Strategie_13h30
Fork of CRAC-Strat_2017_homologation_gros_rob by
Embed:
(wiki syntax)
Show/hide line numbers
Strategie_big.cpp
00001 00002 #include "StrategieManager.h" 00003 #ifdef ROBOT_BIG 00004 #include "Config_big.h" 00005 00006 unsigned char isStopEnable = 1;//Permet de savoir si il faut autoriser le stop via les balises 00007 unsigned short telemetreDistance; 00008 /****************************************************************************************/ 00009 /* FUNCTION NAME: doFunnyAction */ 00010 /* DESCRIPTION : Permet de faire la funny action en fin de partie */ 00011 /****************************************************************************************/ 00012 void doFunnyAction(void) { 00013 //envoie de la funny action 00014 // 0x007, 01, 01 00015 CANMessage msgTx=CANMessage(); 00016 msgTx.id=GLOBAL_FUNNY_ACTION; 00017 msgTx.format=CANStandard; 00018 msgTx.type=CANData; 00019 msgTx.len=2; 00020 msgTx.data[0]=0x01; 00021 msgTx.data[1]=0x01; 00022 can1.write(msgTx); 00023 } 00024 00025 /****************************************************************************************/ 00026 /* FUNCTION NAME: doAction */ 00027 /* DESCRIPTION : Effectuer une action specifique */ 00028 /****************************************************************************************/ 00029 unsigned char doAction(unsigned char id, unsigned short speed, short angle) { 00030 CANMessage msgTx=CANMessage(); 00031 int localData = 0, localData2; 00032 switch(id) { 00033 case 100: // position initiale 00034 msgTx.id=SERVO_AX12_ACTION; 00035 msgTx.format=CANStandard; 00036 msgTx.type=CANData; 00037 msgTx.len=2; 00038 00039 if (InversStrat){ // si on est inversé, on echange les bras 00040 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00041 else speed = BRAS_AVANT; 00042 } 00043 00044 msgTx.data[0]=1; 00045 msgTx.data[1]=speed; 00046 can1.write(msgTx); 00047 break; 00048 00049 case 101: /// preparation prise 00050 msgTx.id=SERVO_AX12_ACTION; 00051 msgTx.format=CANStandard; 00052 msgTx.type=CANData; 00053 msgTx.len=2; 00054 00055 if (InversStrat){ // si on est inversé, on echange les bras 00056 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00057 else speed = BRAS_AVANT; 00058 } 00059 00060 msgTx.data[0]=2; 00061 msgTx.data[1]=speed; 00062 can1.write(msgTx); 00063 break; 00064 00065 case 102: // stockage haut 00066 msgTx.id=SERVO_AX12_ACTION; 00067 msgTx.format=CANStandard; 00068 msgTx.type=CANData; 00069 msgTx.len=2; 00070 00071 if (InversStrat){ // si on est inversé, on echange les bras 00072 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00073 else speed = BRAS_AVANT; 00074 } 00075 00076 msgTx.data[0]=3; 00077 msgTx.data[1]=speed; 00078 can1.write(msgTx); 00079 break; 00080 00081 case 103: // stockage bas 00082 msgTx.id=SERVO_AX12_ACTION; 00083 msgTx.format=CANStandard; 00084 msgTx.type=CANData; 00085 msgTx.len=2; 00086 00087 if (InversStrat){ // si on est inversé, on echange les bras 00088 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00089 else speed = BRAS_AVANT; 00090 } 00091 00092 msgTx.data[0]=4; 00093 msgTx.data[1]=speed; 