
Using DHT11 sensor, sdWrite, wdt. Need to sleep more then this.
Dependencies: DHT GPRSInterface HTTPClient_GPRS SDFileSystem USBDevice mbed
tcp_yeelink.cpp
00001 /* 00002 IOT_Mbed.cpp 00003 2013 Copyright (c) Seeed Technology Inc. All right reserved. 00004 00005 Author:Loovee 00006 2013-7-21 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00021 */ 00022 #include <stdio.h> 00023 00024 #include "mbed.h" 00025 #include "tcp_yeelink.h" 00026 #include "tcp_yeelink_dfs.h" 00027 #include "i2c_uart.h" 00028 #include "ARCH_GPRS_Sleep.h" 00029 00030 00031 Serial serial1(P0_19, P0_18); // tx, rx 00032 Timer tcnt; 00033 00034 void IOT_Mbed::init(char *postURL, char *APIKey) 00035 { 00036 serial1.baud(115200); 00037 strcpy(yeelinkPostURL, postURL); 00038 sprintf(yeelinkPostHeads, "U-ApiKey: %s\r\n", APIKey); 00039 strcpy(yeelinkDns, "42.96.164.52"); // api.yeelink.net 00040 sprintf(yeelinkPort, "%d", HTTP_DEFAULT_PORT); 00041 } 00042 00043 void IOT_Mbed::callTest() 00044 { 00045 sendCmdAndWaitForRest("ATD:10086;\r\n", "OK", 10); 00046 } 00047 00048 int IOT_Mbed::checkAT(int timeout) 00049 { 00050 return sendCmdAndWaitForRest("AT\r\n", "OK", timeout); 00051 } 00052 00053 int IOT_Mbed::waitString(const char *str, int timeout) // time out : s 00054 { 00055 int len = strlen(str); 00056 int sum=0; 00057 00058 tcnt.start(); // start timer 00059 00060 for(;;) 00061 { 00062 if(serial1.readable()) 00063 { 00064 char c = serial1.getc(); 00065 DBG(c); 00066 sum = (c==str[sum]) ? sum+1 : 0; 00067 if(sum == len)break; 00068 } 00069 00070 if(tcnt.read() > timeout) // time out 00071 { 00072 tcnt.stop(); 00073 tcnt.reset(); 00074 00075 DBG("time out\r\n"); 00076 return ERRTOUT; 00077 } 00078 00079 wdt_sleep.feed(); 00080 } 00081 00082 tcnt.stop(); // stop timer 00083 tcnt.reset(); // clear timer 00084 00085 while(serial1.readable()) // display the other thing.. 00086 { 00087 char c = serial1.getc(); 00088 DBG(c); 00089 } 00090 00091 return 1; 00092 } 00093 00094 00095 int IOT_Mbed::sendCmdAndWaitForRest(char *dta, const char *resq, int timeout) 00096 { 00097 sendCmd(dta); 00098 return waitString(resq, timeout); 00099 } 00100 00101 void IOT_Mbed::sendCmd(char *dta) 00102 { 00103 serial1.printf("%s\r\n", dta); 00104 } 00105 00106 int IOT_Mbed::connectTCP() 00107 { 00108 00109 sendCmdAndWaitForRest("ATE0\r\n", "OK", 3); 00110 int tout = 0; 00111 while(1) 00112 { 00113 if(sendCmdAndWaitForRest(STROPENGPRS, "OK", 20) == ERRTOUT) 00114 { 00115 DBG("GPRS OPEN ERR, OPEN AGAIN\r\n"); 00116 wait(5); 00117 } 00118 else 00119 { 00120 DBG("GPRS OPEN OK!\r\n"); 00121 break; 00122 } 00123 tout++; 00124 if(tout>5)return 0; 00125 } 00126 00127 00128 if(!sendCmdAndWaitForRest(STRSETGPRS, "OK", 20))return 0; 00129 if(!sendCmdAndWaitForRest(STRSETAPN, "OK", 20))return 0;; 00130 00131 char cipstart[50]; 00132 sprintf(cipstart, "AT+CIPSTART=\"TCP\",\"%s\",\"%s\"", yeelinkDns, yeelinkPort); 00133 if(!sendCmdAndWaitForRest(cipstart, "CONNECT OK", 20))return 0;; // connect tcp 00134 00135 return 1; 00136 } 00137 00138 int IOT_Mbed::connectTCP(char *ip, char *port) 00139 { 00140 00141 sendCmdAndWaitForRest("ATE0\r\n", "OK", 3); 00142 int tout = 0; 00143 00144 while(1) 00145 { 00146 if(sendCmdAndWaitForRest(STROPENGPRS, "OK", 20) == ERRTOUT) 00147 { 00148 DBG("GPRS OPEN ERR, OPEN AGAIN\r\n"); 00149 wait(5); 00150 } 00151 else 00152 { 00153 DBG("GPRS OPEN OK!\r\n"); 00154 break; 00155 } 00156 tout++; 00157 if(tout>5)return 0; 00158 } 00159 00160 00161 if(!sendCmdAndWaitForRest(STRSETGPRS, "OK", 20))return 0; 00162 if(!sendCmdAndWaitForRest(STRSETAPN, "OK", 20))return 0;; 00163 00164 char cipstart[50]; 00165 sprintf(cipstart, "AT+CIPSTART=\"TCP\",\"%s\",\"%s\"", ip, port); 00166 if(!sendCmdAndWaitForRest(cipstart, "CONNECT OK", 20))return 0;; // connect tcp 00167 return 1; 00168 00169 } 00170 00171 //send data to tcp 00172 int IOT_Mbed::sendDtaTcp(char *dta, int timeout) 00173 { 00174 serial1.printf("AT+CIPSEND=%d\r\n", strlen(dta)); 00175 waitString(">", 