Implemented first Hangar-Service
Dependencies: CalibrateMagneto QuaternionMath
Fork of SML2 by
Sensor.h
00001 #ifndef _H_SENSOR_H 00002 #define _H_SENSOR_H 00003 00004 #include "Vector3.h" 00005 00006 /// Base class for I2C-connected sensors. Defines functionality supported by all sensors. 00007 class Sensor 00008 { 00009 public: 00010 /// Defines protocol used to send data back to owner. Derive from this class and use Sensor.setDelegate() to receive sensor updates. 00011 class Delegate 00012 { 00013 public: 00014 /// A new sensor data frame, might be called several (hundred) times a second. 00015 virtual void sensorUpdate(Vector3 data) {} 00016 }; 00017 00018 virtual void setDelegate(Delegate &d) { 00019 delegate = &d; 00020 } 00021 00022 /// Power on a sensor and make it ready for use. 00023 /// @return true if power-up was successful, false otherwise. 00024 virtual bool powerOn() = 0; 00025 00026 /// Power off a sensor. This will generally only put the sensor into deep sleep. 00027 virtual void powerOff() = 0; 00028 00029 virtual void start() = 0; ///< Start continuous data capture. If a delegate is set, its sensorUpdate() method will be called for each data frame. 00030 virtual void stop() = 0; ///< Stop capturing data. 00031 00032 virtual Vector3 read() = 0; ///< Read and return instantaneous (current) sensor data. No need to start the sensor. 00033 00034 Sensor() : delegate(&defaultDelegate) {} 00035 00036 protected: 00037 Delegate defaultDelegate; 00038 Delegate *delegate; 00039 }; 00040 00041 #endif//_H_SENSOR_H
Generated on Wed Jul 13 2022 08:50:41 by
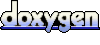