Strategie_13h30
Fork of CRAC-Strat_2017_homologation_gros_rob by
Embed:
(wiki syntax)
Show/hide line numbers
FileHandle.h
00001 /* mbed Microcontroller Library - FileHandler 00002 * Copyright (c) 2007-2009 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_FILEHANDLE_H 00006 #define MBED_FILEHANDLE_H 00007 00008 typedef int FILEHANDLE; 00009 00010 #include <stdio.h> 00011 #ifdef __ARMCC_VERSION 00012 typedef int ssize_t; 00013 typedef long off_t; 00014 #else 00015 #include <sys/types.h> 00016 #endif 00017 00018 namespace mbed { 00019 00020 /* Class FileHandle 00021 * An OO equivalent of the internal FILEHANDLE variable 00022 * and associated _sys_* functions 00023 * 00024 * FileHandle is an abstract class, needing at least sys_write and 00025 * sys_read to be implmented for a simple interactive device 00026 * 00027 * No one ever directly tals to/instanciates a FileHandle - it gets 00028 * created by FileSystem, and wrapped up by stdio 00029 */ 00030 class FileHandle { 00031 00032 public: 00033 00034 /* Function write 00035 * Write the contents of a buffer to the file 00036 * 00037 * Parameters 00038 * buffer - the buffer to write from 00039 * length - the number of characters to write 00040 * 00041 * Returns 00042 * The number of characters written (possibly 0) on success, -1 on error. 00043 */ 00044 virtual ssize_t write(const void* buffer, size_t length) = 0; 00045 00046 /* Function close 00047 * Close the file 00048 * 00049 * Returns 00050 * Zero on success, -1 on error. 00051 */ 00052 virtual int close() = 0; 00053 00054 /* Function read 00055 * Reads the contents of the file into a buffer 00056 * 00057 * Parameters 00058 * buffer - the buffer to read in to 00059 * length - the number of characters to read 00060 * 00061 * Returns 00062 * The number of characters read (zero at end of file) on success, -1 on error. 00063 */ 00064 virtual ssize_t read(void* buffer, size_t length) = 0; 00065 00066 /* Function isatty 00067 * Check if the handle is for a interactive terminal device 00068 * 00069 * If so, line buffered behaviour is used by default 00070 * 00071 * Returns 00072 * 1 if it is a terminal, 0 otherwise 00073 */ 00074 virtual int isatty() = 0 ; 00075 00076 /* Function lseek 00077 * Move the file position to a given offset from a given location. 00078 * 00079 * Parameters 00080 * offset - The offset from whence to move to 00081 * whence - SEEK_SET for the start of the file, SEEK_CUR for the 00082 * current file position, or SEEK_END for the end of the file. 00083 * 00084 * Returns 00085 * New file position on success, -1 on failure or unsupported 00086 */ 00087 virtual off_t lseek(off_t offset, int whence) = 0; 00088 00089 /* Function fsync 00090 * Flush any buffers associated with the FileHandle, ensuring it 00091 * is up to date on disk 00092 * 00093 * Returns 00094 * 0 on success or un-needed, -1 on error 00095 */ 00096 virtual int fsync() = 0; 00097 00098 virtual off_t flen() { 00099 /* remember our current position */ 00100 off_t pos = lseek(0, SEEK_CUR); 00101 if(pos == -1) return -1; 00102 /* seek to the end to get the file length */ 00103 off_t res = lseek(0, SEEK_END); 00104 /* return to our old position */ 00105 lseek(pos, SEEK_SET); 00106 return res; 00107 } 00108 00109 }; 00110 00111 } // namespace mbed 00112 00113 #endif 00114
Generated on Tue Jul 12 2022 19:28:47 by
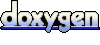