Fork of BlueNRG library to be compatible with bluetooth demo application
Dependents: Nucleo_BLE_Demo Nucleo_BLE_Demo
Fork of Nucleo_BLE_BlueNRG by
BlueNRGGap.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "BlueNRGDevice.h" 00018 #include "mbed.h" 00019 #include "Payload.h" 00020 #include "Utils.h" 00021 00022 //Local Variables 00023 const char *local_name = NULL; 00024 uint8_t local_name_length = 0; 00025 const uint8_t *scan_response_payload = NULL; 00026 uint8_t scan_rsp_length = 0; 00027 uint8_t servUuidlength = 0; 00028 uint8_t* servUuidData = NULL; 00029 00030 uint32_t advtInterval = 0; 00031 00032 /**************************************************************************/ 00033 /*! 00034 @brief Sets the advertising parameters and payload for the device. 00035 Note: Some data types give error when their adv data is updated using aci_gap_update_adv_data() API 00036 00037 @param[in] params 00038 Basic advertising details, including the advertising 00039 delay, timeout and how the device should be advertised 00040 @params[in] advData 00041 The primary advertising data payload 00042 @params[in] scanResponse 00043 The optional Scan Response payload if the advertising 00044 type is set to \ref GapAdvertisingParams::ADV_SCANNABLE_UNDIRECTED 00045 in \ref GapAdveritinngParams 00046 00047 @returns \ref ble_error_t 00048 00049 @retval BLE_ERROR_NONE 00050 Everything executed properly 00051 00052 @retval BLE_ERROR_BUFFER_OVERFLOW 00053 The proposed action would cause a buffer overflow. All 00054 advertising payloads must be <= 31 bytes, for example. 00055 00056 @retval BLE_ERROR_NOT_IMPLEMENTED 00057 A feature was requested that is not yet supported in the 00058 nRF51 firmware or hardware. 00059 00060 @retval BLE_ERROR_PARAM_OUT_OF_RANGE 00061 One of the proposed values is outside the valid range. 00062 00063 @section EXAMPLE 00064 00065 @code 00066 00067 @endcode 00068 */ 00069 /**************************************************************************/ 00070 ble_error_t BlueNRGGap::setAdvertisingData(const GapAdvertisingData &advData, const GapAdvertisingData &scanResponse) 00071 { 00072 DEBUG("BlueNRGGap::setAdvertisingData\n\r"); 00073 /* Make sure we don't exceed the advertising payload length */ 00074 if (advData.getPayloadLen() > GAP_ADVERTISING_DATA_MAX_PAYLOAD) { 00075 return BLE_ERROR_BUFFER_OVERFLOW; 00076 } 00077 00078 /* Make sure we have a payload! */ 00079 if (advData.getPayloadLen() <= 0) { 00080 return BLE_ERROR_PARAM_OUT_OF_RANGE; 00081 } else { 00082 PayloadPtr loadPtr(advData.getPayload(), advData.getPayloadLen()); 00083 for(uint8_t index=0; index<loadPtr.getPayloadUnitCount(); index++) { 00084 PayloadUnit unit = loadPtr.getUnitAtIndex(index); 00085 00086 DEBUG("adData[%d].length=%d\n\r", index,(uint8_t)(*loadPtr.getUnitAtIndex(index).getLenPtr())); 00087 DEBUG("adData[%d].AdType=0x%x\n\r", index,(uint8_t)(*loadPtr.getUnitAtIndex(index).getAdTypePtr())); 00088 00089 switch(*loadPtr.getUnitAtIndex(index).getAdTypePtr()) { 00090 case GapAdvertisingData::FLAGS: /* ref *Flags */ 00091 { 00092 //Check if Flags are OK. BlueNRG only supports LE Mode. 00093 uint8_t *flags = loadPtr.getUnitAtIndex(index).getDataPtr(); 00094 if((*flags & GapAdvertisingData::BREDR_NOT_SUPPORTED) != GapAdvertisingData::BREDR_NOT_SUPPORTED) { 00095 DEBUG("BlueNRG does not support BR/EDR Mode"); 00096 return BLE_ERROR_PARAM_OUT_OF_RANGE; 00097 } 00098 00099 break; 00100 } 00101 case GapAdvertisingData::INCOMPLETE_LIST_16BIT_SERVICE_IDS: /**< Incomplete list of 16-bit Service IDs */ 00102 { 00103 break; 00104 } 00105 case GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS: /**< Complete list of 16-bit Service IDs */ 00106 { 00107 DEBUG("Advertising type: COMPLETE_LIST_16BIT_SERVICE_IDS\n\r"); 00108 DEBUG("Advertising type: COMPLETE_LIST_16BIT_SERVICE_IDS\n"); 00109 #if 0 00110 int err = aci_gap_update_adv_data(*loadPtr.getUnitAtIndex(index).getLenPtr(), loadPtr.getUnitAtIndex(index).getAdTypePtr()); 00111 if(BLE_STATUS_SUCCESS!