Fork of BlueNRG library to be compatible with bluetooth demo application
Dependents: Nucleo_BLE_Demo Nucleo_BLE_Demo
Fork of Nucleo_BLE_BlueNRG by
btle.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 00018 #include "btle.h" 00019 #include "public/Gap.h" 00020 #include "public/GapEvents.h" 00021 #include "BlueNRGGap.h" 00022 #include "BlueNRGGattServer.h" 00023 #include "Utils.h" 00024 00025 #ifdef __cplusplus 00026 extern "C" { 00027 #endif 00028 00029 00030 /* C File Includes ------------------------------------------------------------------*/ 00031 #include "hal_types.h" 00032 #include "hci.h" 00033 #include "bluenrg_hci.h" 00034 #include "gp_timer.h" 00035 #include "hal.h" 00036 #include "osal.h" 00037 #include "hci_internal.h" 00038 #include "bluenrg_hci_internal.h" 00039 #include "bluenrg_gap.h" 00040 #include "sm.h" 00041 #include <stdio.h> 00042 #include <string.h> 00043 #include "role_type.h" 00044 #include "debug.h" 00045 00046 #ifdef __cplusplus 00047 } 00048 #endif 00049 00050 00051 static void btle_handler(/*ble_evt_t * p_ble_evt*/); 00052 void HCI_Input(tHciDataPacket * hciReadPacket); 00053 00054 //#define BDADDR_SIZE 6 00055 //tHalUint8 bdaddr[BDADDR_SIZE]= {0xaa, 0x00, 0x00, 0xE1, 0x80, 0x02}; 00056 00057 uint16_t g_gap_service_handle = 0; 00058 uint16_t g_appearance_char_handle = 0; 00059 uint16_t g_device_name_char_handle = 0; 00060 00061 /* Private variables ---------------------------------------------------------*/ 00062 volatile uint8_t set_connectable = 1; 00063 00064 /**************************************************************************/ 00065 /*! 00066 @brief Initialises BTLE and the underlying HW/Device 00067 00068 @returns 00069 */ 00070 /**************************************************************************/ 00071 void btle_init(bool isSetAddress) 00072 { 00073 DEBUG("btle_init>>\n\r"); 00074 tHalUint8 *bleAddr; 00075 int ret; 00076 uint16_t service_handle, dev_name_char_handle, appearance_char_handle; 00077 00078 //HAL_Init(); 00079 00080 /* Configure the User Button in GPIO Mode */ 00081 //BSP_PB_Init(BUTTON_KEY, BUTTON_MODE_GPIO); 00082 00083 /* Configure the system clock */ 00084 //SystemClock_Config(); 00085 00086 /* Delay needed only to be able to acces the JTAG interface after reset 00087 if it will be disabled later. */ 00088 Clock_Wait(500); 00089 00090 /* Initialize the BlueNRG SPI driver */ 00091 BNRG_SPI_Init(); 00092 00093 /* Initialize the BlueNRG HCI */ 00094 HCI_Init(); 00095 00096 /* Reset BlueNRG SPI interface */ 00097 BlueNRG_RST(); 00098 00099 /* The Nucleo board must be configured as SERVER */ 00100 //check if issetAddress is set than set address. 00101 if(isSetAddress) 00102 { 00103 bleAddr = BlueNRGGap::getInstance().getAddress(); 00104 00105 tHalUint8 bdaddr[BDADDR_SIZE]; 00106 Osal_MemCpy(bdaddr, bleAddr, BDADDR_SIZE); 00107 00108 ret = aci_hal_write_config_data(CONFIG_DATA_PUBADDR_OFFSET, 00109 CONFIG_DATA_PUBADDR_LEN, 00110 bdaddr); 00111 } 00112 00113 ret = aci_gatt_init(); 00114 //GAP is always in PERIPHERAL _ROLE as mbed does not support Master role at the moment 00115 ret = aci_gap_init(GAP_PERIPHERAL_ROLE, &service_handle, &dev_name_char_handle, &appearance_char_handle); 00116 00117 g_gap_service_handle = service_handle; 00118 g_appearance_char_handle = appearance_char_handle; 00119 