Fork of BlueNRG library to be compatible with bluetooth demo application
Dependents: Nucleo_BLE_Demo Nucleo_BLE_Demo
Fork of Nucleo_BLE_BlueNRG by
Utils.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "Utils.h" 00018 00019 #if NEED_CONSOLE_OUTPUT 00020 Serial pc(USBTX, USBRX); 00021 #endif /* #if NEED_CONSOLE_OUTPUT */ 00022 00023 /**************************************************************************/ 00024 /*! 00025 @brief sets values of EN_HIGH_POWER and PA_LEVEL corresponding to dBMLevel of tx power 00026 00027 @returns value of tx power in dbm actually set 00028 00029 @params[in] dBMLevel 00030 dBMLevel of tx power to be set 00031 00032 @params[in] dBMLevel 00033 dBMLevel of tx power to be set 00034 00035 @endcode 00036 */ 00037 /**************************************************************************/ 00038 double getHighPowerAndPALevelValue(int8_t dBMLevel, int8_t& EN_HIGH_POWER, int8_t& PA_LEVEL) { 00039 double dbm = (double) dBMLevel; 00040 if(dbm<-18.0) { 00041 dbm = -18; 00042 EN_HIGH_POWER = 0; 00043 PA_LEVEL = 0; 00044 } 00045 else if(dbm>8.0) { 00046 dbm = 8; 00047 EN_HIGH_POWER = 1; 00048 PA_LEVEL = 7; 00049 } 00050 00051 // As a policy we are setting tx power level to the higher side 00052 if((dbm>-18.0) && (dbm<=-15)) { 00053 // set tx power to -15dBM 00054 EN_HIGH_POWER = 0; 00055 PA_LEVEL = 0; 00056 } 00057 else if((dbm>-15) && (dbm<=-14.7)) { 00058 // set tx power to -14.7dBM 00059 EN_HIGH_POWER = 0; 00060 PA_LEVEL = 1; 00061 } 00062 else if((dbm>-14.7) && (dbm<=-11.7)) { 00063 // set tx power to -11.7dBM 00064 EN_HIGH_POWER = 1; 00065 PA_LEVEL = 1; 00066 } 00067 else if((dbm>-11.7) && (dbm<=-11.4)) { 00068 // set tx power to -11.4dBM 00069 EN_HIGH_POWER = 0; 00070 PA_LEVEL = 2; 00071 } 00072 else if((dbm>-11.4) && (dbm<=-8.4)) { 00073 // set tx power to -8.4dBM 00074 EN_HIGH_POWER = 1; 00075 PA_LEVEL = 2; 00076 } 00077 else if((dbm>-8.4) && (dbm<=-8.1)) { 00078 // set tx power to -8.1dBM 00079 EN_HIGH_POWER = 0; 00080 PA_LEVEL = 3; 00081 } 00082 else if((dbm>-8.1) && (dbm<=-5.1)) { 00083 // set tx power to -5.1dBM 00084 EN_HIGH_POWER = 1; 00085 PA_LEVEL = 3; 00086 } 00087 else if((dbm>-5.1) && (dbm<=-4.9)) { 00088 // set tx power to -4.9dBM 00089 EN_HIGH_POWER = 0; 00090 PA_LEVEL = 4; 00091 } 00092 else if((dbm>-4.9) && (dbm<=-2.1)) { 00093 // set tx power to -2.1dBM 00094 EN_HIGH_POWER = 1; 00095 PA_LEVEL = 4; 00096 } 00097 else if((dbm>-2.1) && (dbm<=-1.6)) { 00098 // set tx power to -1.6dBM 00099 EN_HIGH_POWER = 0; 00100 PA_LEVEL = 5; 00101 } 00102 else if((dbm>-1.6) && (dbm<=1.4)) { 00103 // set tx power to -1.6dBM 00104 EN_HIGH_POWER = 1; 00105 PA_LEVEL = 5; 00106 } 00107 else if((dbm>1.4) && (dbm<=1.7)) { 00108 // set tx power to 1.7dBM 00109 EN_HIGH_POWER = 0; 00110 PA_LEVEL = 6; 00111 } 00112 else if((dbm>1.7) && (dbm<=4.7)) { 00113 // set tx power to 4.7dBM 00114 EN_HIGH_POWER = 1; 00115 PA_LEVEL = 6; 00116 } 00117 else if((dbm>4.7) && (dbm<=5.0)) { 00118 // set tx power to 5.0dBM 00119 EN_HIGH_POWER = 0; 00120 PA_LEVEL = 7; 00121 } 00122 else if((dbm>5.0) && (dbm<=8)) { 00123 // set tx power to 8.0dBM 00124 EN_HIGH_POWER = 1; 00125 PA_LEVEL = 7; 00126 } 00127 00128 return dbm; 00129 }
Generated on Sat Jul 16 2022 01:53:16 by
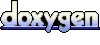