Strategie_13h30
Fork of CRAC-Strat_2017_homologation_gros_rob by
Embed:
(wiki syntax)
Show/hide line numbers
core_cmFunc.h
Go to the documentation of this file.
00001 /**************************************************************************//** 00002 * @file core_cmFunc.h 00003 * @brief CMSIS Cortex-M Core Function Access Header File 00004 * @version V3.00 00005 * @date 09. December 2011 00006 * 00007 * @note 00008 * Copyright (C) 2009-2011 ARM Limited. All rights reserved. 00009 * 00010 * @par 00011 * ARM Limited (ARM) is supplying this software for use with Cortex-M 00012 * processor based microcontrollers. This file can be freely distributed 00013 * within development tools that are supporting such ARM based processors. 00014 * 00015 * @par 00016 * THIS SOFTWARE IS PROVIDED "AS IS". NO WARRANTIES, WHETHER EXPRESS, IMPLIED 00017 * OR STATUTORY, INCLUDING, BUT NOT LIMITED TO, IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. 00019 * ARM SHALL NOT, IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL, OR 00020 * CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER. 00021 * 00022 ******************************************************************************/ 00023 00024 #ifndef __CORE_CMFUNC_H 00025 #define __CORE_CMFUNC_H 00026 00027 00028 /* ########################### Core Function Access ########################### */ 00029 /** \ingroup CMSIS_Core_FunctionInterface 00030 \defgroup CMSIS_Core_RegAccFunctions CMSIS Core Register Access Functions 00031 @{ 00032 */ 00033 00034 #if defined ( __CC_ARM ) /*------------------RealView Compiler -----------------*/ 00035 /* ARM armcc specific functions */ 00036 00037 #if (__ARMCC_VERSION < 400677) 00038 #error "Please use ARM Compiler Toolchain V4.0.677 or later!" 00039 #endif 00040 00041 /* intrinsic void __enable_irq(); */ 00042 /* intrinsic void __disable_irq(); */ 00043 00044 /** \brief Get Control Register 00045 00046 This function returns the content of the Control Register. 00047 00048 \return Control Register value 00049 */ 00050 static __INLINE uint32_t __get_CONTROL(void) 00051 { 00052 register uint32_t __regControl __ASM("control"); 00053 return(__regControl); 00054 } 00055 00056 00057 /** \brief Set Control Register 00058 00059 This function writes the given value to the Control Register. 00060 00061 \param [in] control Control Register value to set 00062 */ 00063 static __INLINE void __set_CONTROL(uint32_t control) 00064 { 00065 register uint32_t __regControl __ASM("control"); 00066 __regControl = control; 00067 } 00068 00069 00070 /** \brief Get IPSR Register 00071 00072 This function returns the content of the IPSR Register. 00073 00074 \return IPSR Register value 00075 */ 00076 static __INLINE uint32_t __get_IPSR(void) 00077 { 00078 register uint32_t __regIPSR __ASM("ipsr"); 00079 return(__regIPSR); 00080 } 00081 00082 00083 /** \brief Get APSR Register 00084 00085 This function returns the content of the APSR Register. 00086 00087 \return APSR Register value 00088 */ 00089 static __INLINE uint32_t __get_APSR(void) 00090 { 00091 register uint32_t __regAPSR __ASM("apsr"); 00092 return(__regAPSR); 00093 } 00094 00095 00096 /** \brief Get xPSR Register 00097 00098 This function returns the content of the xPSR Register. 00099 00100 \return xPSR Register value 00101 */ 00102 static __INLINE uint32_t __get_xPSR(void) 00103 { 00104 register uint32_t __regXPSR __ASM("xpsr"); 00105 return(__regXPSR); 00106 } 00107 00108 00109 /** \brief Get Process Stack Pointer 00110 00111 This function returns the current value of the Process Stack Pointer (PSP). 