=err) { 00112 DEBUG("error occurred while adding adv data\n"); 00113 return BLE_ERROR_PARAM_OUT_OF_RANGE; // no other suitable error code is available 00114 } 00115 #endif 00116 break; 00117 } 00118 case GapAdvertisingData::INCOMPLETE_LIST_32BIT_SERVICE_IDS: /**< Incomplete list of 32-bit Service IDs (not relevant for Bluetooth 4.0) */ 00119 { 00120 break; 00121 } 00122 case GapAdvertisingData::COMPLETE_LIST_32BIT_SERVICE_IDS: /**< Complete list of 32-bit Service IDs (not relevant for Bluetooth 4.0) */ 00123 { 00124 break; 00125 } 00126 case GapAdvertisingData::INCOMPLETE_LIST_128BIT_SERVICE_IDS: /**< Incomplete list of 128-bit Service IDs */ 00127 { 00128 break; 00129 } 00130 case GapAdvertisingData::COMPLETE_LIST_128BIT_SERVICE_IDS: /**< Complete list of 128-bit Service IDs */ 00131 { 00132 break; 00133 } 00134 case GapAdvertisingData::SHORTENED_LOCAL_NAME: /**< Shortened Local Name */ 00135 { 00136 break; 00137 } 00138 case GapAdvertisingData::COMPLETE_LOCAL_NAME: /**< Complete Local Name */ 00139 { 00140 DEBUG("Advertising type: COMPLETE_LOCAL_NAME\n\r"); 00141 loadPtr.getUnitAtIndex(index).printDataAsString(); 00142 local_name_length = *loadPtr.getUnitAtIndex(index).getLenPtr()-1; 00143 local_name = (const char*)loadPtr.getUnitAtIndex(index).getAdTypePtr(); 00144 //COMPLETE_LOCAL_NAME is only advertising device name. Gatt Device Name is not the same.(Must be set right after GAP/GATT init?) 00145 00146 DEBUG("device_name length=%d\n\r", local_name_length); 00147 break; 00148 } 00149 case GapAdvertisingData::TX_POWER_LEVEL: /**< TX Power Level (in dBm) */ 00150 { 00151 DEBUG("Advertising type: TX_POWER_LEVEL\n\r"); 00152 int8_t dbm = *loadPtr.getUnitAtIndex(index).getDataPtr(); 00153 int8_t enHighPower = 0; 00154 int8_t paLevel = 0; 00155 int8_t dbmActuallySet = getHighPowerAndPALevelValue(dbm, enHighPower, paLevel); 00156 DEBUG("dbm=%d, dbmActuallySet=%d\n\r", dbm, dbmActuallySet); 00157 DEBUG("enHighPower=%d, paLevel=%d\n\r", enHighPower, paLevel); 00158 aci_hal_set_tx_power_level(enHighPower, paLevel); 00159 break; 00160 } 00161 case GapAdvertisingData::DEVICE_ID: /**< Device ID */ 00162 { 00163 break; 00164 } 00165 case GapAdvertisingData::SLAVE_CONNECTION_INTERVAL_RANGE: /**< Slave :Connection Interval Range */ 00166 { 00167 break; 00168 } 00169 case GapAdvertisingData::SERVICE_DATA: /**< Service Data */ 00170 { 00171 break; 00172 } 00173 case GapAdvertisingData::APPEARANCE: 00174 { 00175 /* 00176 Tested with GapAdvertisingData::GENERIC_PHONE. 00177 for other appearances BLE Scanner android app is not behaving properly 00178 */ 00179 DEBUG("Advertising type: APPEARANCE\n\r"); 00180 const char *deviceAppearance = NULL; 00181 deviceAppearance = (const char*)loadPtr.getUnitAtIndex(index).getDataPtr(); // to be set later when startAdvertising() is called 00182 00183 uint8_t Appearance[2]; 00184 uint16_t devP = (uint16_t)*deviceAppearance; 00185 STORE_LE_16(Appearance, (uint16_t)devP); 00186 00187 DEBUG("input: deviceAppearance= 0x%x 0x%x..., strlen(deviceAppearance)=%d\n\r", Appearance[1], Appearance[0], (uint8_t)*loadPtr.getUnitAtIndex(index).getLenPtr()-1); /**< \ref Appearance */ 00188 00189 aci_gatt_update_char_value(g_gap_service_handle, g_appearance_char_handle, 0, 2, (tHalUint8 *)deviceAppearance);//not using array Appearance[2] 00190 break; 00191 } 00192 case GapAdvertisingData::ADVERTISING_INTERVAL: /**< Advertising Interval */ 00193 { 00194 advtInterval = (uint16_t)(*loadPtr.getUnitAtIndex(index).getDataPtr()); 00195 DEBUG("advtInterval=%d\n\r", advtInterval); 00196 break; 00197 } 00198 case GapAdvertisingData::MANUFACTURER_SPECIFIC_DATA: /**< Manufacturer Specific Data */ 00199 { 00200 break; 00201 } 00202 00203 } 00204 } 00205 //Set the SCAN_RSP Payload 00206 scan_response_payload = scanResponse.getPayload(); 00207 scan_rsp_length = scanResponse.getPayloadLen(); 00208 } 00209 return BLE_ERROR_NONE; 00210 } 00211 00212 /**************************************************************************/ 00213 /*! 00214 @brief Starts the BLE HW, initialising any services that were 00215 added before this function was called. 00216 00217 @note All services must be added before calling this function! 00218 00219 @returns ble_error_t 00220 00221 @retval BLE_ERROR_NONE 00222 Everything executed properly 00223 00224 @section EXAMPLE 00225 00226 @code 00227 00228 @endcode 00229 */ 00230 /**************************************************************************/ 00231 ble_error_t BlueNRGGap::startAdvertising(const GapAdvertisingParams ¶ms) 00232 { 00233 /* Make sure we support the advertising type */ 00234 if (params.getAdvertisingType() == GapAdvertisingParams::ADV_CONNECTABLE_DIRECTED) { 00235 /* ToDo: This requires a propery security implementation, etc. */ 00236 return BLE_ERROR_NOT_IMPLEMENTED; 00237 } 00238 00239 /* Check interval range */ 00240 if (params.getAdvertisingType() == GapAdvertisingParams::ADV_NON_CONNECTABLE_UNDIRECTED) { 00241 /* Min delay is slightly longer for unconnectable devices */ 00242 if ((params.getInterval() < GAP_ADV_PARAMS_INTERVAL_MIN_NONCON) || 00243 (params.getInterval() > GAP_ADV_PARAMS_INTERVAL_MAX)) { 00244 return BLE_ERROR_PARAM_OUT_OF_RANGE; 00245 } 00246 } else { 00247 if ((params.getInterval() < GAP_ADV_PARAMS_INTERVAL_MIN) || 00248 (params.getInterval() > GAP_ADV_PARAMS_INTERVAL_MAX)) { 00249 return BLE_ERROR_PARAM_OUT_OF_RANGE; 00250 } 00251 } 00252 00253 /* Check timeout is zero for Connectable Directed */ 00254 if ((params.getAdvertisingType() == GapAdvertisingParams::ADV_CONNECTABLE_DIRECTED) && (params.getTimeout() != 0)) { 00255 /* Timeout must be 0 with this type, although we'll never get here */ 00256 /* since this isn't implemented yet anyway */ 00257 return BLE_ERROR_PARAM_OUT_OF_RANGE; 00258 } 00259 00260 /* Check timeout for other advertising types */ 00261 if ((params.getAdvertisingType() != GapAdvertisingParams::ADV_CONNECTABLE_DIRECTED) && 00262 (params.getTimeout() > GAP_ADV_PARAMS_TIMEOUT_MAX)) { 00263 return BLE_ERROR_PARAM_OUT_OF_RANGE; 00264 } 00265 00266 tBleStatus ret; 00267 const LongUUIDBytes_t HRM_SERVICE_UUID_128 = {0x18, 0x0D}; 00268 /* set scan response data */ 00269 hci_le_set_scan_resp_data(scan_rsp_length, scan_response_payload); /*int hci_le_set_scan_resp_data(uint8_t length, const uint8_t data[]);*/ 00270 00271 /*aci_gap_set_discoverable(Advertising_Event_Type, Adv_min_intvl, Adv_Max_Intvl, Addr_Type, Adv_Filter_Policy, 00272 Local_Name_Length, local_name, service_uuid_length, service_uuid_list, Slave_conn_intvl_min, Slave_conn_intvl_max);*/ 00273 /*LINK_LAYER.H DESCRIBES THE ADVERTISING TYPES*/ 00274 00275 char* name = NULL; 00276 uint8_t nameLen = 0; 00277 if(local_name!