
uses pushing box to write to google spreadsheets
Dependencies: GSM_PUSHING_BOX_STATE_MACHINE MBed_Adafruit-GPS-Library SDFileSystem mbed
Fork of DCS by
TCPSocketConnection.cpp
00001 /* 00002 TCPSocketConnection.cpp 00003 2014 Copyright (c) Seeed Technology Inc. All right reserved. 00004 00005 Author:lawliet zou(lawliet.zou@gmail.com) 00006 2014-2-24 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00021 */ 00022 00023 #include "TCPSocketConnection.h" 00024 00025 TCPSocketConnection::TCPSocketConnection() 00026 { 00027 } 00028 00029 int TCPSocketConnection::connect(const char* host, const int port) 00030 { 00031 printf(">%s<",host); 00032 if (_sock_fd < 0) { 00033 _sock_fd = gprs->new_socket(); 00034 if (_sock_fd < 0) { 00035 return -1; 00036 } 00037 } 00038 00039 if (!gprs->connect(_sock_fd, TCP, host, port)) { 00040 return false; 00041 } 00042 return true; 00043 } 00044 00045 bool TCPSocketConnection::is_connected(void) 00046 { 00047 return gprs->is_connected(_sock_fd); 00048 } 00049 00050 int TCPSocketConnection::send(char* data, int length) 00051 { 00052 int size = gprs->wait_writeable(_sock_fd, length); 00053 if (size < 0) { 00054 return -1; 00055 } 00056 if (size > length) { 00057 size = length; 00058 } 00059 return gprs->send(_sock_fd, data, size); 00060 } 00061 00062 int TCPSocketConnection::send_all(char* data, int length) 00063 { 00064 return send(data,length); 00065 } 00066 00067 int TCPSocketConnection::receive(char* data, int length) 00068 { 00069 #if 0 00070 if (size < 0) { 00071 return -1; 00072 } 00073 if(size == 0) { 00074 size = gprs->wait_readable(_sock_fd, DEFAULT_TIMEOUT); 00075 } 00076 00077 if(size > length) { 00078 size = size - length; 00079 } else { 00080 length = size; 00081 size = -1; 00082 } 00083 #endif 00084 int size = gprs->wait_readable(_sock_fd, DEFAULT_TIMEOUT); 00085 return gprs->recv(_sock_fd, data, size>length?length:size); 00086 } 00087 00088 int TCPSocketConnection::receive_all(char* data, int length) 00089 { 00090 return receive(data,length); 00091 }
Generated on Thu Jul 14 2022 02:13:22 by
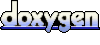