Fork of BlueNRG library to be compatible with bluetooth demo application
Dependents: Nucleo_BLE_Demo Nucleo_BLE_Demo
Fork of Nucleo_BLE_BlueNRG by
BlueNRGDevice.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "BlueNRGDevice.h" 00019 #include "BlueNRGGap.h" 00020 #include "BlueNRGGattServer.h" 00021 00022 #include "btle.h" 00023 #include "Utils.h" 00024 #include "osal.h" 00025 00026 /** 00027 * The singleton which represents the BlueNRG transport for the BLEDevice. 00028 */ 00029 static BlueNRGDevice deviceInstance; 00030 00031 /** 00032 * BLE-API requires an implementation of the following function in order to 00033 * obtain its transport handle. 00034 */ 00035 BLEDeviceInstanceBase * 00036 createBLEDeviceInstance(void) 00037 { 00038 return (&deviceInstance); 00039 } 00040 00041 /**************************************************************************/ 00042 /*! 00043 @brief Constructor 00044 */ 00045 /**************************************************************************/ 00046 BlueNRGDevice::BlueNRGDevice(void) 00047 { 00048 isInitialized = false; 00049 } 00050 00051 /**************************************************************************/ 00052 /*! 00053 @brief Destructor 00054 */ 00055 /**************************************************************************/ 00056 BlueNRGDevice::~BlueNRGDevice(void) 00057 { 00058 } 00059 00060 /**************************************************************************/ 00061 /*! 00062 @brief Initialises anything required to start using BLE 00063 00064 @returns ble_error_t 00065 00066 @retval BLE_ERROR_NONE 00067 Everything executed properly 00068 00069 @section EXAMPLE 00070 00071 @code 00072 00073 @endcode 00074 */ 00075 /**************************************************************************/ 00076 ble_error_t BlueNRGDevice::init(void) 00077 { 00078 /* ToDo: Clear memory contents, reset the SD, etc. */ 00079 btle_init(BlueNRGGap::getInstance().getIsSetAddress()); 00080 00081 isInitialized = true; 00082 00083 return BLE_ERROR_NONE; 00084 } 00085 00086 /**************************************************************************/ 00087 /*! 00088 @brief Resets the BLE HW, removing any existing services and 00089 characteristics 00090 00091 @returns ble_error_t 00092 00093 @retval BLE_ERROR_NONE 00094 Everything executed properly 00095 00096 @section EXAMPLE 00097 00098 @code 00099 00100 @endcode 00101 */ 00102 /**************************************************************************/ 00103 ble_error_t BlueNRGDevice::reset(void) 00104 { 00105 wait(0.5); 00106 00107 /* Reset BlueNRG SPI interface */ 00108 BlueNRG_RST(); 00109 00110 /* Wait for the radio to come back up */ 00111 wait(1); 00112 00113 isInitialized = false; 00114 00115 return BLE_ERROR_NONE; 00116 } 00117 00118 void BlueNRGDevice::waitForEvent(void) 00119 { 00120 HCI_Process();//Send App Events?? 00121 00122 } 00123 00124 00125 /**************************************************************************/ 00126 /*! 00127 @brief get GAP version 00128 00129 @returns char * 00130 00131 @retval pointer to version string 00132 00133 @section EXAMPLE 00134 00135 @code 00136 00137 @endcode 00138 */ 00139 /**************************************************************************/ 00140 const char *BlueNRGDevice::getVersion(void) 00141 { 00142 char *version = new char[6]; 00143 memcpy((void *)version, "1.0.0", 5); 00144 return version; 00145 } 00146 00147 /**************************************************************************/ 00148 /*! 00149 @brief get init state 00150 00151 @returns bool 00152 00153 @retval 00154 00155 @section EXAMPLE 00156 00157 @code 00158 00159 @endcode 00160 */ 00161 /**************************************************************************/ 00162 bool BlueNRGDevice::getIsInitialized(void) 00163 { 00164 return isInitialized; 00165 } 00166 00167 /**************************************************************************/ 00168 /*! 00169 @brief get reference to GAP object 00170 00171 @returns Gap& 00172 00173 @retval reference to gap object 00174 00175 @section EXAMPLE 00176 00177 @code 00178 00179 @endcode 00180 */ 00181 /**************************************************************************/ 00182 Gap &BlueNRGDevice::getGap() 00183 { 00184 return BlueNRGGap::getInstance(); 00185 } 00186 00187 /**************************************************************************/ 00188 /*! 00189 @brief get reference to GATT server object 00190 00191 @returns GattServer& 00192 00193 @retval reference to GATT server object 00194 00195 @section EXAMPLE 00196 00197 @code 00198 00199 @endcode 00200 */ 00201 /**************************************************************************/ 00202 GattServer &BlueNRGDevice::getGattServer() 00203 { 00204 return BlueNRGGattServer::getInstance(); 00205 } 00206 00207 /**************************************************************************/ 00208 /*! 00209 @brief set Tx power level 00210 00211 @returns ble_error_t 00212 00213 @retval BLE_ERROR_NONE 00214 Everything executed properly 00215 00216 @section EXAMPLE 00217 00218 @code 00219 00220 @endcode 00221 */ 00222 /**************************************************************************/ 00223 ble_error_t BlueNRGDevice::setTxPower(int8_t txPower) 00224 { 00225 int8_t enHighPower = 0; 00226 int8_t paLevel = 0; 00227 int8_t dbmActuallySet = getHighPowerAndPALevelValue(txPower, enHighPower, paLevel); 00228 DEBUG("txPower=%d, dbmActuallySet=%d\n\r", txPower, dbmActuallySet); 00229 DEBUG("enHighPower=%d, paLevel=%d\n\r", enHighPower, paLevel); 00230 aci_hal_set_tx_power_level(enHighPower, paLevel); 00231 return BLE_ERROR_NONE; 00232 }
Generated on Sat Jul 16 2022 01:53:16 by
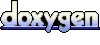