void sendCommand(char* sPtr); 00020 00021 //Return 1 if queue has data and the GSM is done transmitting its response 00022 bool queueHasResponse(); 00023 00024 //Find an occurrence of the given string in the buffer. 00025 //If advanceQueueHead is true, advance queueHead just until a matching string is found. 00026 bool findInQueue(char* str, bool advanceQueueHead); 00027 00028 //Parse through characters until first integer is found 00029 int parseInt(); 00030 00031 //Print queue elements 00032 void printQueue(); //for debugging 00033 00034 00035 //Internal functions ------------------------------------------------------------------------------ 00036 //Get the GSM DMA idle bit (if 1, indicates we aren't in the process of receiving a response) 00037 bool getGSMIdleBit(); 00038 00039 //Returns true if the character is numeric 00040 bool isNumeric(char* qPos); 00041 00042 //Increment queue position by 1 (Note: this function is only used by gsmqueue.cpp) 00043 char* incrementIndex(char* pointerToIncrement); 00044 00045 //Increment queue position by n (Note: this function is only used by gsmqueue.cpp) 00046 char* incrementIndex(char* pointerToIncrement, int n); 00047 00048 //Get size of the queue from reference point of tail parameter 00049 int queueSize(char* tail); 00050 00051 //Clear queue (Note: this function is only used by gsmqueue.cpp) 00052 void flushQueue(); 00053 00054 #endif
Generated on Tue Jul 26 2022 21:19:54 by
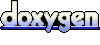