00094 can1.write(msgTx); 00095 break; 00096 00097 case 104: // pousser module 00098 msgTx.id=SERVO_AX12_ACTION; 00099 msgTx.format=CANStandard; 00100 msgTx.type=CANData; 00101 msgTx.len=2; 00102 00103 if (InversStrat){ // si on est inversé, on echange les bras 00104 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00105 else speed = BRAS_AVANT; 00106 } 00107 00108 msgTx.data[0]=7; 00109 msgTx.data[1]=speed; 00110 can1.write(msgTx); 00111 break; 00112 00113 case 105: // preparation prise 00114 msgTx.id=SERVO_AX12_ACTION; 00115 msgTx.format=CANStandard; 00116 msgTx.type=CANData; 00117 msgTx.len=2; 00118 00119 if (InversStrat){ // si on est inversé, on echange les bras 00120 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00121 else speed = BRAS_AVANT; 00122 } 00123 00124 msgTx.data[0]=6; 00125 msgTx.data[1]=speed; 00126 can1.write(msgTx); 00127 break; 00128 00129 case 106: //deposer module 00130 msgTx.id=SERVO_AX12_ACTION; 00131 msgTx.format=CANStandard; 00132 msgTx.type=CANData; 00133 msgTx.len=2; 00134 00135 if (InversStrat){ // si on est inversé, on echange les bras 00136 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00137 else speed = BRAS_AVANT; 00138 } 00139 00140 msgTx.data[0]=5; 00141 msgTx.data[1]=speed; 00142 can1.write(msgTx); 00143 break; 00144 00145 case 110: // repos bras avant 00146 msgTx.id=SERVO_AX12_ACTION; 00147 msgTx.format=CANStandard; 00148 msgTx.type=CANData; 00149 msgTx.len=2; 00150 speed = BRAS_AVANT; 00151 if (InversStrat){ // si on est inversé, on echange les bras 00152 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00153 else speed = BRAS_AVANT; 00154 } 00155 if (speed == BRAS_AVANT)localData = 0x10; 00156 else localData = 0x24; 00157 00158 msgTx.data[0]=localData; 00159 msgTx.data[1]=speed; 00160 can1.write(msgTx); 00161 break; 00162 00163 case 111: // init bras module 00164 msgTx.id=SERVO_AX12_ACTION; 00165 msgTx.format=CANStandard; 00166 msgTx.type=CANData; 00167 msgTx.len=2; 00168 speed = BRAS_AVANT; 00169 if (InversStrat){ // si on est inversé, on echange les bras 00170 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00171 else speed = BRAS_AVANT; 00172 } 00173 if (speed == BRAS_AVANT)localData = 0x0A; 00174 else localData = 0x1E; 00175 00176 msgTx.data[0]=localData; 00177 msgTx.data[1]=speed; 00178 can1.write(msgTx); 00179 break; 00180 00181 case 112: // bouger module avant la gouttière 00182 msgTx.id=SERVO_AX12_ACTION; 00183 msgTx.format=CANStandard; 00184 msgTx.type=CANData; 00185 msgTx.len=2; 00186 speed = BRAS_AVANT; 00187 if (InversStrat){ // si on est inversé, on echange les bras 00188 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00189 else speed = BRAS_AVANT; 00190 } 00191 if (speed == BRAS_AVANT)localData = 0x0B; 00192 else localData = 0x1F; 00193 00194 msgTx.data[0]=localData; 00195 msgTx.data[1]=speed; 00196 can1.write(msgTx); 00197 break; 00198 00199 case 113: // deposer module 00200 msgTx.id=SERVO_AX12_ACTION; 00201 msgTx.format=CANStandard; 00202 msgTx.type=CANData; 00203 msgTx.len=2; 00204 localData2 = BRAS_AVANT; 00205 00206 if (InversStrat){ // si on est