Strategie_13h30
Fork of CRAC-Strat_2017_homologation_gros_rob by
Embed:
(wiki syntax)
Show/hide line numbers
DigitalOut.h
00001 /* mbed Microcontroller Library - DigitalOut 00002 * Copyright (c) 2006-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_DIGITALOUT_H 00006 #define MBED_DIGITALOUT_H 00007 00008 #include "platform.h" 00009 #include "PinNames.h" 00010 #include "PeripheralNames.h" 00011 #include "Base.h" 00012 00013 namespace mbed { 00014 00015 /* Class: DigitalOut 00016 * A digital output, used for setting the state of a pin 00017 * 00018 * Example: 00019 * > // Toggle a LED 00020 * > #include "mbed.h" 00021 * > 00022 * > DigitalOut led(LED1); 00023 * > 00024 * > int main() { 00025 * > while(1) { 00026 * > led = !led; 00027 * > wait(0.2); 00028 * > } 00029 * > } 00030 */ 00031 class DigitalOut : public Base { 00032 00033 public: 00034 00035 /* Constructor: DigitalOut 00036 * Create a DigitalOut connected to the specified pin 00037 * 00038 * Variables: 00039 * pin - DigitalOut pin to connect to 00040 */ 00041 DigitalOut(PinName pin, const char* name = NULL); 00042 00043 /* Function: write 00044 * Set the output, specified as 0 or 1 (int) 00045 * 00046 * Variables: 00047 * value - An integer specifying the pin output value, 00048 * 0 for logical 0 and 1 (or any other non-zero value) for logical 1 00049 */ 00050 void write(int value) { 00051 00052 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00053 00054 if(value) { 00055 _gpio->FIOSET = _mask; 00056 } else { 00057 _gpio->FIOCLR = _mask; 00058 } 00059 00060 #elif defined(TARGET_LPC11U24) 00061 00062 if(value) { 00063 LPC_GPIO->SET[_index] = _mask; 00064 } else { 00065 LPC_GPIO->CLR[_index] = _mask; 00066 } 00067 #endif 00068 00069 } 00070 00071 /* Function: read 00072 * Return the output setting, represented as 0 or 1 (int) 00073 * 00074 * Variables: 00075 * returns - An integer representing the output setting of the pin, 00076 * 0 for logical 0 and 1 for logical 1 00077 */ 00078 int read() { 00079 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00080 return ((_gpio->FIOPIN & _mask) ? 1 : 0); 00081 #elif defined(TARGET_LPC11U24) 00082 return ((LPC_GPIO->PIN[_index] & _mask) ? 1 : 0); 00083 #endif 00084 00085 } 00086 00087 00088 #ifdef MBED_OPERATORS 00089 /* Function: operator= 00090 * A shorthand for <write> 00091 */ 00092 DigitalOut& operator= (int value) { 00093 write(value); 00094 return *this; 00095 } 00096 00097 DigitalOut& operator= (DigitalOut& rhs) { 00098 write(rhs.read()); 00099 return *this; 00100 } 00101 00102 00103 /* Function: operator int() 00104 * A shorthand for <read> 00105 */ 00106 operator int() { 00107 return read(); 00108 } 00109 00110 #endif 00111 00112 #ifdef MBED_RPC 00113 virtual const struct rpc_method *get_rpc_methods(); 00114 static struct rpc_class *get_rpc_class(); 00115 #endif 00116 00117 protected: 00118 00119 PinName _pin; 00120 00121 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00122 LPC_GPIO_TypeDef *_gpio; 00123 #elif defined(TARGET_LPC11U24) 00124 int _index; 00125 #endif 00126 00127 uint32_t _mask; 00128 00129 00130 }; 00131 00132 } // namespace mbed 00133 00134 #endif
Generated on Tue Jul 12 2022 19:28:47 by
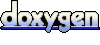