
Demo for Mbed Connect Cloud board and an MQTT Python Broker/Client
Embed:
(wiki syntax)
Show/hide line numbers
main_working.h
00001 //---------------------------------------------------------------------------- 00002 // The confidential and proprietary information contained in this file may 00003 // only be used by a person authorised under and to the extent permitted 00004 // by a subsisting licensing agreement from ARM Limited or its affiliates. 00005 // 00006 // (C) COPYRIGHT 2016 ARM Limited or its affiliates. 00007 // ALL RIGHTS RESERVED 00008 // 00009 // This entire notice must be reproduced on all copies of this file 00010 // and copies of this file may only be made by a person if such person is 00011 // permitted to do so under the terms of a subsisting license agreement 00012 // from ARM Limited or its affiliates. 00013 //---------------------------------------------------------------------------- 00014 #include "mbed.h" 00015 #include "C12832.h" 00016 #include "OdinWiFiInterface.h" 00017 #include "MQTTNetwork.h" 00018 #include "MQTTmbed.h" 00019 #include "MQTTClient.h" 00020 00021 // GLOBAL VARIABLES HERE 00022 00023 C12832 lcd(PE_14, PE_12, PD_12, PD_11, PE_9); 00024 OdinWiFiInterface wifi; 00025 InterruptIn button(PF_2); 00026 volatile bool publish = false; 00027 volatile int message_num = 0; 00028 00029 // FUNCTION DEFINITIONS HERE 00030 00031 void lcd_print(const char* message) { 00032 lcd.cls(); 00033 lcd.locate(0, 3); 00034 lcd.printf(message); 00035 } 00036 00037 void messageArrived(MQTT::MessageData& md) { 00038 MQTT::Message &message = md.message; 00039 char msg[300]; 00040 sprintf(msg, "Message arrived: QoS%d, retained %d, dup %d, packetID %d\r\n", message.qos, message.retained, message.dup, message.id); 00041 lcd_print(msg); 00042 wait_ms(1000); 00043 char payload[300]; 00044 sprintf(payload, "Payload %.*s\r\n", message.payloadlen, (char*)message.payload); 00045 lcd_print(payload); 00046 } 00047 00048 void publish_message() { 00049 publish = true; 00050 } 00051 00052 int main() { 00053 00054 // MAIN CODE HERE 00055 00056 lcd_print("Connecting..."); 00057 int ret = wifi.connect(MBED_CONF_APP_WIFI_SSID, MBED_CONF_APP_WIFI_PASSWORD, NSAPI_SECURITY_WPA_WPA2); 00058 if (ret != 0) { 00059 lcd_print("Connection error."); 00060 return -1; 00061 } 00062 00063 NetworkInterface* net = &wifi; 00064 MQTTNetwork mqttNetwork(net); 00065 MQTT::Client<MQTTNetwork, Countdown> client(mqttNetwork); 00066 00067 const char* host = "IP_ADDRESS_HERE"; 00068 const char* topic = "Mbed"; 00069 lcd_print("Connecting to MQTT network..."); 00070 int rc = mqttNetwork.connect(host, 1883); 00071 if (rc != 0) { 00072 lcd_print("Connection error."); 00073 return -1; 00074 } 00075 lcd_print("Successfully connected!"); 00076 00077 MQTTPacket_connectData data = MQTTPacket_connectData_initializer; 00078 data.MQTTVersion = 3; 00079 data.clientID.cstring = "connect-cloud-board"; 00080 client.connect(data); 00081 client.subscribe(topic, MQTT::QOS0, messageArrived); 00082 00083 button.fall(&publish_message); 00084 00085 while (true) { 00086 00087 // WHILE LOOP CODE HERE 00088 00089 if (publish) { 00090 message_num++; 00091 MQTT::Message message; 00092 char buff[100]; 00093 sprintf(buff, "QoS0 Hello, Python! #%d", message_num); 00094 message.qos = MQTT::QOS0; 00095 message.retained = false; 00096 message.dup = false; 00097 message.payload = (void*) buff; 00098 message.payloadlen = strlen(buff) + 1; 00099 rc = client.publish(topic, message); 00100 client.yield(100); 00101 publish = false; 00102 } 00103 00104 } 00105 00106 }
Generated on Tue Jul 12 2022 18:50:18 by
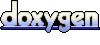