inversé, on echange les bras 00207 if (localData2 == BRAS_AVANT) localData2 = BRAS_ARRIERE; 00208 else localData2 = BRAS_AVANT; 00209 } 00210 00211 if(localData2 == BRAS_AVANT){ 00212 if(speed == INV){ 00213 localData = 0x0E; 00214 }else{ 00215 localData = 0x0C; 00216 } 00217 }else{ 00218 if(speed == INV){ 00219 localData = 0x22; 00220 }else{ 00221 localData = 0x20; 00222 } 00223 } 00224 00225 msgTx.data[0]=localData; 00226 msgTx.data[1]=localData2; 00227 can1.write(msgTx); 00228 break; 00229 00230 case 114: // repositionner bras avant 00231 msgTx.id=SERVO_AX12_ACTION; 00232 msgTx.format=CANStandard; 00233 msgTx.type=CANData; 00234 msgTx.len=2; 00235 speed = BRAS_AVANT; 00236 if (InversStrat){ // si on est inversé, on echange les bras 00237 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00238 else speed = BRAS_AVANT; 00239 } 00240 if (speed == BRAS_AVANT)localData = 0x0F; 00241 else localData = 0x23; 00242 00243 msgTx.data[0]=localData; 00244 msgTx.data[1]=speed; 00245 can1.write(msgTx); 00246 break; 00247 00248 case 115: // pompe avant ON 00249 msgTx.id=0x400; 00250 msgTx.format=CANStandard; 00251 msgTx.type=CANData; 00252 msgTx.len=2; 00253 localData = BRAS_AVANT; 00254 if (InversStrat){ // si on est inversé, on echange les bras 00255 if (localData == BRAS_AVANT) localData = BRAS_ARRIERE; 00256 else localData = BRAS_AVANT; 00257 } 00258 00259 msgTx.data[0]=1; 00260 msgTx.data[1]=localData; 00261 00262 can1.write(msgTx); 00263 wait_ms(1); 00264 can1.write(msgTx); 00265 waitingAckID= 0; 00266 waitingAckFrom = 0; 00267 break; 00268 00269 case 116: // pompe avant OFF 00270 msgTx.id=0x400; 00271 msgTx.format=CANStandard; 00272 msgTx.type=CANData; 00273 msgTx.len=2; 00274 localData = BRAS_AVANT; 00275 if (InversStrat){ // si on est inversé, on echange les bras 00276 if (localData == BRAS_AVANT) localData = BRAS_ARRIERE; 00277 else localData = BRAS_AVANT; 00278 } 00279 00280 msgTx.data[0]=0; 00281 msgTx.data[1]=localData; 00282 00283 can1.write(msgTx); 00284 wait_ms(1); 00285 can1.write(msgTx); 00286 waitingAckID= 0; 00287 waitingAckFrom = 0; 00288 break; 00289 00290 case 120: // repos bras arrière 00291 msgTx.id=SERVO_AX12_ACTION; 00292 msgTx.format=CANStandard; 00293 msgTx.type=CANData; 00294 msgTx.len=2; 00295 speed = BRAS_ARRIERE; 00296 if (InversStrat){ // si on est inversé, on echange les bras 00297 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00298 else speed = BRAS_AVANT; 00299 } 00300 if (speed == BRAS_AVANT)localData = 0x10; 00301 else localData = 0x24; 00302 00303 msgTx.data[0]=localData; 00304 msgTx.data[1]=speed; 00305 can1.write(msgTx); 00306 break; 00307 00308 case 121: // position d'init avant de prendre un module bras arriere 00309 msgTx.id=SERVO_AX12_ACTION; 00310 msgTx.format=CANStandard; 00311 msgTx.type=CANData; 00312 msgTx.len=2; 00313 speed = BRAS_ARRIERE; 00314 if (InversStrat){ // si on est inversé, on echange les bras 00315 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00316 else speed = BRAS_AVANT; 00317 } 00318 if (speed == BRAS_AVANT)localData = 0xA0; 00319 else localData = 0x1E; 00320 00321 