Strategie_13h30
Fork of CRAC-Strat_2017_homologation_gros_rob by
Embed:
(wiki syntax)
Show/hide line numbers
rtc_time.h
00001 /* Title: time 00002 * Implementation of the C time.h functions 00003 * 00004 * Provides mechanisms to set and read the current time, based 00005 * on the microcontroller Real-Time Clock (RTC), plus some 00006 * standard C manipulation and formating functions. 00007 * 00008 * Example: 00009 * > #include "mbed.h" 00010 * > 00011 * > int main() { 00012 * > set_time(1256729737); // Set RTC time to Wed, 28 Oct 2009 11:35:37 00013 * > 00014 * > while(1) { 00015 * > time_t seconds = time(NULL); 00016 * > 00017 * > printf("Time as seconds since January 1, 1970 = %d\n", seconds); 00018 * > 00019 * > printf("Time as a basic string = %s", ctime(&seconds)); 00020 * > 00021 * > char buffer[32]; 00022 * > strftime(buffer, 32, "%I:%M %p\n", localtime(&seconds)); 00023 * > printf("Time as a custom formatted string = %s", buffer); 00024 * > 00025 * > wait(1); 00026 * > } 00027 * > } 00028 */ 00029 00030 /* mbed Microcontroller Library - rtc_time 00031 * Copyright (c) 2009 ARM Limited. All rights reserved. 00032 */ 00033 00034 #include <time.h> 00035 00036 #ifdef __cplusplus 00037 extern "C" { 00038 #endif 00039 00040 #if 0 // for documentation only 00041 /* Function: time 00042 * Get the current time 00043 * 00044 * Returns the current timestamp as the number of seconds since January 1, 1970 00045 * (the UNIX timestamp). The value is based on the current value of the 00046 * microcontroller Real-Time Clock (RTC), which can be set using <set_time>. 00047 * 00048 * Example: 00049 * > #include "mbed.h" 00050 * > 00051 * > int main() { 00052 * > time_t seconds = time(NULL); 00053 * > printf("It is %d seconds since January 1, 1970\n", seconds); 00054 * > } 00055 * 00056 * Variables: 00057 * t - Pointer to a time_t to be set, or NULL if not used 00058 * returns - Number of seconds since January 1, 1970 (the UNIX timestamp) 00059 */ 00060 time_t time(time_t *t); 00061 #endif 00062 00063 /* Function: set_time 00064 * Set the current time 00065 * 00066 * Initialises and sets the time of the microcontroller Real-Time Clock (RTC) 00067 * to the time represented by the number of seconds since January 1, 1970 00068 * (the UNIX timestamp). 00069 * 00070 * Example: 00071 * > #include "mbed.h" 00072 * > 00073 * > int main() { 00074 * > set_time(1256729737); // Set time to Wed, 28 Oct 2009 11:35:37 00075 * > } 00076 * 00077 * Variables: 00078 * t - Number of seconds since January 1, 1970 (the UNIX timestamp) 00079 */ 00080 void set_time(time_t t); 00081 00082 #if 0 // for documentation only 00083 /* Function: mktime 00084 * Converts a tm structure in to a timestamp 00085 * 00086 * Converts the tm structure in to a timestamp in seconds since January 1, 1970 00087 * (the UNIX timestamp). The values of tm_wday and tm_yday of the tm structure 00088 * are also updated to their appropriate values. 00089 * 00090 * Example: 00091 * > #include "mbed.h" 00092 * > 00093 * > int main() { 00094 * > // setup time structure for Wed, 28 Oct 2009 11:35:37 00095 * > struct tm t; 00096 * > t.tm_sec = 37; // 0-59 00097 * > t.tm_min = 35; // 0-59 00098 * > t.tm_hour = 11; // 0-23 00099 * > t.tm_mday = 28; // 1-31 00100 * > t.tm_mon = 9; // 0-11 00101 * > t.tm_year = 109; // year since 1900 00102 * > 00103 * > // convert to timestamp and display (1256729737) 00104 * > time_t seconds = mktime(&t); 00105 * > printf("Time as seconds since January 1, 1970 = %d\n", seconds); 00106 * > } 00107 * 00108 * Variables: 00109 * t - The tm structure to convert 00110 * returns - The converted timestamp 00111 */ 00112 time_t mktime(struct tm *t); 00113 #endif 00114 00115 #if 0 // for documentation only 00116 /* Function: localtime 00117 * Converts a timestamp in to a tm structure 00118 * 00119 * Converts the timestamp pointed to by t to a (statically allocated) 00120 * tm structure. 00121 * 00122 * Example: 00123 * > #include "mbed.h" 00124 * > 00125 * > int main() { 00126 * > time_t seconds = 1256729737; 00127 * > struct tm *t = localtime(&seconds); 00128 * > } 00129 * 00130 * Variables: 00131 * t - Pointer to the timestamp 00132 * returns - Pointer to the (statically allocated) tm structure 00133 */ 00134 struct tm *localtime(const time_t *t); 00135 #endif 00136 00137 #if 0 // for documentation only 00138 /* Function: ctime 00139 * Converts a timestamp to a human-readable string 00140 * 00141 * Converts a time_t timestamp in seconds since January 1, 1970 (the UNIX 00142 * timestamp) to a human readable string format. The result is of the 00143 * format: "Wed Oct 28 11:35:37 2009\n" 00144 * 00145 * Example: 00146 * > #include "mbed.h" 00147 * > 00148 * > int main() { 00149 * > time_t seconds = time(NULL); 00150 * > printf("Time as a string = %s", ctime(&seconds)); 00151 * > } 00152 * 00153 * Variables: 00154 * t - The timestamp to convert 00155 * returns - Pointer to a (statically allocated) string containing the 00156 * human readable representation, including a '\n' character 00157 */ 00158 char *ctime(const time_t *t); 00159 #endif 00160 00161 #if 0 // for documentation only 00162 /* Function: strftime 00163 * Converts a tm structure to a custom format human-readable string 00164 * 00165 * Creates a formated string from a tm structure, based on a string format 00166 * specifier provided. 00167 * 00168 * Format Specifiers: 00169 * %S - Second (00-59) 00170 * %M - Minute (00-59) 00171 * %H - Hour (00-23) 00172 * %d - Day (01-31) 00173 * %m - Month (01-12) 00174 * %Y/%y - Year (2009/09) 00175 * 00176 * %A/%a - Weekday Name (Monday/Mon) 00177 * %B/%b - Month Name (January/Jan) 00178 * %I - 12 Hour Format (01-12) 00179 * %p - "AM" or "PM" 00180 * %X - Time (14:55:02) 00181 * %x - Date (08/23/01) 00182 * 00183 * Example: 00184 * > #include "mbed.h" 00185 * > 00186 * > int main() { 00187 * > time_t seconds = time(NULL); 00188 * > 00189 * > char buffer[32]; 00190 * > strftime(buffer, 32, "%I:%M %p\n", localtime(&seconds)); 00191 * > printf("Time as a formatted string = %s", buffer); 00192 * > } 00193 * 00194 * Variables: 00195 * buffer - String buffer to store the result 00196 * max - Maximum number of characters to store in the buffer 00197 * format - Format specifier string 00198 * t - Pointer to the tm structure to convert 00199 * returns - Number of characters copied 00200 */ 00201 size_t strftime(char *buffer, size_t max, const char *format, const struct tm *t); 00202 #endif 00203 00204 #ifdef __cplusplus 00205 } 00206 #endif
Generated on Tue Jul 12 2022 19:28:48 by
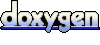