
(Working) Code to interface 3 LoadCells to ADISense1000 and display values using the Labview code.
Fork of 4Bridge_ADISense1000_Example_copy by
adi_sense_gpio.cpp
00001 /*! 00002 ****************************************************************************** 00003 * @file: adi_sense_gpio.cpp 00004 * @brief: ADI Sense OS-dependent wrapper layer for GPIO interface 00005 *----------------------------------------------------------------------------- 00006 */ 00007 00008 /****************************************************************************** 00009 Copyright 2017 (c) Analog Devices, Inc. 00010 00011 All rights reserved. 00012 00013 Redistribution and use in source and binary forms, with or without 00014 modification, are permitted provided that the following conditions are met: 00015 - Redistributions of source code must retain the above copyright 00016 notice, this list of conditions and the following disclaimer. 00017 - Redistributions in binary form must reproduce the above copyright 00018 notice, this list of conditions and the following disclaimer in 00019 the documentation and/or other materials provided with the 00020 distribution. 00021 - Neither the name of Analog Devices, Inc. nor the names of its 00022 contributors may be used to endorse or promote products derived 00023 from this software without specific prior written permission. 00024 - The use of this software may or may not infringe the patent rights 00025 of one or more patent holders. This license does not release you 00026 from the requirement that you obtain separate licenses from these 00027 patent holders to use this software. 00028 - Use of the software either in source or binary form, must be run 00029 on or directly connected to an Analog Devices Inc. component. 00030 00031 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00032 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00033 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00034 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00035 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00036 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00037 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00038 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00039 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00040 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00041 * 00042 *****************************************************************************/ 00043 00044 #include <mbed.h> 00045 00046 #include "inc/adi_sense_gpio.h" 00047 #include "inc/adi_sense_log.h" 00048 00049 class GpioContext 00050 { 00051 public: 00052 GpioContext( 00053 PinName resetPin, 00054 PinName errorPin, 00055 PinName alertPin, 00056 PinName datareadyPin) 00057 : _reset(resetPin), 00058 _error(errorPin), 00059 _alert(alertPin), 00060 _dataready(datareadyPin), 00061 _errorIrq(errorPin), 00062 _alertIrq(alertPin), 00063 _datareadyIrq(datareadyPin) {} 00064 00065 ADI_SENSE_RESULT get( 00066 ADI_SENSE_GPIO_PIN ePinId, 00067 bool_t *pState); 00068 00069 ADI_SENSE_RESULT set( 00070 ADI_SENSE_GPIO_PIN ePinId, 00071 bool_t state); 00072 00073 ADI_SENSE_RESULT enableIrq( 00074 ADI_SENSE_GPIO_PIN ePinId, 00075 ADI_SENSE_GPIO_CALLBACK callbackFn, 00076 void *pArg); 00077 00078 ADI_SENSE_RESULT disableIrq( 00079 ADI_SENSE_GPIO_PIN ePinId); 00080 00081 private: 00082 DigitalOut _reset; 00083 00084 DigitalIn _error; 00085 DigitalIn _alert; 00086 DigitalIn _dataready; 00087 00088 InterruptIn _errorIrq; 00089 InterruptIn _alertIrq; 00090 InterruptIn _datareadyIrq; 00091 00092 ADI_SENSE_GPIO_CALLBACK _errorIrqCallback; 00093 ADI_SENSE_GPIO_CALLBACK _alertIrqCallback; 00094 ADI_SENSE_GPIO_CALLBACK _datareadyIrqCallback; 00095 00096 void *_errorIrqArg; 00097 void *_alertIrqArg; 00098 void *_datareadyIrqArg; 00099 00100 void _errorIrqHandler() 00101 { 00102 _errorIrqCallback(ADI_SENSE_GPIO_PIN_ERROR , _errorIrqArg); 00103 } 00104 void _alertIrqHandler() 00105 { 00106 _alertIrqCallback(ADI_SENSE_GPIO_PIN_ALERT , _alertIrqArg); 00107 } 00108 void _datareadyIrqHandler() 00109 { 00110 _datareadyIrqCallback(ADI_SENSE_GPIO_PIN_DATAREADY , _datareadyIrqArg); 00111 } 00112 }; 00113 00114 ADI_SENSE_RESULT GpioContext::get( 00115 ADI_SENSE_GPIO_PIN ePinId, 00116 bool_t *pState) 00117 { 00118 switch(ePinId) 00119 { 00120 case ADI_SENSE_GPIO_PIN_ERROR : 00121 *pState = _error; 00122 return ADI_SENSE_SUCCESS ; 00123 case ADI_SENSE_GPIO_PIN_ALERT : 00124 *pState = _alert; 00125 return ADI_SENSE_SUCCESS ; 00126 case