msgTx.data[0]=localData; 00322 msgTx.data[1]=speed; 00323 can1.write(msgTx); 00324 break; 00325 00326 case 122: // bouger le bras arriere avant la gouttiere 00327 msgTx.id=SERVO_AX12_ACTION; 00328 msgTx.format=CANStandard; 00329 msgTx.type=CANData; 00330 msgTx.len=2; 00331 speed = BRAS_ARRIERE; 00332 if (InversStrat){ // si on est inversé, on echange les bras 00333 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00334 else speed = BRAS_AVANT; 00335 } 00336 if (speed == BRAS_AVANT)localData = 0x0B; 00337 else localData = 0x1F; 00338 00339 msgTx.data[0]=localData; 00340 msgTx.data[1]=speed; 00341 can1.write(msgTx); 00342 break; 00343 00344 case 123: // deposer module 00345 msgTx.id=SERVO_AX12_ACTION; 00346 msgTx.format=CANStandard; 00347 msgTx.type=CANData; 00348 msgTx.len=2; 00349 localData2 = BRAS_ARRIERE; 00350 00351 if (InversStrat){ // si on est inversé, on echange les bras 00352 if (localData2 == BRAS_AVANT) localData2 = BRAS_ARRIERE; 00353 else localData2 = BRAS_AVANT; 00354 } 00355 00356 if(localData2 == BRAS_AVANT){ 00357 if(speed == INV){ 00358 localData = 0x0E; 00359 }else{ 00360 localData = 0x0C; 00361 } 00362 }else{ 00363 if(speed == INV){ 00364 localData = 0x22; 00365 }else{ 00366 localData = 0x20; 00367 } 00368 } 00369 00370 msgTx.data[0]=localData; 00371 msgTx.data[1]=localData2; 00372 can1.write(msgTx); 00373 break; 00374 00375 case 124: // remettre le bras arriere en posiion initiale 00376 msgTx.id=SERVO_AX12_ACTION; 00377 msgTx.format=CANStandard; 00378 msgTx.type=CANData; 00379 msgTx.len=2; 00380 speed = BRAS_ARRIERE; 00381 if (InversStrat){ // si on est inversé, on echange les bras 00382 if (speed == BRAS_AVANT) speed = BRAS_ARRIERE; 00383 else speed = BRAS_AVANT; 00384 } 00385 if (speed == BRAS_AVANT)localData = 0x0F; 00386 else localData = 0x23; 00387 00388 msgTx.data[0]=localData; 00389 msgTx.data[1]=speed; 00390 can1.write(msgTx); 00391 break; 00392 00393 case 125: // pompe pwm arriere ON 00394 msgTx.id=0x400; 00395 msgTx.format=CANStandard; 00396 msgTx.type=CANData; 00397 msgTx.len=2; 00398 localData = BRAS_ARRIERE; 00399 if (InversStrat){ // si on est inversé, on echange les bras 00400 if (localData == BRAS_AVANT) localData = BRAS_ARRIERE; 00401 else localData = BRAS_AVANT; 00402 } 00403 00404 msgTx.data[0]=1; 00405 msgTx.data[1]=localData; 00406 00407 can1.write(msgTx); 00408 wait_ms(1); 00409 can1.write(msgTx); 00410 00411 waitingAckID= 0; 00412 waitingAckFrom = 0; 00413 break; 00414 00415 case 126: // pompe arriere pwm OFF 00416 msgTx.id=0x400; 00417 msgTx.format=CANStandard; 00418 msgTx.type=CANData; 00419 msgTx.len=2; 00420 localData = BRAS_ARRIERE; 00421 if (InversStrat){ // si on est inversé, on echange les bras 00422 if (localData == BRAS_AVANT) localData = BRAS_ARRIERE; 00423 else localData = BRAS_AVANT; 00424 } 00425 00426 msgTx.data[0]=0; 00427 msgTx.data[1]=localData; 00428 00429 can1.write(msgTx); 00430 wait_ms(1); 00431 can1.write(msgTx); 00432 waitingAckID= 0; 00433 waitingAckFrom = 0; 00434 break; 00435 00436 case 130: // baiser bras turbine 00437 msgTx.id=SERVO_AX12_ACTION; 00438 