Strategie_13h30
Fork of CRAC-Strat_2017_homologation_gros_rob by
Embed:
(wiki syntax)
Show/hide line numbers
DigitalIn.h
00001 /* mbed Microcontroller Library - DigitalIn 00002 * Copyright (c) 2006-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_DIGITALIN_H 00006 #define MBED_DIGITALIN_H 00007 00008 #include "platform.h" 00009 #include "PinNames.h" 00010 #include "PeripheralNames.h" 00011 #include "Base.h" 00012 00013 namespace mbed { 00014 00015 /* Class: DigitalIn 00016 * A digital input, used for reading the state of a pin 00017 * 00018 * Example: 00019 * > // Flash an LED while a DigitalIn is true 00020 * > 00021 * > #include "mbed.h" 00022 * > 00023 * > DigitalIn enable(p5); 00024 * > DigitalOut led(LED1); 00025 * > 00026 * > int main() { 00027 * > while(1) { 00028 * > if(enable) { 00029 * > led = !led; 00030 * > } 00031 * > wait(0.25); 00032 * > } 00033 * > } 00034 */ 00035 class DigitalIn : public Base { 00036 00037 public: 00038 00039 /* Constructor: DigitalIn 00040 * Create a DigitalIn connected to the specified pin 00041 * 00042 * Variables: 00043 * pin - DigitalIn pin to connect to 00044 * name - (optional) A string to identify the object 00045 */ 00046 DigitalIn(PinName pin, const char *name = NULL); 00047 00048 /* Function: read 00049 * Read the input, represented as 0 or 1 (int) 00050 * 00051 * Variables: 00052 * returns - An integer representing the state of the input pin, 00053 * 0 for logical 0 and 1 for logical 1 00054 */ 00055 int read() { 00056 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00057 return ((_gpio->FIOPIN & _mask) ? 1 : 0); 00058 #elif defined(TARGET_LPC11U24) 00059 return ((LPC_GPIO->PIN[_index] & _mask) ? 1 : 0); 00060 #endif 00061 } 00062 00063 00064 /* Function: mode 00065 * Set the input pin mode 00066 * 00067 * Variables: 00068 * mode - PullUp, PullDown, PullNone, OpenDrain 00069 */ 00070 void mode(PinMode pull); 00071 00072 #ifdef MBED_OPERATORS 00073 /* Function: operator int() 00074 * An operator shorthand for <read()> 00075 */ 00076 operator int() { 00077 return read(); 00078 } 00079 00080 #endif 00081 00082 #ifdef MBED_RPC 00083 virtual const struct rpc_method *get_rpc_methods(); 00084 static struct rpc_class *get_rpc_class(); 00085 #endif 00086 00087 protected: 00088 00089 PinName _pin; 00090 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00091 LPC_GPIO_TypeDef *_gpio; 00092 #elif defined(TARGET_LPC11U24) 00093 int _index; 00094 #endif 00095 uint32_t _mask; 00096 00097 }; 00098 00099 } // namespace mbed 00100 00101 #endif 00102
Generated on Tue Jul 12 2022 19:28:47 by
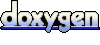