
uses pushing box to write to google spreadsheets
Dependencies: GSM_PUSHING_BOX_STATE_MACHINE MBed_Adafruit-GPS-Library SDFileSystem mbed
Fork of DCS by
modem.cpp
00001 /* 00002 modem.cpp 00003 2014 Copyright (c) Seeed Technology Inc. All right reserved. 00004 00005 Author:lawliet zou(lawliet.zou@gmail.com) 00006 2014-2-24 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00021 */ 00022 00023 #include "modem.h" 00024 //Serial pc(USBTX,USBRX); 00025 extern SDFileSystem sd; //MOSI, MISO, SCLK, SSEL. Tested on K64F, correct pins. 00026 AnalogIn LM35(PTB2); 00027 // added by Noah Milam 00028 void Modem::gprs_response() 00029 { 00030 printf("\nlistening for client\n"); 00031 char buffer[100]; 00032 int count = 0; 00033 00034 mkdir("/sd/mydir", 0777); // makes directory if needed 00035 FILE *fp = fopen("/sd/mydir/chemData.csv", "w"); // creats new file to write 00036 fprintf(fp,"phone Number,chem data, latitude,longitude\n"); // writes in a header for the table 00037 fclose(fp); // closes file 00038 00039 while(1) 00040 { if(serialModem.readable()) { 00041 00042 while(serialModem.readable()) { 00043 char c = serialModem.getc(); 00044 buffer[count++] = c; 00045 //pc.puts(&c); 00046 if(count == 64) break; 00047 } 00048 00049 if(buffer[0] != '\0'){ 00050 buffer[count] = '\0'; 00051 FILE *fp = fopen("/sd/mydir/chemicalData.csv", "a"); // opens file to append it 00052 fprintf(fp,"%s\n",buffer);//writes to file 00053 fclose(fp); // closes file 00054 00055 printf("%s \n",buffer); 00056 for(int i = 0; i < count+2; i++) { 00057 buffer[i] = NULL; 00058 } 00059 } 00060 count = 0; 00061 } 00062 } 00063 } 00064 00065 void Modem::wait_for_sms(){ 00066 printf("waiting for message\n"); 00067 while(1){ 00068 if(serialModem.readable()){ 00069 return; 00070 } 00071 } 00072 } 00073 void Modem::get_message(){ 00074 char tempStr[30]; 00075 int count= 0; 00076 int line_count = 0; 00077 for(int i= 0;i < strlen(IPAdd);i++){ 00078 if(line_count == 3){ 00079 tempStr[count++] = IPAdd[i]; 00080 } 00081 if(IPAdd[i] == '\n'){ 00082 line_count++; 00083 } 00084 } 00085 tempStr[count - 2] = '\0'; 00086 count++; 00087 strncpy(IPAdd, tempStr, count); 00088 printf("IP Addr > %s \n", IPAdd); 00089 printf("text size > %d\n",strlen(IPAdd)); 00090 } 00091 void Modem::storeResp(){ 00092 char buffer[100]; 00093 int count = 0; 00094 timeCnt.start(); 00095 while(timeCnt.read() < 5) 00096 { 00097 while(serialModem.readable()) { 00098 char c = serialModem.getc(); 00099 buffer[count++] = c; 00100 } 00101 } 00102 timeCnt.stop(); 00103 timeCnt.reset(); 00104 buffer[count] = '\0'; 00105 00106 strncpy(IPAdd, buffer, count); 00107 printf("original>> %s",IPAdd); 00108 printf("size of text > %d",strlen(IPAdd)); 00109 count = 0; 00110 get_message(); 00111 } 00112 00113 char* Modem::get_server_IP(){ 00114 return IPAdd; 00115 } 00116 //end added by Noah Milam 00117 char Modem::readByte(void) 00118 { 00119 return serialModem.getc(); 00120 } 00121 00122 bool Modem::readable() 00123 { 00124 return serialModem.readable(); 00125 } 00126 00127 int Modem::readBuffer(char *buffer,int count, unsigned int timeOut) 00128 { 00129 int i = 0; 00130 timeCnt.start(); 00131 while(1) { 00132 while (serialModem.readable()) { 00133 char c = serialModem.getc(); 00134 buffer[i++] = c; 00135 if(i >= count)break; 00136 } 00137 if(i >= count)break; 00138 if(timeCnt.read() > timeOut) { 00139 timeCnt.stop(); 00140 timeCnt.reset(); 00141 break; 00142 } 00143 } 00144 return 0; 00145 } 00146 00147 void Modem::cleanBuffer(char *buffer, int count) 00148 { 00149 for(int i=0; i < count; i++) { 00150 buffer[i] = '\0'; 00151 } 00152 } 00153 00154 void Modem::sendCmd(const char* cmd) 00155 { 00156 serialModem.puts(cmd); 00157 } 00158 void Modem::sendCmdResp(const char* cmd) 00159 { 00160 serialModem.puts(cmd); 00161 getResp(); 00162 } 00163 void Modem::getResp() 00164 { 00165 char buffer[1000]; 00166 int count = 0; 00167 timeCnt.start(); 00168 while(timeCnt.read() < 5) 00169 { while(serialModem.readable()) { 00170 char c = serialModem.getc(); 00171 buffer[count++] = c; 00172 } 00173 } 00174 timeCnt.stop(); 00175 timeCnt.reset(); 00176 buffer[count] = '\0'; 00177 printf("Response:--%s--\r\n",buffer); 00178 for(int i = 0; i < count+2; i++) { 00179 buffer[i] = NULL; 00180 } 00181 count = 0; 00182 } 00183 00184 00185 void Modem::sendATTest(void) 00186 { 00187 sendCmdAndWaitForResp("AT\r\n","OK",DEFAULT_TIMEOUT,CMD); 00188 } 00189 00190 bool Modem::respCmp(const char *resp, unsigned int len, unsigned int timeout) 00191 { 00192 int sum=0; 00193 timeCnt.start(); 00194 00195 while(1) { 00196 if(serialModem.readable()) { 00197 char c = serialModem.getc(); 00198 sum = (c==resp[sum]) ? sum+1 : 0; 00199 if(sum == len)break; 00200 } 00201 if(timeCnt.read() > timeout) { 00202 timeCnt.stop(); 00203 timeCnt.reset(); 00204 return false; 00205 } 00206 } 00207 timeCnt.stop(); 00208 timeCnt.reset(); 00209 00210 return true; 00211 } 00212 00213 int Modem::waitForResp(const char *resp, unsigned int timeout,DataType type) 00214 { 00215 int len = strlen(resp); 00216 int sum=0; 00217 timeCnt.start(); 00218 00219 while(1) { 00220 if(serialModem.readable()) { 00221 char c = serialModem.getc(); 00222 sum = (c==resp[sum]) ? sum+1 : 0; 00223 if(sum == len)break; 00224 } 00225 if(timeCnt.read() > timeout) { 00226 timeCnt.stop(); 00227 timeCnt.reset(); 00228 return -1; 00229 } 00230 } 00231 00232 timeCnt.stop(); 00233 timeCnt.reset(); 00234 00235 if(type == CMD) { 00236 while(serialModem.readable()) { 00237 char c = serialModem.getc(); 00238 } 00239 00240 } 00241 return 0; 00242 } 00243 00244 int Modem::sendCmdAndWaitForResp(const char* data, const char *resp, unsigned timeout,DataType type) 00245 { 00246 sendCmd(data); 00247 return waitForResp(resp,timeout,type); 00248 }
Generated on Thu Jul 14 2022 02:13:22 by
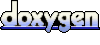