00112 00113 \return PSP Register value 00114 */ 00115 static __INLINE uint32_t __get_PSP(void) 00116 { 00117 register uint32_t __regProcessStackPointer __ASM("psp"); 00118 return(__regProcessStackPointer); 00119 } 00120 00121 00122 /** \brief Set Process Stack Pointer 00123 00124 This function assigns the given value to the Process Stack Pointer (PSP). 00125 00126 \param [in] topOfProcStack Process Stack Pointer value to set 00127 */ 00128 static __INLINE void __set_PSP(uint32_t topOfProcStack) 00129 { 00130 register uint32_t __regProcessStackPointer __ASM("psp"); 00131 __regProcessStackPointer = topOfProcStack; 00132 } 00133 00134 00135 /** \brief Get Main Stack Pointer 00136 00137 This function returns the current value of the Main Stack Pointer (MSP). 00138 00139 \return MSP Register value 00140 */ 00141 static __INLINE uint32_t __get_MSP(void) 00142 { 00143 register uint32_t __regMainStackPointer __ASM("msp"); 00144 return(__regMainStackPointer); 00145 } 00146 00147 00148 /** \brief Set Main Stack Pointer 00149 00150 This function assigns the given value to the Main Stack Pointer (MSP). 00151 00152 \param [in] topOfMainStack Main Stack Pointer value to set 00153 */ 00154 static __INLINE void __set_MSP(uint32_t topOfMainStack) 00155 { 00156 register uint32_t __regMainStackPointer __ASM("msp"); 00157 __regMainStackPointer = topOfMainStack; 00158 } 00159 00160 00161 /** \brief Get Priority Mask 00162 00163 This function returns the current state of the priority mask bit from the Priority Mask Register. 00164 00165 \return Priority Mask value 00166 */ 00167 static __INLINE uint32_t __get_PRIMASK(void) 00168 { 00169 register uint32_t __regPriMask __ASM("primask"); 00170 return(__regPriMask); 00171 } 00172 00173 00174 /** \brief Set Priority Mask 00175 00176 This function assigns the given value to the Priority Mask Register. 00177 00178 \param [in] priMask Priority Mask 00179 */ 00180 static __INLINE void __set_PRIMASK(uint32_t priMask) 00181 { 00182 register uint32_t __regPriMask __ASM("primask"); 00183 __regPriMask = (priMask); 00184 } 00185 00186 00187 #if (__CORTEX_M >= 0x03) 00188 00189 /** \brief Enable FIQ 00190 00191 This function enables FIQ interrupts by clearing the F-bit in the CPSR. 00192 Can only be executed in Privileged modes. 00193 */ 00194 #define __enable_fault_irq __enable_fiq 00195 00196 00197 /** \brief Disable FIQ 00198 00199 This function disables FIQ interrupts by setting the F-bit in the CPSR. 00200 Can only be executed in Privileged modes. 00201 */ 00202 #define __disable_fault_irq __disable_fiq 00203 00204 00205 /** \brief Get Base Priority 00206 00207 This function returns the current value of the Base Priority register. 00208 00209 \return Base Priority register value 00210 */ 00211 static __INLINE uint32_t __get_BASEPRI(void) 00212 { 00213 register uint32_t __regBasePri __ASM("basepri"); 00214 return(__regBasePri); 00215 } 00216 00217 00218 /** \brief Set Base Priority 00219 00220 This function assigns the given value to the Base Priority register. 00221 00222 \param [in] basePri Base Priority value to set 00223 */ 00224 static __INLINE void __set_BASEPRI(uint32_t basePri) 00225 { 00226 register uint32_t __regBasePri __ASM("basepri"); 00227 __regBasePri = (basePri & 0xff); 00228 } 00229 00230 00231 /** \brief Get Fault Mask 00232 00233 This function returns the current value of the Fault Mask register. 