
NUCLEO-F042K6 Simple demo with buttons intterupt changing PWM DC - with autoincrement when button pressed longer than 1s
Dependencies: mbed
main.cpp
00001 #include "mbed.h" // import of mbed library (required) 00002 /******************************************************************************* 00003 00004 EXAMPLE DESCRIPTION 00005 00006 Uses two buttons connected to PA_0 - plus and PA_1 - minus with internal pull 00007 up resistors. On PA_8 is PWM output with variable duty cycle (DC) and frequncy 00008 of 500 Hz.DC can be changed true buttons. On press of button DC is incremented 00009 or decremented by 0.01. Interrupt is attached to falling edge on appropriate 00010 pin and process of incrementation/decrementation is driven throu this 00011 interrupt. Programm also demonstrates auto incrementation/decrementation while 00012 button is pressed for longer than 1 s. After button is pressed timer is 00013 started. Ticker periodically (every 10 ms) calls function that checks timer 00014 value. If the timer had counted more than 1 s, It is stopped and auto 00015 incementation/decrementation is stared (+-0.01 every 500 ms). After button 00016 release auto incementation/decrementation is stopped and timer reset. 00017 Programm is written in way, that there can NOT be simultaneously active both 00018 auto incrementation and decrementation. Note that there are also prints to 00019 UART to check states of buttons and change of DC. 00020 00021 *******************************************************************************/ 00022 00023 InterruptIn buttonPlus(PA_0); // deffition of interrupt 00024 InterruptIn buttonMinus(PA_1); // deffition of interrupt 00025 00026 PwmOut PWM(PA_8); // definition of PWM pin 00027 00028 Timer tim; // Timer definition 00029 00030 Ticker tick; // Ticker definition 00031 00032 bool autoIncrement=false; // true - do autoincrement 00033 // false - do not autoincrement 00034 00035 bool plus=true; // true - auto increment 00036 // false - auto decrement 00037 00038 // called after falling edge on PA_0 00039 // starts timer for auto increment check 00040 // increments DC 00041 void pressedPlus() { 00042 tim.start(); // start Timer 00043 plus=true; // incrementation 00044 if (PWM.read()+0.01 <= 1) { 00045 PWM.write(PWM.read()+0.01); 00046 printf("Presed plus button. DC: %f.\n",PWM.read()); 00047 }else{ 00048 PWM.write(1); 00049 printf("Presed plus button. Already maximum DC.\n"); 00050 } 00051 } 00052 00053 // called after rising edge on PA_0 00054 // stops and resets auto increment timer 00055 // stops auto increment 00056 void releasedPlus(){ 00057 tim.stop(); 00058 tim.reset(); 00059 autoIncrement=false; 00060 printf("Plus button released.\n"); 00061 } 00062 00063 // called after falling edge on PA_1 00064 // starts timer for auto decrement check 00065 // decrements DC 00066 void pressedMinus() { 00067 tim.start(); // start Timer 00068 plus=false; // decrementation 00069 if (PWM.read()-0.01 >= 0) { 00070 PWM.write(PWM.read()-0.01); 00071 printf("Presed minus button. DC: %f\n",PWM.read()); 00072 }else{ 00073 PWM.write(0); 00074 printf("Presed minus button. Already minimum DC.\n"); 00075 } 00076 } 00077 00078 // called after rising edge on PA_1 00079 // stops and resets auto decrement timer 00080 // stops auto decrement 00081 void releasedMinus(){ 00082 tim.stop(); 00083 tim.reset(); 00084 autoIncrement=false; 00085 printf("Minus button released.\n"); 00086 } 00087 00088 00089 // checks timer value 00090 // if > 1 s starts auto incerement/decrement 00091 void checkTimer(){ 00092 if(tim.read_ms()>1000){ 00093 tim.stop(); 00094 autoIncrement=true; 00095 } 00096 } 00097 00098 int main() 00099 { 00100 00101 // Set PWM 00102 PWM.period_ms(2); // 500 Hz 00103 PWM.write(0); // duration of active pulse 00104 00105 // Set buttons interrupts 00106 buttonPlus.fall(&pressedPlus); 00107 buttonMinus.fall(&pressedMinus); 00108 buttonPlus.rise(&releasedPlus); 00109 buttonMinus.rise(&releasedMinus); 00110 00111 // internal pull ups 00112 buttonPlus.mode(PullUp); 00113 buttonMinus.mode(PullUp); 00114 00115 // set ticker interrupt 00116 tick.attach(&checkTimer,0.01); 00117 00118 while (1) { 00119 // check if auto increment/decrement 00120 if(autoIncrement && plus && PWM.read()+0.01 <= 1){ 00121 PWM.write(PWM.read()+0.01); 00122 printf("Autoincrement. DC: %f.\n",PWM.read()); 00123 } 00124 else if(autoIncrement && !plus && PWM.read()-0.01 >= 0){ 00125 PWM.write(PWM.read()-0.01); 00126 printf("Autodecrement. DC: %f.\n",PWM.read()); 00127 } 00128 wait_ms(500); 00129 } 00130 }
Generated on Wed Jul 13 2022 09:54:17 by
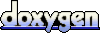