
Transmit pressure/temperature reading once per hour from xDot to iupivot server
Dependencies: ATParser MPL3115A2 mbed
main.cpp
00001 #include "mbed.h" 00002 #include "MPL3115A2.h" 00003 #include "string.h" 00004 #include "ATParser.h" 00005 00006 MPL3115A2 pressure_sensor(PB_7,PB_6,0x60); 00007 BufferedSerial pc(SERIAL_TX, SERIAL_RX); 00008 BufferedSerial device(PA_9, PA_10); // tx, rx 00009 DigitalOut myled(LED1); 00010 00011 00012 /* Checks to see if the xDot has a buffered message OK to 00013 confirm that operation has succeeded 00014 */ 00015 int confirmOK() { 00016 // Wait until device has a response 00017 while (!device.readable()) { 00018 wait_ms(100); 00019 } 00020 int bufferSize = 100; 00021 char buf[bufferSize]; 00022 int i = 0; 00023 for (i = 0; i < bufferSize; i++) { 00024 buf[i] = 0; 00025 } 00026 i = 0; 00027 00028 //Read message from device into buffer 00029 for (i = 0; device.readable(); i++) { 00030 buf[i] = device.getc(); 00031 if (buf[i] == '\n') { 00032 // Read new line so check to see if we got an OK or Error 00033 // else keep reading til we get one 00034 //pc.printf("The message from the device is %s\r\n",buf); 00035 if (!strncmp("OK",buf,2)) { 00036 //pc.printf("I compared against %s and got OK\r\n",buf); 00037 return 1; 00038 } else if (!strncmp("ERROR",buf,5)) { 00039 //pc.printf("I compared against %s and got ERROR\r\n",buf); 00040 return 0; 00041 } 00042 return confirmOK(); 00043 } 00044 if (i >= bufferSize) { 00045 return 0; 00046 } 00047 } 00048 return confirmOK(); 00049 } 00050 /* Sends a message to the xDot 00051 Messages are expected to end in \n so that an OK confirmation will be 00052 recieved 00053 */ 00054 int sendAtMessage(char * message) { 00055 while (*message) { 00056 device.putc(*message); 00057 message++; 00058 } 00059 return confirmOK(); 00060 } 00061 00062 /* 00063 Send a message to the xDot without checking for acknowledge 00064 */ 00065 void sendAtMessageNACK(char * message, int size) { 00066 int i = 0; 00067 while (i < size) { 00068 device.putc(*message); 00069 //wait_ms(500); // Not succesfully sending the message unless it waits. 00070 //pc.printf("putting %c on device\n\r", *message); 00071 message++; 00072 i++; 00073 } 00074 } 00075 int main() { 00076 pc.baud(115200); 00077 device.baud(115200); 00078 00079 pc.printf("Starting program\n\r"); 00080 00081 myled = 0; 00082 00083 if (!sendAtMessage("AT\n")) { 00084 pc.printf("xDot not responding to message\n\r"); 00085 } 00086 //Try to connect xDot to network 00087 // Provice network name 00088 char networkName[] = "AT+NI=1,MTCDT-19400691\n"; 00089 if (!sendAtMessage(networkName)) { 00090 pc.printf("Unable to set network name\n\r"); 00091 } 00092 //Provide network passphrase 00093 char networkPassphrase[] = "AT+NK=1,MTCDT-19400691\n"; 00094 if (!sendAtMessage(networkPassphrase)) { 00095 pc.printf("Network passphrase not successfully set\n\r"); 00096 } 00097 //Set the frequency sub band 00098 char frequencySubBand[] = "AT+FSB=1\n"; 00099 if (!sendAtMessage(frequencySubBand)) { 00100 pc.printf("Network frequency SubBand not successfully set\n\r"); 00101 } 00102 //Join the network 00103 char joinNetwork[] = "AT+JOIN\n"; // Unable to join network atm. 00104 if (!sendAtMessage(joinNetwork)) { 00105 pc.printf("Failed to joined the network\n\r"); 00106 } 00107 pc.printf("I got here\r\n"); 00108 int i = 0; 00109 for (i = 0; i < 48; i++) { 00110 int tempBufSize = 4; 00111 int presBufSize = 6; 00112 double temp = pressure_sensor.getTemperature(); 00113 char tempBuf[tempBufSize]; 00114 double pres = pressure_sensor.getPressure(); 00115 char presBuf[presBufSize]; 00116 //pc.printf("Current temp is %f\n\r",temp); 00117 //pc.printf("Current pres is %f\n\r",pres); 00118 sprintf(tempBuf, "%2.1f",temp); 00119 sprintf(presBuf, "%4.1f",pres); 00120 //pc.printf("After convert with sprintf temp = %s\n\r",tempBuf); 00121 //pc.printf("After convert with sprintf pres = %s\n\r",presBuf); 00122 sendAtMessageNACK("AT+SEND=",8); 00123 sendAtMessageNACK(tempBuf,tempBufSize); 00124 sendAtMessageNACK(",",1); 00125 sendAtMessageNACK(presBuf,presBufSize); 00126 //wait_ms(500); 00127 if (sendAtMessage("\n")) { 00128 pc.printf("message succesfully sent\n\r"); 00129 myled = 1; 00130 } else { 00131 pc.printf("Failed to send the message for some reason\r\n"); 00132 } 00133 00134 wait(1000); 00135 00136 } 00137 00138 //When an s is read from the serial pc, 00139 // read the current pressure/temperature from the I2C pressure sensor 00140 // and send it to the MQTT server pivot.iuiot 00141 //Unsure of what size to set Buffer so that it doesn't go over 00142 //when trying to send a message 00143 00144 /* 00145 while(1) { 00146 if (pc.readable()) { 00147 //pc.printf("pc.readable\r\n"); 00148 if (pc.getc() == 's') { 00149 pc.printf("I recieved an s\r\n"); 00150 int tempBufSize = 4; 00151 int presBufSize = 6; 00152 double temp = pressure_sensor.getTemperature(); 00153 char tempBuf[tempBufSize]; 00154 double pres = pressure_sensor.getPressure(); 00155 char presBuf[presBufSize]; 00156 pc.printf("Current temp is %f\n\r",temp); 00157 pc.printf("Current pres is %f\n\r",pres); 00158 sprintf(tempBuf, "%2.1f",temp); 00159 sprintf(presBuf, "%4.1f",pres); 00160 pc.printf("After convert with sprintf temp = %s\n\r",tempBuf); 00161 pc.printf("After convert with sprintf pres = %s\n\r",presBuf); 00162 sendAtMessageNACK("AT+SEND=",8); 00163 sendAtMessageNACK(tempBuf,tempBufSize); 00164 sendAtMessageNACK(",",1); 00165 sendAtMessageNACK(presBuf,presBufSize); 00166 wait_ms(500); 00167 if (sendAtMessage("\n")) { 00168 pc.printf("message succesfully sent\n\r"); 00169 } else { 00170 pc.printf("Failed to send the message for some reason\r\n"); 00171 } 00172 } 00173 } 00174 } 00175 */ 00176 00177 /* 00178 while(1) { 00179 if(pc.readable()) { 00180 device.putc(pc.getc()); 00181 myled = !myled; 00182 } 00183 if(device.readable()) { 00184 pc.putc(device.getc()); 00185 myled = !myled; 00186 } 00187 } 00188 */ 00189 }
Generated on Fri Jul 15 2022 22:49:04 by
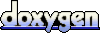