msgTx.format=CANStandard; 00439 msgTx.type=CANData; 00440 msgTx.len=2; 00441 msgTx.data[0]=0x14; 00442 msgTx.data[1]=BRAS_AVANT; 00443 can1.write(msgTx); 00444 break; 00445 00446 case 131: // lever bras turbine 00447 msgTx.id=SERVO_AX12_ACTION; 00448 msgTx.format=CANStandard; 00449 msgTx.type=CANData; 00450 msgTx.len=2; 00451 msgTx.data[0]=0x15; 00452 msgTx.data[1]=BRAS_AVANT; 00453 can1.write(msgTx); 00454 break; 00455 00456 case 132: // allumer turbine 00457 msgTx.id=0x403; 00458 msgTx.format=CANStandard; 00459 msgTx.type=CANData; 00460 msgTx.len=2; 00461 msgTx.data[0]=1; 00462 msgTx.data[1]=BRAS_AVANT; 00463 can1.write(msgTx); 00464 break; 00465 00466 case 133: // stop turbine 00467 msgTx.id=0x403; 00468 msgTx.format=CANStandard; 00469 msgTx.type=CANData; 00470 msgTx.len=2; 00471 msgTx.data[0]=0; 00472 msgTx.data[1]=BRAS_AVANT; 00473 can1.write(msgTx); 00474 break; 00475 00476 case 140:// lanceur ON 00477 msgTx.id=0x402; 00478 msgTx.format=CANStandard; 00479 msgTx.type=CANData; 00480 msgTx.len=2; 00481 00482 msgTx.data[0]=1; 00483 msgTx.data[1]=0x02; 00484 can1.write(msgTx); 00485 break; 00486 00487 case 141: // lanceur OFF 00488 msgTx.id=0x402; 00489 msgTx.format=CANStandard; 00490 msgTx.type=CANData; 00491 msgTx.len=2; 00492 00493 msgTx.data[0]=0; 00494 msgTx.data[1]=0x02; 00495 can1.write(msgTx); 00496 break; 00497 00498 case 150: 00499 msgTx.id=0x404; 00500 msgTx.format=CANStandard; 00501 msgTx.type=CANData; 00502 msgTx.len=2; 00503 00504 msgTx.data[0]=1; 00505 msgTx.data[1]=BRAS_AVANT; 00506 00507 can1.write(msgTx); 00508 break; 00509 00510 case 151: 00511 msgTx.id=0x404; 00512 msgTx.format=CANStandard; 00513 msgTx.type=CANData; 00514 msgTx.len=2; 00515 00516 msgTx.data[0]=0; 00517 msgTx.data[1]=BRAS_AVANT; 00518 00519 can1.write(msgTx); 00520 break; 00521 00522 case 10://Désactiver le stop 00523 isStopEnable = 0; 00524 break; 00525 case 11://Activer le stop 00526 isStopEnable = 1; 00527 break; 00528 case 20://Désactiver l'asservissement 00529 setAsservissementEtat(0); 00530 break; 00531 case 21://Activer l'asservissement 00532 setAsservissementEtat(1); 00533 break; 00534 00535 case 22://Changer la vitesse du robot 00536 SendSpeed(speed,(unsigned short)angle); 00537 break; 00538 00539 case 30://Action tempo 00540 wait_ms(speed); 00541 break; 00542 00543 /*case 40: // demande au telemetre la position d'un objet 00544 //SendRawId(TELEMETRE_RECHERCHE_OBJET); 00545 00546 modeTelemetre = 1; 00547 00548 //angle = angle /10; 00549 00550 msgTx.id=TELEMETRE_OBJET; 00551 msgTx.format=CANStandard; 00552 msgTx.type=CANData; 00553 msgTx.len=1; 00554 // indice du module sur le terrain 00555 msgTx.data[0] = (unsigned char)speed; 00556 00557 00558 // x sur 2 octets 00559 msgTx.data[0]=(unsigned char)speed; 00560 msgTx.data[1]=(unsigned char)(speed>>8); 00561 // y sur 2 octets 00562 msgTx.data[2]=(unsigned char)angle; 00563 msgTx.data[3]=(unsigned char)(angle>>8); 00564 // theta signé sur 2 octets 00565 //msgTx.data[4]=(unsigned char)theta; 00566 //msgTx.data[5]=(unsigned char)(theta>>8); 00567 msgTx.data[4]=0; 00568 msgTx.data[5]=0; 00569 00570 can1.write(msgTx); 00571 00572 break;*/ 00573 00574 /* case 130://Lancer mouvement de sortie de la zone de départ 00575 msgTx.id=ACTION_BIG_DEMARRAGE; 00576 msgTx.format=CANStandard; 00577 msgTx.type=CANData; 00578 msgTx.len=1; 00579 msgTx.data[0] = (unsigned char)speed; 00580 can1.write(msgTx); 00581 break;*/ 00582 00583 default: 00584 return 0;//L'action n'existe pas, il faut utiliser le CAN 00585 00586 } 00587 return 1;//L'action est spécifique. 