=NULL) { 00278 name = (char*)local_name; 00279 DEBUG("name=%s\n\r", name); 00280 nameLen = local_name_length; 00281 } else { 00282 char str[] = "ST_BLE_DEV"; 00283 name = new char[strlen(str)+1]; 00284 name[0] = AD_TYPE_COMPLETE_LOCAL_NAME; 00285 strcpy(name+1, str); 00286 nameLen = strlen(name); 00287 DEBUG("nameLen=%d\n\r", nameLen); 00288 DEBUG("name=%s\n\r", name); 00289 } 00290 00291 00292 advtInterval = params.getInterval(); // set advtInterval in case it is not already set by user application 00293 ret = aci_gap_set_discoverable(params.getAdvertisingType(), // Advertising_Event_Type 00294 0, // Adv_Interval_Min 00295 advtInterval, // Adv_Interval_Max 00296 PUBLIC_ADDR, // Address_Type 00297 NO_WHITE_LIST_USE, // Adv_Filter_Policy 00298 nameLen, //local_name_length, // Local_Name_Length 00299 (const char*)name, //local_name, // Local_Name 00300 servUuidlength, //Service_Uuid_Length 00301 servUuidData, //Service_Uuid_List 00302 0, // Slave_Conn_Interval_Min 00303 0); // Slave_Conn_Interval_Max 00304 00305 state.advertising = 1; 00306 00307 return BLE_ERROR_NONE; 00308 } 00309 00310 /**************************************************************************/ 00311 /*! 00312 @brief Stops the BLE HW and disconnects from any devices 00313 00314 @returns ble_error_t 00315 00316 @retval BLE_ERROR_NONE 00317 Everything executed properly 00318 00319 @section EXAMPLE 00320 00321 @code 00322 00323 @endcode 00324 */ 00325 /**************************************************************************/ 00326 ble_error_t BlueNRGGap::stopAdvertising(void) 00327 { 00328 tBleStatus ret; 00329 00330 if(state.advertising == 1) { 00331 //Set non-discoverable to stop advertising 00332 ret = aci_gap_set_non_discoverable(); 00333 00334 if (ret != BLE_STATUS_SUCCESS){ 00335 DEBUG("Error in stopping advertisement!!\n\r") ; 00336 return BLE_ERROR_PARAM_OUT_OF_RANGE ; //Not correct Error Value 00337 //FIXME: Define Error values equivalent to BlueNRG Error Codes. 00338 } 00339 DEBUG("Advertisement stopped!!\n\r") ; 00340 //Set GapState_t::advertising state 00341 state.advertising = 0; 00342 } 00343 00344 return BLE_ERROR_NONE; 00345 } 00346 00347 /**************************************************************************/ 00348 /*! 00349 @brief Disconnects if we are connected to a central device 00350 00351 @returns ble_error_t 00352 00353 @retval BLE_ERROR_NONE 00354 Everything executed properly 00355 00356 @section EXAMPLE 00357 00358 @code 00359 00360 @endcode 00361 */ 00362 /**************************************************************************/ 00363 ble_error_t BlueNRGGap::disconnect(Gap::DisconnectionReason_t reason) 00364 { 00365 tBleStatus ret; 00366 //For Reason codes check BlueTooth HCI Spec 00367 00368 if(m_connectionHandle != BLE_CONN_HANDLE_INVALID) { 00369 ret = aci_gap_terminate(m_connectionHandle, 0x16);//0x16 Connection Terminated by Local Host. 00370 00371 if (ret != BLE_STATUS_SUCCESS){ 00372 DEBUG("Error in GAP termination!!\n\r") ; 00373 return BLE_ERROR_PARAM_OUT_OF_RANGE ; //Not correct Error Value 00374 //FIXME: Define Error values equivalent to BlueNRG Error Codes. 00375 } 00376 00377 //DEBUG("Disconnected from localhost!!\n\r") ; 00378 m_connectionHandle = BLE_CONN_HANDLE_INVALID; 00379 } 00380 00381 return BLE_ERROR_NONE; 00382 } 00383 00384 /**************************************************************************/ 00385 /*! 00386 @brief Sets the 16-bit connection handle 00387 */ 00388 /**************************************************************************/ 00389 void BlueNRGGap::setConnectionHandle(uint16_t con_handle) 00390 { 00391 m_connectionHandle = con_handle; 00392 } 00393 00394 /**************************************************************************/ 00395 /*! 00396 @brief Gets the 16-bit connection handle 00397 */ 00398 /**************************************************************************/ 00399 uint16_t BlueNRGGap::getConnectionHandle(void) 00400 { 00401 return m_connectionHandle; 00402 } 00403 00404 /**************************************************************************/ 00405 /*! 00406 @brief Sets the BLE device address. SetAddress will reset the BLE 00407 device and re-initialize BTLE. Will not start advertising. 