g_device_name_char_handle = dev_name_char_handle; 00120 //Device Name is set from Accumulate Adv Data Payload or through setDeviceName API 00121 /*ret = aci_gatt_update_char_value(service_handle, dev_name_char_handle, 0, 00122 strlen(name), (tHalUint8 *)name);*/ 00123 00124 return; 00125 } 00126 00127 void User_Process() 00128 { 00129 if(set_connectable){ 00130 setConnectable(); 00131 set_connectable = FALSE; 00132 } 00133 } 00134 00135 void setConnectable(void) 00136 { 00137 tBleStatus ret; 00138 00139 const char local_name[] = {AD_TYPE_COMPLETE_LOCAL_NAME,'B','l','u','e','N','R','G', '1', '2'}; 00140 00141 /* disable scan response */ 00142 hci_le_set_scan_resp_data(0,NULL); 00143 00144 00145 ret = aci_gap_set_discoverable(ADV_IND, 0, 0, PUBLIC_ADDR, NO_WHITE_LIST_USE, 00146 8, local_name, 0, NULL, 0, 0); 00147 00148 } 00149 00150 /**************************************************************************/ 00151 /*! 00152 @brief 00153 00154 @param[in] p_ble_evt 00155 00156 @returns 00157 */ 00158 /**************************************************************************/ 00159 static void btle_handler() 00160 { 00161 00162 } 00163 00164 00165 void SPI_Poll(void) 00166 { 00167 //HAL_GPIO_EXTI_Callback_Poll(BNRG_SPI_EXTI_PIN); 00168 return; 00169 } 00170 00171 #ifdef __cplusplus 00172 extern "C" { 00173 #endif 00174 00175 /**************************************************************************/ 00176 /*! 00177 @brief Handle HCI Stack Event 00178 00179 @param[in] pckt 00180 Event Packet sent by the stack to be decoded 00181 00182 @returns 00183 */ 00184 /**************************************************************************/ 00185 extern void HCI_Event_CB(void *pckt) { 00186 00187 hci_uart_pckt *hci_pckt = (hci_uart_pckt*)pckt; 00188 hci_event_pckt *event_pckt = (hci_event_pckt*)hci_pckt->data; 00189 00190 if(hci_pckt->type != HCI_EVENT_PKT) 00191 return; 00192 00193 switch(event_pckt->evt){ 00194 00195 case EVT_DISCONN_COMPLETE: 00196 { 00197 evt_disconn_complete *evt = (evt_disconn_complete*)event_pckt; 00198 00199 BlueNRGGap::getInstance().processDisconnectionEvent(evt->handle, BlueNRGGap::REMOTE_USER_TERMINATED_CONNECTION); 00200 } 00201 break; 00202 00203 case EVT_LE_META_EVENT: 00204 { 00205 evt_le_meta_event *evt = (evt_le_meta_event *)event_pckt->data; 00206 00207 switch(evt->subevent){ 00208 case EVT_LE_CONN_COMPLETE: 00209 { 00210 evt_le_connection_complete *cc = (evt_le_connection_complete *)evt->data; 00211 00212 BlueNRGGap::getInstance().setConnectionHandle(cc->handle); 00213 BlueNRGGap::ConnectionParams_t connectionParams; 00214 BlueNRGGap::getInstance().getPreferredConnectionParams(&connectionParams); 00215 BlueNRGGap::getInstance().processConnectionEvent(cc->handle, (const BlueNRGGap::ConnectionParams_t *)&connectionParams); 00216 } 00217 break; 00218 } 00219 } 00220 break; 00221 00222 case EVT_VENDOR: 00223 { 00224 evt_blue_aci *blue_evt = (evt_blue_aci*)event_pckt->data; 00225 switch(blue_evt->ecode){ 00226 00227 case EVT_BLUE_GATT_READ_PERMIT_REQ: 00228 { 00229 DEBUG("EVT_BLUE_GATT_READ_PERMIT_REQ_OK\n\r"); 00230 evt_gatt_read_permit_req *pr = (evt_gatt_read_permit_req*)blue_evt->data; 00231 BlueNRGGattServer::getInstance().Read_Request_CB(pr->attr_handle); 00232 } 00233 break; 00234 00235 case EVT_BLUE_GATT_ATTRIBUTE_MODIFIED: 00236 { 00237 /* this callback is invoked when a GATT attribute is modified 00238 extract callback data and pass to suitable handler function */ 00239 evt_gatt_attr_modified *evt = (evt_gatt_attr_modified*)blue_evt->data; 00240 DEBUG("EVT_BLUE_GATT_ATTRIBUTE_MODIFIED\n\r"); 00241 //DEBUG("CharHandle 0x%x, length: 0x%x, Data: 0x%x\n\r",evt->attr_handle, evt->data_length, (uint16_t)evt->att_data[0]); 00242 DEBUG("CharHandle %d, length: %d, Data: %d\n\r",evt->attr_handle, evt->data_length, (uint16_t)evt->att_data[0]); 00243 00244 //Extract the GattCharacteristic from p_characteristics[] and find the properties mask 00245 GattCharacteristic *p_char = BlueNRGGattServer::getInstance().getCharacteristicFromHandle(evt->attr_handle-1); 00246 if(p_char!=NULL) { 00247 DEBUG("getProperties 0x%x\n\r",p_char->getProperties()); 00248 if((p_char->getProperties() & (GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY 00249 | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_INDICATE))) { 00250 00251 //Now Check if data written in Enable or Disable 00252 if((uint16_t)evt->att_data[0]==1) { 00253 //DEBUG("Notify ENABLED\n\r"); 00254 BlueNRGGattServer::getInstance().handleEvent(GattServerEvents::GATT_EVENT_UPDATES_ENABLED, evt->attr_handle); 00255 } 00256 else { 00257 //DEBUG("Notify DISABLED\n\r"); 00258 BlueNRGGattServer::getInstance().handleEvent(GattServerEvents::GATT_EVENT_UPDATES_DISABLED, evt->attr_handle); 00259 } 00260 } 00261 00262 //Check is attr handle property is WRITEABLE, if yes, generate GATT_EVENT_DATA_WRITTEN Event 00263 if((p_char->getProperties() & 00264 (GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE| 00265 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE))) { 00266 GattCharacteristicWriteCBParams params; 00267 params.charHandle = evt->attr_handle-1; 00268 params.op = GattCharacteristicWriteCBParams::GATTS_CHAR_OP_WRITE_CMD; 00269 params.data = evt->att_data; 00270 params.len = evt->data_length; 00271 params.offset = 0; 00272 00273 DEBUG("Data written condition\n\r"); 00274 //BlueNRGGattServer::getInstance().handleEvent(GattServerEvents::GATT_EVENT_DATA_WRITTEN, evt->attr_handle); 00275 BlueNRGGattServer::getInstance().handleDataWrittenEvent(¶ms); 00276 //Write the actual Data to the Attr Handle? (uint8_1[])evt->att_data contains the data 00277 if ((p_char->getValueAttribute().getValuePtr() != NULL) && (p_char->getValueAttribute().getInitialLength() > 0)) { 00278 BlueNRGGattServer::getInstance().updateValue(p_char->getValueAttribute().getHandle(), 00279 p_char->getValueAttribute().getValuePtr(), p_char->getValueAttribute().getInitialLength(), false /* localOnly */); 00280 } 00281 } 00282 } 00283 } 00284 break; 00285 00286 //Any cases for Data Sent Notifications? 00287 case EVT_BLUE_GATT_NOTIFICATION: 00288 //This is only relevant for Client Side Event 00289 DEBUG("EVT_BLUE_GATT_NOTIFICATION"); 00290 break; 00291 case EVT_BLUE_GATT_INDICATION: 00292 //This is only relevant for Client Side Event 00293 DEBUG("EVT_BLUE_GATT_INDICATION"); 00294 break; 00295 00296 case EVT_BLUE_GATT_PROCEDURE_COMPLETE: 00297 DEBUG("EVT_BLUE_GATT_PROCEDURE_COMPLETE"); 00298 break; 00299 } 00300 } 00301 break; 00302 } 00303 00304 return ; 00305 } 00306 00307 00308 #ifdef __cplusplus 00309 } 00310 #endif
Generated on Sat Jul 16 2022 01:53:16 by
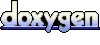