00588 00589 } 00590 00591 /****************************************************************************************/ 00592 /* FUNCTION NAME: initRobot */ 00593 /* DESCRIPTION : initialiser le robot */ 00594 /****************************************************************************************/ 00595 void initRobot(void) 00596 { 00597 //Enregistrement de tous les AX12 présent sur la carte 00598 /*AX12_register(5, AX12_SERIAL2); 00599 AX12_register(18, AX12_SERIAL2); 00600 AX12_register(13, AX12_SERIAL2); 00601 AX12_register(1, AX12_SERIAL1); 00602 AX12_register(11, AX12_SERIAL1); 00603 AX12_register(8, AX12_SERIAL1); 00604 AX12_register(7, AX12_SERIAL2);*/ 00605 00606 //AX12_setGoal(AX12_ID_FUNNY_ACTION, AX12_ANGLE_FUNNY_ACTION_CLOSE,AX12_SPEED_FUNNY_ACTION); 00607 //AX12_processChange(); 00608 //runRobotTest(); 00609 00610 } 00611 00612 /****************************************************************************************/ 00613 /* FUNCTION NAME: initRobotActionneur */ 00614 /* DESCRIPTION : Initialiser la position des actionneurs du robot */ 00615 /****************************************************************************************/ 00616 void initRobotActionneur(void) 00617 { 00618 00619 } 00620 00621 /****************************************************************************************/ 00622 /* FUNCTION NAME: runTest */ 00623 /* DESCRIPTION : tester l'ensemble des actionneurs du robot */ 00624 /****************************************************************************************/ 00625 void runRobotTest(void) 00626 { 00627 /* 00628 int waitTime = 500; 00629 00630 //Test des AX12 dans l'ordre 00631 doAction(111,0,0);//Fermeture pince arrière haute 00632 wait_ms(waitTime); 00633 doAction(110,0,0);//Ouverture pince arrière haute 00634 wait_ms(waitTime); 00635 doAction(113,0,0);//Fermeture pince arrière basse 00636 wait_ms(waitTime); 00637 doAction(112,0,0);//Ouverture pince arrière basse 00638 wait_ms(waitTime); 00639 doAction(115,0,0);//Fermeture porte arrière 00640 wait_ms(waitTime); 00641 doAction(114,0,0);//Ouverture porte arrière 00642 wait_ms(waitTime); 00643 doAction(101,0,0);//Fermer les portes avant 00644 wait_ms(waitTime); 00645 doAction(100,0,0);//Ouvrir les portes avant 00646 wait_ms(waitTime); 00647 doAction(103,0,0);//Descendre le peigne 00648 wait_ms(waitTime); 00649 doAction(102,0,0);//Remonter le peigne*/ 00650 } 00651 00652 /****************************************************************************************/ 00653 /* FUNCTION NAME: SelectStrategy */ 00654 /* DESCRIPTION : Charger le fichier de stratégie correspondante à un id */ 00655 /* RETURN : 0=> Erreur, 1=> OK