00408 00409 @returns ble_error_t 00410 00411 @section EXAMPLE 00412 00413 @code 00414 00415 @endcode 00416 */ 00417 /**************************************************************************/ 00418 ble_error_t BlueNRGGap::setAddress(addr_type_t type, const uint8_t address[6]) 00419 { 00420 tBleStatus ret; 00421 00422 if (type > ADDR_TYPE_RANDOM_PRIVATE_NON_RESOLVABLE) { 00423 return BLE_ERROR_PARAM_OUT_OF_RANGE; 00424 } 00425 00426 //copy address to bdAddr[6] 00427 for(int i=0; i<BDADDR_SIZE; i++) { 00428 bdaddr[i] = address[i]; 00429 //DEBUG("i[%d]:0x%x\n\r",i,bdaddr[i]); 00430 } 00431 00432 if(!isSetAddress) isSetAddress = true; 00433 00434 //Re-Init the BTLE Device with SetAddress as true 00435 //if(BlueNRGDevice::getIsInitialized())//Re-init only initialization is already done 00436 btle_init(isSetAddress); 00437 00438 //if (ret==BLE_STATUS_SUCCESS) 00439 return BLE_ERROR_NONE; 00440 } 00441 00442 /**************************************************************************/ 00443 /*! 00444 @brief Returns boolean if the address of the device has been set 00445 or not 00446 00447 @returns bool 00448 00449 @section EXAMPLE 00450 00451 @code 00452 00453 @endcode 00454 */ 00455 /**************************************************************************/ 00456 bool BlueNRGGap::getIsSetAddress() 00457 { 00458 return isSetAddress; 00459 } 00460 00461 /**************************************************************************/ 00462 /*! 00463 @brief Returns the address of the device if set 00464 00465 @returns ble_error_t 00466 00467 @section EXAMPLE 00468 00469 @code 00470 00471 @endcode 00472 */ 00473 /**************************************************************************/ 00474 tHalUint8* BlueNRGGap::getAddress() 00475 { 00476 if(isSetAddress) 00477 return bdaddr; 00478 else return NULL; 00479 } 00480 00481 /**************************************************************************/ 00482 /*! 00483 @brief obtains preferred connection params 00484 00485 @returns ble_error_t 00486 00487 @section EXAMPLE 00488 00489 @code 00490 00491 @endcode 00492 */ 00493 /**************************************************************************/ 00494 ble_error_t BlueNRGGap::getPreferredConnectionParams(ConnectionParams_t *params) 00495 { 00496 return BLE_ERROR_NONE; 00497 } 00498 00499 00500 /**************************************************************************/ 00501 /*! 00502 @brief sets preferred connection params 00503 00504 @returns ble_error_t 00505 00506 @section EXAMPLE 00507 00508 @code 00509 00510 @endcode 00511 */ 00512 /**************************************************************************/ 00513 ble_error_t BlueNRGGap::setPreferredConnectionParams(const ConnectionParams_t *params) 00514 { 00515 return BLE_ERROR_NONE; 00516 } 00517 00518 /**************************************************************************/ 00519 /*! 00520 @brief updates preferred connection params 00521 00522 @returns ble_error_t 00523 00524 @section EXAMPLE 00525 00526 @code 00527 00528 @endcode 00529 */ 00530 /**************************************************************************/ 00531 ble_error_t BlueNRGGap::updateConnectionParams(Handle_t handle, const ConnectionParams_t *params) 00532 { 00533 return BLE_ERROR_NONE; 00534 } 00535 00536 /**************************************************************************/ 00537 /*! 