00234 00235 \return Fault Mask register value 00236 */ 00237 static __INLINE uint32_t __get_FAULTMASK(void) 00238 { 00239 register uint32_t __regFaultMask __ASM("faultmask"); 00240 return(__regFaultMask); 00241 } 00242 00243 00244 /** \brief Set Fault Mask 00245 00246 This function assigns the given value to the Fault Mask register. 00247 00248 \param [in] faultMask Fault Mask value to set 00249 */ 00250 static __INLINE void __set_FAULTMASK(uint32_t faultMask) 00251 { 00252 register uint32_t __regFaultMask __ASM("faultmask"); 00253 __regFaultMask = (faultMask & (uint32_t)1); 00254 } 00255 00256 #endif /* (__CORTEX_M >= 0x03) */ 00257 00258 00259 #if (__CORTEX_M == 0x04) 00260 00261 /** \brief Get FPSCR 00262 00263 This function returns the current value of the Floating Point Status/Control register. 00264 00265 \return Floating Point Status/Control register value 00266 */ 00267 static __INLINE uint32_t __get_FPSCR(void) 00268 { 00269 #if (__FPU_PRESENT == 1) && (__FPU_USED == 1) 00270 register uint32_t __regfpscr __ASM("fpscr"); 00271 return(__regfpscr); 00272 #else 00273 return(0); 00274 #endif 00275 } 00276 00277 00278 /** \brief Set FPSCR 00279 00280 This function assigns the given value to the Floating Point Status/Control register. 00281 00282 \param [in] fpscr Floating Point Status/Control value to set 00283 */ 00284 static __INLINE void __set_FPSCR(uint32_t fpscr) 00285 { 00286 #if (__FPU_PRESENT == 1) && (__FPU_USED == 1) 00287 register uint32_t __regfpscr __ASM("fpscr"); 00288 __regfpscr = (fpscr); 00289 #endif 00290 } 00291 00292 #endif /* (__CORTEX_M == 0x04) */ 00293 00294 00295 #elif defined ( __ICCARM__ ) /*------------------ ICC Compiler -------------------*/ 00296 /* IAR iccarm specific functions */ 00297 00298 #include <cmsis_iar.h> 00299 00300 #elif defined ( __GNUC__ ) /*------------------ GNU Compiler ---------------------*/ 00301 /* GNU gcc specific functions */ 00302 00303 /** \brief Enable IRQ Interrupts 00304 00305 This function enables IRQ interrupts by clearing the I-bit in the CPSR. 00306 Can only be executed in Privileged modes. 00307 */ 00308 __attribute__( ( always_inline ) ) static __INLINE void __enable_irq(void) 00309 { 00310 __ASM volatile ("cpsie i"); 00311 } 00312 00313 00314 /** \brief Disable IRQ Interrupts 00315 00316 This function disables IRQ interrupts by setting the I-bit in the CPSR. 00317 Can only be executed in Privileged modes. 00318 */ 00319 __attribute__( ( always_inline ) ) static __INLINE void __disable_irq(void) 00320 { 00321 __ASM volatile ("cpsid i"); 00322 } 00323 00324 00325 /** \brief Get Control Register 00326 00327 This function returns the content of the Control Register. 00328 00329 \return Control Register value 00330 */ 00331 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_CONTROL(void) 00332 { 00333 uint32_t result; 00334 00335 __ASM volatile ("MRS %0, control" : "=r" (result) ); 00336 return(result); 00337 } 00338 00339 00340 /** \brief Set Control Register 00341 00342 This function writes the given value to the Control Register. 00343 00344 \param [in] control Control Register value to set 00345 */ 00346 __attribute__( ( always_inline ) ) static __INLINE void __set_CONTROL(uint32_t control) 00347 { 00348 __ASM volatile ("MSR control, %0" : : "r" (control) ); 00349 } 00350 00351 00352 /** \brief Get IPSR Register 00353 00354 This function returns the content of the IPSR Register. 