si le fichier existe */ 00656 /****************************************************************************************/ 00657 int SelectStrategy(unsigned char id) 00658 { 00659 switch(id) 00660 { 00661 // strat de match 00662 case 1: 00663 strcpy(cheminFileStart,"/local/strat1.txt"); 00664 return FileExists(cheminFileStart); 00665 case 2: 00666 strcpy(cheminFileStart,"/local/strat2.txt"); 00667 return FileExists(cheminFileStart); 00668 case 3: 00669 strcpy(cheminFileStart,"/local/strat3.txt"); 00670 return FileExists(cheminFileStart); 00671 case 4: 00672 strcpy(cheminFileStart,"/local/strat4.txt"); 00673 return FileExists(cheminFileStart); 00674 case 5: 00675 strcpy(cheminFileStart,"/local/strat5.txt"); 00676 return FileExists(cheminFileStart); 00677 case 6: 00678 strcpy(cheminFileStart,"/local/strat6.txt"); 00679 return FileExists(cheminFileStart); 00680 case 7: 00681 strcpy(cheminFileStart,"/local/strat7.txt"); 00682 return FileExists(cheminFileStart); 00683 case 8: 00684 strcpy(cheminFileStart,"/local/strat8.txt"); 00685 return FileExists(cheminFileStart); 00686 case 9: 00687 strcpy(cheminFileStart,"/local/strat9.txt"); 00688 return FileExists(cheminFileStart); 00689 case 10: 00690 strcpy(cheminFileStart,"/local/strat10.txt"); 00691 return FileExists(cheminFileStart); 00692 00693 // strat de demo 00694 case 0x10: 00695 strcpy(cheminFileStart,"/local/moteur.txt"); 00696 return FileExists(cheminFileStart); 00697 case 0x11: 00698 #ifdef ROBOT_BIG 00699 strcpy(cheminFileStart,"/local/bras.txt"); 00700 #else 00701 strcpy(cheminFileStart,"/local/porteAvant.txt"); 00702 #endif 00703 return FileExists(cheminFileStart); 00704 case 0x12: 00705 #ifdef ROBOT_BIG 00706 strcpy(cheminFileStart,"/local/balancier.txt"); 00707 #else 00708 strcpy(cheminFileStart,"/local/mainTourneuse.txt"); 00709 #endif 00710 return FileExists(cheminFileStart); 00711 default: 00712 strcpy(cheminFileStart,"/local/strat1.txt"); 00713 return 0; 00714 } 00715 } 00716 00717 /****************************************************************************************/ 00718 /* FUNCTION NAME: needToStop */ 00719 /* DESCRIPTION : Savoir si il faut autoriser le stop du robot via balise */ 00720 /****************************************************************************************/ 00721 unsigned char needToStop(void) 00722 { 00723 return isStopEnable; 00724 } 00725 00726 /****************************************************************************************/ 00727 /* FUNCTION NAME: doBeforeEndAction */ 00728 /* DESCRIPTION : Terminer les actions du robot 1s avant la fin du match */ 00729 /****************************************************************************************/ 00730 void doBeforeEndAction(void) 00731 { 00732 doAction(110,0,0);//Ouverture pince arrière haute 00733 doAction(112,0,0);//Ouverture pince arrière basse 00734 doAction(114,0,0);//Ouverture porte arrière 00735 doAction(100,0,0);//Ouvrir les portes avant 00736 doAction(102,0,0);//Remonter le peigne 00737 } 00738 00739 #endif
Generated on Tue Jul 12 2022 19:28:48 by
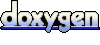