mouvement AX12 petit robot version 2
Fork of command_AX12_petit_robot by
Base.h
00001 /* mbed Microcontroller Library - Base 00002 * Copyright (c) 2006-2008 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_BASE_H 00006 #define MBED_BASE_H 00007 00008 #include "platform.h" 00009 #include "PinNames.h" 00010 #include "PeripheralNames.h" 00011 #include <cstdlib> 00012 #include "DirHandle.h" 00013 00014 namespace mbed { 00015 00016 #ifdef MBED_RPC 00017 struct rpc_function { 00018 const char *name; 00019 void (*caller)(const char*, char*); 00020 }; 00021 00022 struct rpc_class { 00023 const char *name; 00024 const rpc_function *static_functions; 00025 struct rpc_class *next; 00026 }; 00027 #endif 00028 00029 /* Class Base 00030 * The base class for most things 00031 */ 00032 class Base { 00033 00034 public: 00035 00036 Base(const char *name = NULL); 00037 00038 virtual ~Base(); 00039 00040 /* Function register_object 00041 * Registers this object with the given name, so that it can be 00042 * looked up with lookup. If this object has already been 00043 * registered, then this just changes the name. 00044 * 00045 * Variables 00046 * name - The name to give the object. If NULL we do nothing. 00047 */ 00048 void register_object(const char *name); 00049 00050 /* Function name 00051 * Returns the name of the object, or NULL if it has no name. 00052 */ 00053 const char *name(); 00054 00055 #ifdef MBED_RPC 00056 00057 /* Function rpc 00058 * Call the given method with the given arguments, and write the 00059 * result into the string pointed to by result. The default 00060 * implementation calls rpc_methods to determine the supported 00061 * methods. 00062 * 00063 * Variables 00064 * method - The name of the method to call. 00065 * arguments - A list of arguments separated by spaces. 00066 * result - A pointer to a string to write the result into. May 00067 * be NULL, in which case nothing is written. 00068 * 00069 * Returns 00070 * true if method corresponds to a valid rpc method, or 00071 * false otherwise. 00072 */ 00073 virtual bool rpc(const char *method, const char *arguments, char *result); 00074 00075 /* Function get_rpc_methods 00076 * Returns a pointer to an array describing the rpc methods 00077 * supported by this object, terminated by either 00078 * RPC_METHOD_END or RPC_METHOD_SUPER(Superclass). 00079 * 00080 * Example 00081 * > class Example : public Base { 00082 * > int foo(int a, int b) { return a + b; } 00083 * > virtual const struct rpc_method *get_rpc_methods() { 00084 * > static const rpc_method rpc_methods[] = { 00085 * > { "foo", generic_caller<int, Example, int, int, &Example::foo> }, 00086 * > RPC_METHOD_SUPER(Base) 00087 * > }; 00088 * > return rpc_methods; 00089 * > } 00090 * > }; 00091 */ 00092 virtual const struct rpc_method *get_rpc_methods(); 00093 00094 /* Function rpc 00095 * Use the lookup function to lookup an object and, if 00096 * successful, call its rpc method 00097 * 00098 * Variables 00099 * returns - false if name does not correspond to an object, 00100 * otherwise the return value of the call to the object's rpc 00101 * method. 00102 */ 00103 static bool rpc(const char *name, const char *method, const char *arguments, char *result); 00104 00105 #endif 00106 00107 /* Function lookup 00108 * Lookup and return the object that has the given name. 00109 * 00110 * Variables 00111 * name - the name to lookup. 00112 * len - the length of name. 00113 */ 00114 static Base *lookup(const char *name, unsigned int len); 00115 00116 static DirHandle *opendir(); 00117 friend class BaseDirHandle; 00118 00119 protected: 00120 00121 static Base *_head; 00122 Base *_next; 00123 const char *_name; 00124 bool _from_construct; 00125 00126 private: 00127 00128 #ifdef MBED_RPC 00129 static rpc_class *_classes; 00130 00131 static const rpc_function _base_funcs[]; 00132 static rpc_class _base_class; 00133 #endif 00134 00135 void delete_self(); 00136 static void list_objs(const char *arguments, char *result); 00137 static void clear(const char*,char*); 00138 00139 static char *new_name(Base *p); 00140 00141 public: 00142 00143 #ifdef MBED_RPC 00144 /* Function add_rpc_class 00145 * Add the class to the list of classes which can have static 00146 * methods called via rpc (the static methods which can be called 00147 * are defined by that class' get_rpc_class() static method). 00148 */ 00149 template<class C> 00150 static void add_rpc_class() { 00151 rpc_class *c = C::get_rpc_class(); 00152 c->next = _classes; 00153 _classes = c; 00154 } 00155 00156 template<class C> 00157 static const char *construct() { 00158 Base *p = new C(); 00159 p->_from_construct = true; 00160 if(p->_name==NULL) { 00161 p->register_object(new_name(p)); 00162 } 00163 return p->_name; 00164 } 00165 00166 template<class C, typename A1> 00167 static const char *construct(A1 arg1) { 00168 Base *p = new C(arg1); 00169 p->_from_construct = true; 00170 if(p->_name==NULL) { 00171 p->register_object(new_name(p)); 00172 } 00173 return p->_name; 00174 } 00175 00176 template<class C, typename A1, typename A2> 00177 static const char *construct(A1 arg1, A2 arg2) { 00178 Base *p = new C(arg1,arg2); 00179 p->_from_construct = true; 00180 if(p->_name==NULL) { 00181 p->register_object(new_name(p)); 00182 } 00183 return p->_name; 00184 } 00185 00186 template<class C, typename A1, typename A2, typename A3> 00187 static const char *construct(A1 arg1, A2 arg2, A3 arg3) { 00188 Base *p = new C(arg1,arg2,arg3); 00189 p->_from_construct = true; 00190 if(p->_name==NULL) { 00191 p->register_object(new_name(p)); 00192 } 00193 return p->_name; 00194 } 00195 00196 template<class C, typename A1, typename A2, typename A3, typename A4> 00197 static const char *construct(A1 arg1, A2 arg2, A3 arg3, A4 arg4) { 00198 Base *p = new C(arg1,arg2,arg3,arg4); 00199 p->_from_construct = true; 00200 if(p->_name==NULL) { 00201 p->register_object(new_name(p)); 00202 } 00203 return p->_name; 00204 } 00205 #endif 00206 00207 }; 00208 00209 /* Macro MBED_OBJECT_NAME_MAX 00210 * The maximum size of object name (including terminating null byte) 00211 * that will be recognised when using fopen to open a FileLike 00212 * object, or when using the rpc function. 00213 */ 00214 #define MBED_OBJECT_NAME_MAX 32 00215 00216 /* Macro MBED_METHOD_NAME_MAX 00217 * The maximum size of rpc method name (including terminating null 00218 * byte) that will be recognised by the rpc function (in rpc.h). 00219 */ 00220 #define MBED_METHOD_NAME_MAX 32 00221 00222 } // namespace mbed 00223 00224 #endif 00225
Generated on Tue Jul 12 2022 20:39:16 by
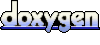