00355 00356 \return IPSR Register value 00357 */ 00358 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_IPSR(void) 00359 { 00360 uint32_t result; 00361 00362 __ASM volatile ("MRS %0, ipsr" : "=r" (result) ); 00363 return(result); 00364 } 00365 00366 00367 /** \brief Get APSR Register 00368 00369 This function returns the content of the APSR Register. 00370 00371 \return APSR Register value 00372 */ 00373 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_APSR(void) 00374 { 00375 uint32_t result; 00376 00377 __ASM volatile ("MRS %0, apsr" : "=r" (result) ); 00378 return(result); 00379 } 00380 00381 00382 /** \brief Get xPSR Register 00383 00384 This function returns the content of the xPSR Register. 00385 00386 \return xPSR Register value 00387 */ 00388 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_xPSR(void) 00389 { 00390 uint32_t result; 00391 00392 __ASM volatile ("MRS %0, xpsr" : "=r" (result) ); 00393 return(result); 00394 } 00395 00396 00397 /** \brief Get Process Stack Pointer 00398 00399 This function returns the current value of the Process Stack Pointer (PSP). 00400 00401 \return PSP Register value 00402 */ 00403 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_PSP(void) 00404 { 00405 register uint32_t result; 00406 00407 __ASM volatile ("MRS %0, psp\n" : "=r" (result) ); 00408 return(result); 00409 } 00410 00411 00412 /** \brief Set Process Stack Pointer 00413 00414 This function assigns the given value to the Process Stack Pointer (PSP). 00415 00416 \param [in] topOfProcStack Process Stack Pointer value to set 00417 */ 00418 __attribute__( ( always_inline ) ) static __INLINE void __set_PSP(uint32_t topOfProcStack) 00419 { 00420 __ASM volatile ("MSR psp, %0\n" : : "r" (topOfProcStack) ); 00421 } 00422 00423 00424 /** \brief Get Main Stack Pointer 00425 00426 This function returns the current value of the Main Stack Pointer (MSP). 00427 00428 \return MSP Register value 00429 */ 00430 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_MSP(void) 00431 { 00432 register uint32_t result; 00433 00434 __ASM volatile ("MRS %0, msp\n" : "=r" (result) ); 00435 return(result); 00436 } 00437 00438 00439 /** \brief Set Main Stack Pointer 00440 00441 This function assigns the given value to the Main Stack Pointer (MSP). 00442 00443 \param [in] topOfMainStack Main Stack Pointer value to set 00444 */ 00445 __attribute__( ( always_inline ) ) static __INLINE void __set_MSP(uint32_t topOfMainStack) 00446 { 00447 __ASM volatile ("MSR msp, %0\n" : : "r" (topOfMainStack) ); 00448 } 00449 00450 00451 /** \brief Get Priority Mask 00452 00453 This function returns the current state of the priority mask bit from the Priority Mask Register. 00454 00455 \return Priority Mask value 00456 */ 00457 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_PRIMASK(void) 00458 { 00459 uint32_t result; 00460 00461 __ASM volatile ("MRS %0, primask" : "=r" (result) ); 00462 return(result); 00463 } 00464 00465 00466 /** \brief Set Priority Mask 00467 00468 This function assigns the given value to the Priority Mask Register. 00469 00470 \param [in] priMask Priority Mask 00471 */ 00472 __attribute__( ( always_inline ) ) static __INLINE void __set_PRIMASK(uint32_t priMask) 00473 { 00474 __ASM volatile ("MSR primask, %0" : : "r" (priMask) ); 00475 } 00476 00477 00478 #if (__CORTEX_M >= 0x03) 00479 00480 /** \brief Enable FIQ 00481 00482 This function enables FIQ interrupts by clearing the F-bit in the CPSR. 00483 Can only be executed in Privileged modes. 