
Demo for Mbed Connect Cloud board and an MQTT Python Broker/Client
Embed:
(wiki syntax)
Show/hide line numbers
broker.py
00001 import paho.mqtt.client as paho 00002 import socket 00003 00004 # https://os.mbed.com/teams/mqtt/wiki/Using-MQTT#python-client 00005 00006 # MQTT broker hosted on local machine 00007 mqttc = paho.Client() 00008 00009 # Settings for connection 00010 host = "IP_ADDRESS_HERE" 00011 topic= "Mbed/#" 00012 00013 # Callbacks 00014 def on_connect(self, mosq, obj, rc): 00015 print("Connected rc: " + str(rc)) 00016 00017 def on_message(mosq, obj, msg): 00018 print("[Received] Topic: " + msg.topic + ", Message: " + str(msg.payload) + "\n"); 00019 00020 def on_subscribe(mosq, obj, mid, granted_qos): 00021 print("Subscribed OK") 00022 00023 def on_unsubscribe(mosq, obj, mid, granted_qos): 00024 print("Unsubscribed OK") 00025 00026 # Set callbacks 00027 mqttc.on_message = on_message 00028 mqttc.on_connect = on_connect 00029 mqttc.on_subscribe = on_subscribe 00030 mqttc.on_unsubscribe = on_unsubscribe 00031 00032 # Connect and subscribe 00033 print "Your IP address is:", socket.gethostbyname(socket.gethostname()) 00034 print("Connecting to " + host + "/" + topic) 00035 mqttc.connect(host, port=1883, keepalive=60) 00036 mqttc.subscribe(topic, 0) 00037 00038 # Loop forever, receiving messages 00039 mqttc.loop_forever() 00040 00041 print("rc: " + str(rc))
Generated on Tue Jul 12 2022 18:50:18 by
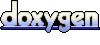