10); 00176 serial1.printf("%s", dta); 00177 00178 wait_ms(50); 00179 return waitString("SEND OK", timeout); 00180 } 00181 00182 bool IOT_Mbed::sendToYeelink_t() 00183 { 00184 char dtaSend[300]; 00185 sprintf(dtaSend, "%s\r\n%s\r\n%s\r\n%s\r\n%s\r\n%s\r\n\r\n%s\r\n", POST1, POST2, POST3, POST4, POST5, POST6, POST7); 00186 return sendDtaTcp(dtaSend, 20); 00187 } 00188 00189 void IOT_Mbed::postDtaToYeelink() 00190 { 00191 00192 } 00193 00194 int IOT_Mbed::postDtaToYeelink(char *url, char *apikey, int sensorDta) 00195 { 00196 return postDtaToYeelink(url, apikey, sensorDta, 0); 00197 } 00198 00199 int IOT_Mbed::postDtaToYeelink(char *url, char *apikey, float sensorDta, int dec) 00200 { 00201 char dtaPost[350]; 00202 00203 char request[100]; 00204 char heads[200]; 00205 char body[100]; 00206 00207 unsigned int port; 00208 00209 char host[HTTP_MAX_HOST_LEN]; 00210 char path[HTTP_MAX_PATH_LEN]; 00211 00212 if (parseURL(url, host, sizeof(host), &port, path, sizeof(path)) != 0) 00213 { 00214 DBG("Failed to parse URL.\r\n"); 00215 return 0; 00216 } 00217 00218 if(!connectTCP()) 00219 { 00220 DBG("connect to tcp err!\r\n"); 00221 return 0; 00222 } 00223 00224 if(dec == 0) 00225 { 00226 sprintf(body, "{\"value\": %.0f}\r\n", sensorDta); 00227 } 00228 else if(dec == 1) 00229 { 00230 sprintf(body, "{\"value\": %.1f}\r\n", sensorDta); 00231 } 00232 else if(dec == 2) 00233 { 00234 sprintf(body, "{\"value\": %.2f}\r\n", sensorDta); 00235 } 00236 else 00237 { 00238 sprintf(body, "{\"value\": %.3f}\r\n", sensorDta); 00239 00240 } 00241 sprintf(request, "POST %s HTTP/1.1\r\n", path); 00242 sprintf(heads, "Host: %s\r\nU-ApiKey: %s\r\nContent-Length: %d\r\nContent-Type: %s\r\n\r\n",host, apikey, strlen(body), CONTENT_TYPE); 00243 sprintf(dtaPost, "%s%s%s", request, heads, body); 00244 00245 sendDtaTcp(dtaPost, 10); 00246 00247 while(serial1.readable()) 00248 { 00249 char c = serial1.getc(); 00250 DBG(c); 00251 } 00252 00253 return sendCmdAndWaitForRest(STRCLOSE, "OK", 20); 00254 } 00255 00256 int IOT_Mbed::parseURL(const char *url, char *host, int max_host_len, unsigned int *port, char *path, int max_path_len) 00257 { 00258 char *scheme_ptr = (char *)url; 00259 char *host_ptr = (char *)strstr(url, "://"); 00260 if (host_ptr != NULL) 00261 { 00262 if (strncmp(scheme_ptr, "http://", 7)) 00263 { 00264 DBG("Bad scheme\r\n"); 00265 return -1; 00266 } 00267 host_ptr += 3; 00268 } 00269 else 00270 { 00271 host_ptr = (char *)url; 00272 } 00273 00274 int host_len = 0; 00275 char *port_ptr = strchr(host_ptr, ':'); 00276 00277 if (port_ptr != NULL) 00278 { 00279 host_len = port_ptr - host_ptr; 00280 port_ptr++; 00281 if (sscanf(port_ptr, "%hu", port) != 1) 00282 { 00283 DBG("Could not find port.\r\n"); 00284 return -3; 00285 } 00286 } 00287 else 00288 { 00289 *port = HTTP_DEFAULT_PORT; 00290 } 00291 00292 char *path_ptr = strchr(host_ptr, '/'); 00293 00294 if (host_len == 0) 00295 { 00296 host_len = path_ptr - host_ptr; 00297 } 00298 00299 if (max_host_len < (host_len + 1)) 00300 { 00301 DBG("Host buffer is too small.\r\n"); 00302 return -4; 00303 } 00304 00305 memcpy(host, host_ptr, host_len); 00306 host[host_len] = '\0'; 00307 00308 int path_len; 00309 00310 char *fragment_ptr = strchr(host_ptr, '#'); 00311 if (fragment_ptr != NULL) 00312 { 00313 path_len = fragment_ptr - path_ptr; 00314 } 00315 else 00316 { 00317 path_len = strlen(path_ptr); 00318 } 00319 00320 if (max_path_len < (path_len + 1)) 00321 { 00322 DBG("Path buffer is too small.\r\n"); 00323 return -5; 00324 } 00325 memcpy(path, path_ptr, path_len); 00326 path[path_len] = '\0'; 00327 00328 return 0; 00329 } 00330 00331 00332 00333 IOT_Mbed IOT; 00334 /********************************************************************************************************* 00335 END FILE 00336 *********************************************************************************************************/
Generated on Thu Jul 14 2022 23:53:26 by
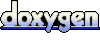