00484 */ 00485 __attribute__( ( always_inline ) ) static __INLINE void __enable_fault_irq(void) 00486 { 00487 __ASM volatile ("cpsie f"); 00488 } 00489 00490 00491 /** \brief Disable FIQ 00492 00493 This function disables FIQ interrupts by setting the F-bit in the CPSR. 00494 Can only be executed in Privileged modes. 00495 */ 00496 __attribute__( ( always_inline ) ) static __INLINE void __disable_fault_irq(void) 00497 { 00498 __ASM volatile ("cpsid f"); 00499 } 00500 00501 00502 /** \brief Get Base Priority 00503 00504 This function returns the current value of the Base Priority register. 00505 00506 \return Base Priority register value 00507 */ 00508 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_BASEPRI(void) 00509 { 00510 uint32_t result; 00511 00512 __ASM volatile ("MRS %0, basepri_max" : "=r" (result) ); 00513 return(result); 00514 } 00515 00516 00517 /** \brief Set Base Priority 00518 00519 This function assigns the given value to the Base Priority register. 00520 00521 \param [in] basePri Base Priority value to set 00522 */ 00523 __attribute__( ( always_inline ) ) static __INLINE void __set_BASEPRI(uint32_t value) 00524 { 00525 __ASM volatile ("MSR basepri, %0" : : "r" (value) ); 00526 } 00527 00528 00529 /** \brief Get Fault Mask 00530 00531 This function returns the current value of the Fault Mask register. 00532 00533 \return Fault Mask register value 00534 */ 00535 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_FAULTMASK(void) 00536 { 00537 uint32_t result; 00538 00539 __ASM volatile ("MRS %0, faultmask" : "=r" (result) ); 00540 return(result); 00541 } 00542 00543 00544 /** \brief Set Fault Mask 00545 00546 This function assigns the given value to the Fault Mask register. 00547 00548 \param [in] faultMask Fault Mask value to set 00549 */ 00550 __attribute__( ( always_inline ) ) static __INLINE void __set_FAULTMASK(uint32_t faultMask) 00551 { 00552 __ASM volatile ("MSR faultmask, %0" : : "r" (faultMask) ); 00553 } 00554 00555 #endif /* (__CORTEX_M >= 0x03) */ 00556 00557 00558 #if (__CORTEX_M == 0x04) 00559 00560 /** \brief Get FPSCR 00561 00562 This function returns the current value of the Floating Point Status/Control register. 00563 00564 \return Floating Point Status/Control register value 00565 */ 00566 __attribute__( ( always_inline ) ) static __INLINE uint32_t __get_FPSCR(void) 00567 { 00568 #if (__FPU_PRESENT == 1) && (__FPU_USED == 1) 00569 uint32_t result; 00570 00571 __ASM volatile ("VMRS %0, fpscr" : "=r" (result) ); 00572 return(result); 00573 #else 00574 return(0); 00575 #endif 00576 } 00577 00578 00579 /** \brief Set FPSCR 00580 00581 This function assigns the given value to the Floating Point Status/Control register. 00582 00583 \param [in] fpscr Floating Point Status/Control value to set 00584 */ 00585 __attribute__( ( always_inline ) ) static __INLINE void __set_FPSCR(uint32_t fpscr) 00586 { 00587 #if (__FPU_PRESENT == 1) && (__FPU_USED == 1) 00588 __ASM volatile ("VMSR fpscr, %0" : : "r" (fpscr) ); 00589 #endif 00590 } 00591 00592 #endif /* (__CORTEX_M == 0x04) */ 00593 00594 00595 #elif defined ( __TASKING__ ) /*------------------ TASKING Compiler --------------*/ 00596 /* TASKING carm specific functions */ 00597 00598 /* 00599 * The CMSIS functions have been implemented as intrinsics in the compiler. 00600 * Please use "carm -?i" to get an up to date list of all instrinsics, 00601 * Including the CMSIS ones. 00602 */ 00603 00604 #endif 00605 00606 /*@} end of CMSIS_Core_RegAccFunctions */ 00607 00608 00609 #endif /* __CORE_CMFUNC_H */
Generated on Tue Jul 12 2022 19:28:47 by
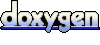