Strategie_13h30
Fork of CRAC-Strat_2017_homologation_gros_rob by
Embed:
(wiki syntax)
Show/hide line numbers
Serial.h
00001 /* mbed Microcontroller Library - Serial 00002 * Copyright (c) 2007-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_SERIAL_H 00006 #define MBED_SERIAL_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_SERIAL 00011 00012 #include "platform.h" 00013 #include "PinNames.h" 00014 #include "PeripheralNames.h" 00015 #include "Stream.h" 00016 #include "FunctionPointer.h" 00017 00018 namespace mbed { 00019 00020 /* Class: Serial 00021 * A serial port (UART) for communication with other serial devices 00022 * 00023 * Can be used for Full Duplex communication, or Simplex by specifying 00024 * one pin as NC (Not Connected) 00025 * 00026 * Example: 00027 * > // Print "Hello World" to the PC 00028 * > 00029 * > #include "mbed.h" 00030 * > 00031 * > Serial pc(USBTX, USBRX); 00032 * > 00033 * > int main() { 00034 * > pc.printf("Hello World\n"); 00035 * > } 00036 */ 00037 class Serial : public Stream { 00038 00039 public: 00040 00041 /* Constructor: Serial 00042 * Create a Serial port, connected to the specified transmit and receive pins 00043 * 00044 * Variables: 00045 * tx - Transmit pin 00046 * rx - Receive pin 00047 * 00048 * Note: Either tx or rx may be specified as NC if unused 00049 */ 00050 Serial(PinName tx, PinName rx, const char *name = NULL); 00051 00052 /* Function: baud 00053 * Set the baud rate of the serial port 00054 * 00055 * Variables: 00056 * baudrate - The baudrate of the serial port (default = 9600). 00057 */ 00058 void baud(int baudrate); 00059 00060 enum Parity { 00061 None = 0 00062 , Odd 00063 , Even 00064 , Forced1 00065 , Forced0 00066 }; 00067 00068 enum IrqType { 00069 RxIrq = 0 00070 , TxIrq 00071 }; 00072 00073 /* Function: format 00074 * Set the transmission format used by the Serial port 00075 * 00076 * Variables: 00077 * bits - The number of bits in a word (5-8; default = 8) 00078 * parity - The parity used (Serial::None, Serial::Odd, Serial::Even, Serial::Forced1, Serial::Forced0; default = Serial::None) 00079 * stop - The number of stop bits (1 or 2; default = 1) 00080 */ 00081 void format(int bits = 8, Parity parity = Serial::None, int stop_bits = 1); 00082 00083 #if 0 // Inhereted from Stream, for documentation only 00084 00085 /* Function: putc 00086 * Write a character 00087 * 00088 * Variables: 00089 * c - The character to write to the serial port 00090 */ 00091 int putc(int c); 00092 00093 /* Function: getc 00094 * Read a character 00095 * 00096 * Reads a character from the serial port. This will block until 00097 * a character is available. To see if a character is available, 00098 * see <readable> 00099 * 00100 * Variables: 00101 * returns - The character read from the serial port 00102 */ 00103 int getc(); 00104 00105 /* Function: printf 00106 * Write a formated string 00107 * 00108 * Variables: 00109 * format - A printf-style format string, followed by the 00110 * variables to use in formating the string. 00111 */ 00112 int printf(const char* format, ...); 00113 00114 /* Function: scanf 00115 * Read a formated string 00116 * 00117 * Variables: 00118 * format - A scanf-style format string, 00119 * followed by the pointers to variables to store the results. 00120 */ 00121 int scanf(const char* format, ...); 00122 00123 #endif 00124 00125 /* Function: readable 00126 * Determine if there is a character available to read 00127 * 00128 * Variables: 00129 * returns - 1 if there is a character available to read, else 0 00130 */ 00131 int readable(); 00132 00133 /* Function: writeable 00134 * Determine if there is space available to write a character 00135 * 00136 * Variables: 00137 * returns - 1 if there is space to write a character, else 0 00138 */ 00139 int writeable(); 00140 00141 /* Function: attach 00142 * Attach a function to call whenever a serial interrupt is generated 00143 * 00144 * Variables: 00145 * fptr - A pointer to a void function, or 0 to set as none 00146 * type - Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00147 */ 00148 void attach(void (*fptr)(void), IrqType type = RxIrq); 00149 00150 /* Function: attach 00151 * Attach a member function to call whenever a serial interrupt is generated 00152 * 00153 * Variables: 00154 * tptr - pointer to the object to call the member function on 00155 * mptr - pointer to the member function to be called 00156 * type - Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00157 */ 00158 template<typename T> 00159 void attach(T* tptr, void (T::*mptr)(void), IrqType type = RxIrq) { 00160 if((mptr != NULL) && (tptr != NULL)) { 00161 _irq[type].attach(tptr, mptr); 00162 setup_interrupt(type); 00163 } 00164 } 00165 00166 #ifdef MBED_RPC 00167 virtual const struct rpc_method *get_rpc_methods(); 00168 static struct rpc_class *get_rpc_class(); 00169 #endif 00170 00171 protected: 00172 00173 void setup_interrupt(IrqType type); 00174 void remove_interrupt(IrqType type); 00175 00176 virtual int _getc(); 00177 virtual int _putc(int c); 00178 00179 UARTName _uart; 00180 FunctionPointer _irq[2]; 00181 int _uidx; 00182 00183 }; 00184 00185 } // namespace mbed 00186 00187 #endif 00188 00189 #endif
Generated on Tue Jul 12 2022 19:28:48 by
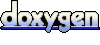