00538 @brief sets device name characteristic 00539 00540 @param[in] deviceName 00541 pointer to device name to be set 00542 00543 @returns ble_error_t 00544 00545 @retval BLE_ERROR_NONE 00546 Everything executed properly 00547 00548 @section EXAMPLE 00549 00550 @code 00551 00552 @endcode 00553 */ 00554 /**************************************************************************/ 00555 ble_error_t BlueNRGGap::setDeviceName(const uint8_t *deviceName) 00556 { 00557 int ret; 00558 uint8_t nameLen = 0; 00559 00560 DeviceName = (uint8_t *)deviceName; 00561 //DEBUG("SetDeviceName=%s\n\r", DeviceName); 00562 00563 nameLen = strlen((const char*)DeviceName); 00564 //DEBUG("DeviceName Size=%d\n\r", nameLen); 00565 00566 ret = aci_gatt_update_char_value(g_gap_service_handle, 00567 g_device_name_char_handle, 00568 0, 00569 nameLen, 00570 (tHalUint8 *)DeviceName); 00571 00572 if(ret){ 00573 DEBUG("device set name failed\n\r"); 00574 return BLE_ERROR_PARAM_OUT_OF_RANGE;//TODO:Wrong error code 00575 } 00576 00577 return BLE_ERROR_NONE; 00578 } 00579 00580 /**************************************************************************/ 00581 /*! 00582 @brief gets device name characteristic 00583 00584 @param[in] deviceName 00585 pointer to device name 00586 00587 00588 @param[in] lengthP 00589 pointer to device name length 00590 00591 @returns ble_error_t 00592 00593 @retval BLE_ERROR_NONE 00594 Everything executed properly 00595 00596 @section EXAMPLE 00597 00598 @code 00599 00600 @endcode 00601 */ 00602 /**************************************************************************/ 00603 ble_error_t BlueNRGGap::getDeviceName(uint8_t *deviceName, unsigned *lengthP) 00604 { 00605 int ret; 00606 00607 if(DeviceName==NULL) 00608 return BLE_ERROR_PARAM_OUT_OF_RANGE; 00609 00610 strcpy((char*)deviceName, (const char*)DeviceName); 00611 //DEBUG("GetDeviceName=%s\n\r", deviceName); 00612 00613 *lengthP = strlen((const char*)DeviceName); 00614 //DEBUG("DeviceName Size=%d\n\r", *lengthP); 00615 00616 return BLE_ERROR_NONE; 00617 } 00618 00619 /**************************************************************************/ 00620 /*! 00621 @brief sets device appearance characteristic 00622 00623 @param[in] appearance 00624 device appearance 00625 00626 @returns ble_error_t 00627 00628 @retval BLE_ERROR_NONE 00629 Everything executed properly 00630 00631 @section EXAMPLE 00632 00633 @code 00634 00635 @endcode 00636 */ 00637 /**************************************************************************/ 00638 ble_error_t BlueNRGGap::setAppearance(uint16_t appearance) 00639 { 00640 /* 00641 Tested with GapAdvertisingData::GENERIC_PHONE. 00642 for other appearances BLE Scanner android app is not behaving properly 00643 */ 00644 //char deviceAppearance[2]; 00645 STORE_LE_16(deviceAppearance, appearance); 00646 DEBUG("input: incoming = %d deviceAppearance= 0x%x 0x%x\n\r", appearance, deviceAppearance[1], deviceAppearance[0]); 00647 00648 aci_gatt_update_char_value(g_gap_service_handle, g_appearance_char_handle, 0, 2, (tHalUint8 *)deviceAppearance); 00649 00650 return BLE_ERROR_NONE; 00651 } 00652 00653 /**************************************************************************/ 00654 /*! 00655 @brief gets device appearance 00656 00657 @param[in] appearance 00658 pointer to device appearance value 00659 00660 @returns ble_error_t 00661 00662 @retval BLE_ERROR_NONE 00663 Everything executed properly 00664 00665 @section EXAMPLE 00666 00667 @code 00668 00669 @endcode 00670 */ 00671 /**************************************************************************/ 00672 ble_error_t BlueNRGGap::getAppearance(uint16_t *appearanceP) 00673 { 00674 uint16_t devP; 00675 if(!appearanceP) return BLE_ERROR_PARAM_OUT_OF_RANGE; 00676 devP = ((uint16_t)(0x0000|deviceAppearance[0])) | (((uint16_t)(0x0000|deviceAppearance[1]))<<8); 00677 strcpy((char*)appearanceP, (const char*)&devP); 00678 00679 return BLE_ERROR_NONE; 00680 }
Generated on Sat Jul 16 2022 01:53:16 by
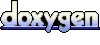