ADI_SENSE_GPIO_PIN_DATAREADY : 00127 *pState = _dataready; 00128 return ADI_SENSE_SUCCESS ; 00129 case ADI_SENSE_GPIO_PIN_RESET : 00130 *pState = _reset; 00131 return ADI_SENSE_SUCCESS ; 00132 default: 00133 return ADI_SENSE_INVALID_DEVICE_NUM ; 00134 } 00135 } 00136 00137 ADI_SENSE_RESULT GpioContext::set( 00138 ADI_SENSE_GPIO_PIN ePinId, 00139 bool_t state) 00140 { 00141 switch(ePinId) 00142 { 00143 case ADI_SENSE_GPIO_PIN_RESET : 00144 _reset = state; 00145 break; 00146 default: 00147 return ADI_SENSE_INVALID_DEVICE_NUM ; 00148 } 00149 00150 return ADI_SENSE_SUCCESS ; 00151 } 00152 00153 ADI_SENSE_RESULT GpioContext::enableIrq( 00154 ADI_SENSE_GPIO_PIN ePinId, 00155 ADI_SENSE_GPIO_CALLBACK callbackFn, 00156 void *pArg) 00157 { 00158 switch(ePinId) 00159 { 00160 case ADI_SENSE_GPIO_PIN_ERROR : 00161 _errorIrqCallback = callbackFn; 00162 _errorIrqArg = pArg; 00163 _errorIrq.rise(callback(this, &GpioContext::_errorIrqHandler)); 00164 return ADI_SENSE_SUCCESS ; 00165 case ADI_SENSE_GPIO_PIN_ALERT : 00166 _alertIrqCallback = callbackFn; 00167 _alertIrqArg = pArg; 00168 _alertIrq.rise(callback(this, &GpioContext::_alertIrqHandler)); 00169 return ADI_SENSE_SUCCESS ; 00170 case ADI_SENSE_GPIO_PIN_DATAREADY : 00171 _datareadyIrqCallback = callbackFn; 00172 _datareadyIrqArg = pArg; 00173 _datareadyIrq.rise(callback(this, &GpioContext::_datareadyIrqHandler)); 00174 return ADI_SENSE_SUCCESS ; 00175 default: 00176 return ADI_SENSE_INVALID_DEVICE_NUM ; 00177 } 00178 } 00179 00180 ADI_SENSE_RESULT GpioContext::disableIrq( 00181 ADI_SENSE_GPIO_PIN ePinId) 00182 { 00183 switch(ePinId) 00184 { 00185 case ADI_SENSE_GPIO_PIN_ERROR : 00186 _errorIrq.rise(NULL); 00187 return ADI_SENSE_SUCCESS ; 00188 case ADI_SENSE_GPIO_PIN_ALERT : 00189 _alertIrq.rise(NULL); 00190 return ADI_SENSE_SUCCESS ; 00191 case ADI_SENSE_GPIO_PIN_DATAREADY : 00192 _datareadyIrq.rise(NULL); 00193 return ADI_SENSE_SUCCESS ; 00194 default: 00195 return ADI_SENSE_INVALID_DEVICE_NUM ; 00196 } 00197 } 00198 00199 #ifdef __cplusplus 00200 extern "C" { 00201 #endif 00202 00203 /* 00204 * Open the GPIO interface and allocate resources 00205 */ 00206 ADI_SENSE_RESULT adi_sense_GpioOpen( 00207 ADI_SENSE_PLATFORM_GPIO_CONFIG *pConfig, 00208 ADI_SENSE_GPIO_HANDLE *phDevice) 00209 { 00210 GpioContext *pCtx = new GpioContext((PinName)pConfig->resetPin, 00211 (PinName)pConfig->errorPin, 00212 (PinName)pConfig->alertPin, 00213 (PinName)pConfig->datareadyPin); 00214 if (!pCtx) 00215 { 00216 ADI_SENSE_LOG_ERROR("Failed to allocate memory for GPIO context"); 00217 return ADI_SENSE_NO_MEM ; 00218 } 00219 00220 *phDevice = reinterpret_cast<ADI_SENSE_GPIO_HANDLE >(pCtx); 00221 return ADI_SENSE_SUCCESS ; 00222 } 00223 00224 /* 00225 * Get the state of the specified GPIO pin 00226 */ 00227 ADI_SENSE_RESULT adi_sense_GpioGet( 00228 ADI_SENSE_GPIO_HANDLE hDevice, 00229 ADI_SENSE_GPIO_PIN ePinId, 00230 bool_t *pbState) 00231 { 00232 GpioContext *pCtx = reinterpret_cast<GpioContext *>(hDevice); 00233 00234 return pCtx->get(ePinId, pbState); 00235 } 00236 00237 /* 00238 * Set the state of the specified GPIO pin 00239 */ 00240 ADI_SENSE_RESULT adi_sense_GpioSet( 00241 ADI_SENSE_GPIO_HANDLE hDevice, 00242 ADI_SENSE_GPIO_PIN ePinId, 00243 bool_t bState) 00244 { 00245 GpioContext *pCtx = reinterpret_cast<GpioContext *>(hDevice); 00246 00247 return pCtx->set(ePinId, bState); 00248 } 00249 00250 /* 00251 * Enable interrupt notifications on the specified GPIO pin 00252 */ 00253 ADI_SENSE_RESULT adi_sense_GpioIrqEnable( 00254 ADI_SENSE_GPIO_HANDLE hDevice, 00255 ADI_SENSE_GPIO_PIN ePinId, 00256 ADI_SENSE_GPIO_CALLBACK callback, 00257 void *arg) 00258 { 00259 GpioContext *pCtx = reinterpret_cast<GpioContext *>(hDevice); 00260 00261 return pCtx->enableIrq(ePinId, callback, arg); 00262 } 00263 00264 /* 00265 * Disable interrupt notifications on the specified GPIO pin 00266 */ 00267 ADI_SENSE_RESULT adi_sense_GpioIrqDisable( 00268 ADI_SENSE_GPIO_HANDLE hDevice, 00269 ADI_SENSE_GPIO_PIN ePinId) 00270 { 00271 GpioContext *pCtx = reinterpret_cast<GpioContext *>(hDevice); 00272 00273 return pCtx->disableIrq(ePinId); 00274 } 00275 00276 /* 00277 * Close the GPIO interface and free resources 00278 */ 00279 void adi_sense_GpioClose( 00280 ADI_SENSE_GPIO_HANDLE hDevice) 00281 { 00282 GpioContext *pCtx = reinterpret_cast<GpioContext *>(hDevice); 00283 00284 delete pCtx; 00285 } 00286 00287 #ifdef __cplusplus 00288 } 00289 #endif 00290
Generated on Tue Jul 12 2022 21:13:17 by
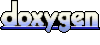